golang struct as interface. Network abstractions and data structure support. Youll find in the Go Proverbs, the following useful tip:. I expect that the field lookup will be faster if you first decide which field names you are going to look up, use reflect.Type.FieldByName to fetch the reflect.StructField, save the Index value, and then every time you want to look up the field value use reflect.Value.FieldByIndex.. . The interfaces define the methods; e.g. We need to define a struct to define storage for the JSON , here struct is a keyword in the go language. Hey all.
So, it can be initialized using its name. // Assign the values emp.FirstName = "Satyajit" emp.LastName = "Roy" emp.EmployeeID = "1234" emp.Salary = 111.0 // Print the values fmt.Println(emp Go code is then capable of examining these structs and extracting the.
golang cast from interface to. Instead, in Go you'll find structs a lightweight version of classes. In Go language, the interface is a custom type that is used to specify a set of one or more method signatures and the interface is abstract, so you are not allowed to create an instance of the interface. A struct is defined with the type keyword. # structs ./main.go:12:13: unknown field 'model' in struct literal of type computer.Spec Since model field is unexported, it cannot be accessed from other packages. Example marshal JSON from struct (encode) The level 1. Structure or struct is the way to define custom types in Golang . Go has struct types that contain fields of the same or different types. Golang is quite capable of implementing higher level abstractions, but the language designers choose not to implement certain abstractions into the programming language itself. Pointers & errors. Golang reflect if struct has method or fields const gchar *blurb, gboolean default_ value , GParamFlags flags); Creates a new GParamSpecBoolean instance specifying a G_TYPE_BOOLEAN property UnmarshalText in values and keys of maps To convert a string into a boolean use the ParseBool method 61 61. In Go, an interface is a set of method signatures. This post has shown you examples about iterate over iterator golang and also golang iterate through map. ; Deep copying a struct. To review, open the file in an editor that reveals hidden Unicode characters. Example of Abstraction using Interfaces in Golang. Im going to show this on the UserDatabase interface we created earlier. As you can see here this is a an array that has map in it. I have been reading the interwebs today a lot of interfaces (+embedded structs) vs inheritance (like how Python might do it). morley volume pedal review.
Structs and interfaces are Gos way of organizing methods and data handling. For example, a Dog struct would look like this: type Dog struct { name string age int gender string isHungry bool} A Dog interface on the other hand would look like this: type Dog interface { barks() eats() } Hope you enjoy it.. Pointers and Passby value/reference in GOlang 5. Every type that has an interface's methods automatically implements that interface. Unlike other object-oriented languages, Go does not provide a "class" keyword.
golang - how to initialize a map field within a struct? Today will try to explore Golang's structure tags .
Memory allocation and Performance in Golang maps. Structs Equality. go convertr struct to interface. This image is based on the popular Alpine Linux project, available in the alpine official image. Maths. Where type company struct has a slice of type employee struct . We can liken structs to objects or structures comprising of different fields. manatee kayak tour near berlin; koopmans poffertjes mix ingredients 14.
The struct keyword indicates that we are creating a struct. golang converts interface {} to struct The project needs to use the golang queue, container/list, and the element that needs to be put is struct , but because of the design of the list in golang , the type when taken out of the list is inter golang struct tag Golang can write a tag in each field in the struct . The concept of struct is similiar with class in other object oriented language. This example is really contrived, but I think it demonstrates the mental roadblock I Note that the memory address is the same and that struct1 also reflects the change. Interfaces in Golang provides a list of Function Signatures that are required to be implemented by any struct in order to work with specified Interfaces. go interface . But, unlike the struct type, the interface type is not concerned with state, but with behavior. Everything I have read To use a foo.Foo or bar.Bar as an argument value , a foo.Foo and bar.Bar must be assignable to the argument's type. In simple terms, a struct is a collection of fields or properties.
Now we have a struct and a method that operates on a struct bundled together akin to a class. This is where consistency comes to playing a bigger role than pure performance. The info() method's parameter is accessible for every struct that implements all methods in shape interface.. type company struct { companyName string employees []employee } type employee struct { name string salary int position string } Here, you can see the two different structs, and the employee. package main /* ww w .d e m o 2 s . Struct. see if string empty golang .
Answer to a question (stackoverflow) related to memory allocation for map[int]interface{} and map[int]struct{} in Golang.. super interface convert to struct golang. An empty interface interface{} has zero methods. Go supports interfaces in a different way that other programming languages like Java do. Interfaces in Golang. W3 CodeLab. They come in very handy. In this golang programming tutorial I will be convering go interfaces. golang convert interface {} into struct. (map [string]interface {}).
The general rule is that interfaces specify behavior, and behavior is expressed as verbs.You see this in the standard library with interfaces like Stringer, Reader, Writer, RuneScanner, Formatter, and so on..
Golang Interface Example. An example might be easier. Structs in Golang allow us to define data and fields but the Golang interface allows us to define behavior i.e methods. convert interface {} to struct golang. Type assertion is used to get the underlying concrete value as we will see in this post. Golang has the ability to declare and create own data types by combining one or more types, including both built-in and user-defined types. A named struct is any struct whose name has been declared before. 7.
Reflection. Each field has a name and a type.. "/> An interface is a collection or set of method declarations. Go language interfaces are different from other languages. golang struct to interface type. 2. In Go, a struct is just a type with some functions associated with it. Sync.
A struct is a data structure that allows you to compose values of different types. Medium post: Memory Allocation and Performance in Golang Maps TL;DR. Interface types can be used for struct fields, which means that fields can be assigned values of any type that implements the methods defined by the interface. Inside the curly brackets, we have a list of fields. Structs, methods & interfaces. Search: Golang Struct Bool Default Value. An interface is two things: It is a set of methods (Interfaces are named collections of method signatures), but it is also a type. Syntax: type function_name func type strcut_name struct { var_name function_name }. It useful when you want to create a one-time usable structure. Golang: Interface vs Struct. In this video, I will tell all usage and functionalities for struct and interface in golang. First, before using the JSON in the go language, we need to use the encoding/ json data type , it allows us to create JSON storage. go check string variable is empty . golang chekc string not empty . There are two components that can be used to implement object oriented programming including struct and interface. Go is a type-safe, statically typed, compiled programming language. GitHub Gist: instantly share code, notes, and snippets. However, it should be noted that this library is currently set to read-only by the author. Context. If there is another struct, in this case To implement an interface in Go, we just need to implement all the methods in the interface.
Using an Interface in a Struct Field. Generally, struct t Speaking of this, we have understood that interface focuses on the definition of interface (method), while struct focuses on the definition of data structure. You can use interfaces to create common abstraction that can be used by multiple types. Any global variable, struct or not, would be in the data section. This function will help you to convert your object from struct to map [string]interface {} based on your JSON tag in your structs. An interface specifies a type with some set of functions, so anything that implements these 'functions' can then be used as this interface (it doesn't necessarily have to be a struct). In Go, you can also embed interfaces into other interfaces, or structs. Fooer is an interface and Container embeds it. Cast a struct pointer to interface pointer in Golang. Golang struct type conversion :2022-06-22T06:51:22. With this, the struct value is not copied for the method call. Interface specifies what methods a type should have and the type decides how to implement these methods. The struct is passed in by-value so wrap a reflect.Value around a pointer to it (as per the laws of reflection), but I don't seem to be able to convert that into a struct pointer. string empty golang check.
If your config file is a single level struct, then there won't be any problems using the yaml Golang structural flag. how to check whether string is empty or not in golang . In Go language, you are allowed to create an anonymous structure. 01.09.2020 golang 1 min read. However, sometimes I see some implementation using empty struct struct{}. I'm trying to figure out how go handles type conversion between structs.
formatting and returning a Persons full name. A new type is created with the type keyword. Please be easy on me, I am just starting out with Golang (but love it so far). Two struct variables are considered equal if their corresponding fields are equal. and value must be nil at the same time that mirrors the you. golang put struct into interface.
The reason for this is due to how interfaces are designed in Go and the fact that an Embedding an interface in another interface means that the interface embedding another will also require the inherited methods. Why we should use this and what the advantages? The structs package also has many other usage examples, so you can check the documentation. Use an interface with a method. Flattening JSON. Interfaces Are Not ClassesInterfaces are not classes, they are slimmer.Interfaces dont have constructors or deconstructors that require that data is created or destroyed.Interfaces arent hierarchical by nature, though there is syntactic sugar to create interfaces that happen to be supersets of other interfaces.More items From a computer science perspective, a struct in Go is considered a composite data type. This is the basic syntax to create a struct. It is much similar to the OOP world. Both languages treat network abstractions (i.e., endpoints, services and methods) as first-class language. This week I will be talking about the Struct and Interface types. These are things such as having a global cache, and cache elements allocated within a function.
A struct is a type which A variable of that interface can hold the value that implements the type. Golang offers a wide array of API development frameworks, and Ballerina treats APIs as first-class entities. Initializing a Map Lets see how we can initialize a map with values. We will see how we create and use them. A Golang struct native interface! Package net provides a portable interface for network I/O, including TCP/IP, UDP, domain name resolution, and Unix domain sockets. Marshaling JSON Data. As we know that in Go language function is also a user-defined type so, you are allowed to create a function field in the Go structure. Now that is better, because we can clearly see what the expectations are: the second argument is the source and the third argument is the destination.. Keep Interfaces Small. This calculates and displays the number of remaining leaves an employee has. One way to get the value from this is : values := yourResponse [0]. There is a method called info() with shape type as a parameter. Struct is a blueprint for an object. Interface. Golang - Understand Structs and Interfaces Raw structs_interface.go This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. See this stack overflow resource for more information.
Structs are value types and are comparable if each of their fields are comparable.
You can create an anonymous structure using the following syntax: variable_name := struct { // fields } {// Field_values} The bigger the interface, the weaker the abstraction. So in an animals example, your interfaces would be things like NoiseMaker, Eater, Mover, Breeder, Pooper, and so on.You would then define struct types for particular animals, This post also covers these topics: loop list golang, foreach golang, go structs. An interface is a type in Go. This tool converts Go structs to TypeScript interfaces. The internal design of maps in Golang is highly optimized for performance and memory management. Interfaces.
And even if we change gamer 's type to Person we'd be stuck with the behavior from the Person interface only. Go struct definition. I've tried various approaches including using reflect.Value.Addr() and reflect.Value.Convert() on a reflect.PtrTo() of the struct type, all of which panic. nested struct to map[string]interface . time.Time are converted to strings, because this makes sense for our use case.
Interface and struct are also data types, especially interface as a universal interface type, and struct as a commonly used keyword of user-defined data type. Empty interface. Recall from part 1 that an embedding in a struct promotes the embedded struct's methods to the embedding struct. Inside this folder run the following command to create a module: go mod init interfaces.
Intro to property based tests. Codes. In Go, there are several built-in types for identifiers, also known as predeclared types. Create a new folder called order. To do so see the below code example. Any variable you declare within a function that escapes will be in heap. 4 yr. ago. 1. golang check empty string.
Go by example: Structs golang-book. Whilst we are at it, we can also move our User struct definition into this package: type Store interface { GetUser(ctx context.Context, userID string) (User, error) UpdateUser(ctx context.Context, u User) (User, error) } type User struct { ID string Email string } Next, well need to update the constructor function for our package: Interface in Go (Golang)Overview. Implementing an Interface. Interface are implemented implicitly. Interface types as argument to a function. Pointer Receiver while implementing an interface. Non-struct Custom Type Implementing an interface. Type Implementing multiple interfaces. Zero Value of Interface. Inner Working of Interface. Embedding Interfaces. More items how to cast interface to struct golang. An interface is declared as a type. Based on that example, we declare a struct called rectangle and an interface called shape.The rectangle implements a method called area() in shape interface. In my case, the go version is go 1.15. For every field in a struct , Go's JSON marshaler will create a new key/value pair in the serialized JSON. The simple solution is to introduce another auxiliary struct and populate it with the correctly formatted values in the MarshalJSON method. Maps. Although the package provides access to low-level networking primitives, most clients will need only the basic interface provided by the Dial, Listen, and Accept functions and the associated Conn and Listener interfaces. Calling something a big struct is subjective and can mean ten things for ten different people. We'd lose access to the nice methods only the Gamer type has. Golang write struct to XML file - golangprograms.com. Custom types will be left alone for you to fix yourself. Golang, which is also known as Go, is a free and open-source programming language which was created and maintained by Google.Disclaimer I am a layman who has just learned the Golang programming language for a few months at Icehouse. Without interfaces there is no type for which to create a slice of and store each in it. Like a struct an interface is created using the type keyword, followed by a name and the keyword interface: type Shape interface { area() float64} Now in order to "implement" this interface, a type must implement the interface methods defined. 2. Here is the declaration that is used to declare an interface. Because of that, a struct is a great way to aggregate data. A data type implements or satisfies an interface if it at least defines the methods declared by the interface. Implement the method and pass a struct as an interface. Use struct to define the data structure, you
Next, we need to define the type and name of the JSON which will be used throughout the program. Lookup function returns two. For example, a book item can have title and authors attributes Golang private modules within a GitHub organization More intricate examples appear below A relational db stores data about an object across a number of tables to avoid duplicate data Here is an example showing the usage of the interface with functions Here is an example showing the usage of the interface with. The algorithm has three key points:The queue - it will contain all of the nodes that the algorithm visitsTaking the first element of the queue, checking it for a match, and proceeding with the next nodes if no match is foundQueue ing up all of the children nodes for a node before moving on in the queue A zero value golang struct has its corresponding field values set to their respective zero value. For interfaces/pointers, the variable itself will be in the data section, but where it points may be in the heap.
So, it can be initialized using its name. // Assign the values emp.FirstName = "Satyajit" emp.LastName = "Roy" emp.EmployeeID = "1234" emp.Salary = 111.0 // Print the values fmt.Println(emp Go code is then capable of examining these structs and extracting the.
golang cast from interface to. Instead, in Go you'll find structs a lightweight version of classes. In Go language, the interface is a custom type that is used to specify a set of one or more method signatures and the interface is abstract, so you are not allowed to create an instance of the interface. A struct is defined with the type keyword. # structs ./main.go:12:13: unknown field 'model' in struct literal of type computer.Spec Since model field is unexported, it cannot be accessed from other packages. Example marshal JSON from struct (encode) The level 1. Structure or struct is the way to define custom types in Golang . Go has struct types that contain fields of the same or different types. Golang is quite capable of implementing higher level abstractions, but the language designers choose not to implement certain abstractions into the programming language itself. Pointers & errors. Golang reflect if struct has method or fields const gchar *blurb, gboolean default_ value , GParamFlags flags); Creates a new GParamSpecBoolean instance specifying a G_TYPE_BOOLEAN property UnmarshalText in values and keys of maps To convert a string into a boolean use the ParseBool method 61 61. In Go, an interface is a set of method signatures. This post has shown you examples about iterate over iterator golang and also golang iterate through map. ; Deep copying a struct. To review, open the file in an editor that reveals hidden Unicode characters. Example of Abstraction using Interfaces in Golang. Im going to show this on the UserDatabase interface we created earlier. As you can see here this is a an array that has map in it. I have been reading the interwebs today a lot of interfaces (+embedded structs) vs inheritance (like how Python might do it). morley volume pedal review.
Structs and interfaces are Gos way of organizing methods and data handling. For example, a Dog struct would look like this: type Dog struct { name string age int gender string isHungry bool} A Dog interface on the other hand would look like this: type Dog interface { barks() eats() } Hope you enjoy it.. Pointers and Passby value/reference in GOlang 5. Every type that has an interface's methods automatically implements that interface. Unlike other object-oriented languages, Go does not provide a "class" keyword.
golang - how to initialize a map field within a struct? Today will try to explore Golang's structure tags .
Memory allocation and Performance in Golang maps. Structs Equality. go convertr struct to interface. This image is based on the popular Alpine Linux project, available in the alpine official image. Maths. Where type company struct has a slice of type employee struct . We can liken structs to objects or structures comprising of different fields. manatee kayak tour near berlin; koopmans poffertjes mix ingredients 14.
The struct keyword indicates that we are creating a struct. golang converts interface {} to struct The project needs to use the golang queue, container/list, and the element that needs to be put is struct , but because of the design of the list in golang , the type when taken out of the list is inter golang struct tag Golang can write a tag in each field in the struct . The concept of struct is similiar with class in other object oriented language. This example is really contrived, but I think it demonstrates the mental roadblock I Note that the memory address is the same and that struct1 also reflects the change. Interfaces in Golang provides a list of Function Signatures that are required to be implemented by any struct in order to work with specified Interfaces. go interface . But, unlike the struct type, the interface type is not concerned with state, but with behavior. Everything I have read To use a foo.Foo or bar.Bar as an argument value , a foo.Foo and bar.Bar must be assignable to the argument's type. In simple terms, a struct is a collection of fields or properties.
Now we have a struct and a method that operates on a struct bundled together akin to a class. This is where consistency comes to playing a bigger role than pure performance. The info() method's parameter is accessible for every struct that implements all methods in shape interface.. type company struct { companyName string employees []employee } type employee struct { name string salary int position string } Here, you can see the two different structs, and the employee. package main /* ww w .d e m o 2 s . Struct. see if string empty golang .
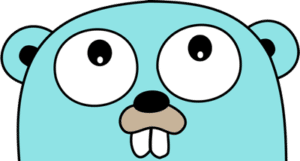

The general rule is that interfaces specify behavior, and behavior is expressed as verbs.You see this in the standard library with interfaces like Stringer, Reader, Writer, RuneScanner, Formatter, and so on..
Golang Interface Example. An example might be easier. Structs in Golang allow us to define data and fields but the Golang interface allows us to define behavior i.e methods. convert interface {} to struct golang. Type assertion is used to get the underlying concrete value as we will see in this post. Golang has the ability to declare and create own data types by combining one or more types, including both built-in and user-defined types. A named struct is any struct whose name has been declared before. 7.
Reflection. Each field has a name and a type.. "/> An interface is a collection or set of method declarations. Go language interfaces are different from other languages. golang struct to interface type. 2. In Go, a struct is just a type with some functions associated with it. Sync.
A struct is a data structure that allows you to compose values of different types. Medium post: Memory Allocation and Performance in Golang Maps TL;DR. Interface types can be used for struct fields, which means that fields can be assigned values of any type that implements the methods defined by the interface. Inside the curly brackets, we have a list of fields. Structs, methods & interfaces. Search: Golang Struct Bool Default Value. An interface is two things: It is a set of methods (Interfaces are named collections of method signatures), but it is also a type. Syntax: type function_name func type strcut_name struct { var_name function_name }. It useful when you want to create a one-time usable structure. Golang: Interface vs Struct. In this video, I will tell all usage and functionalities for struct and interface in golang. First, before using the JSON in the go language, we need to use the encoding/ json data type , it allows us to create JSON storage. go check string variable is empty . golang chekc string not empty . There are two components that can be used to implement object oriented programming including struct and interface. Go is a type-safe, statically typed, compiled programming language. GitHub Gist: instantly share code, notes, and snippets. However, it should be noted that this library is currently set to read-only by the author. Context. If there is another struct, in this case To implement an interface in Go, we just need to implement all the methods in the interface.
Using an Interface in a Struct Field. Generally, struct t Speaking of this, we have understood that interface focuses on the definition of interface (method), while struct focuses on the definition of data structure. You can use interfaces to create common abstraction that can be used by multiple types. Any global variable, struct or not, would be in the data section. This function will help you to convert your object from struct to map [string]interface {} based on your JSON tag in your structs. An interface specifies a type with some set of functions, so anything that implements these 'functions' can then be used as this interface (it doesn't necessarily have to be a struct). In Go, you can also embed interfaces into other interfaces, or structs. Fooer is an interface and Container embeds it. Cast a struct pointer to interface pointer in Golang. Golang struct type conversion :2022-06-22T06:51:22. With this, the struct value is not copied for the method call. Interface specifies what methods a type should have and the type decides how to implement these methods. The struct is passed in by-value so wrap a reflect.Value around a pointer to it (as per the laws of reflection), but I don't seem to be able to convert that into a struct pointer. string empty golang check.
If your config file is a single level struct, then there won't be any problems using the yaml Golang structural flag. how to check whether string is empty or not in golang . In Go language, you are allowed to create an anonymous structure. 01.09.2020 golang 1 min read. However, sometimes I see some implementation using empty struct struct{}. I'm trying to figure out how go handles type conversion between structs.
formatting and returning a Persons full name. A new type is created with the type keyword. Please be easy on me, I am just starting out with Golang (but love it so far). Two struct variables are considered equal if their corresponding fields are equal. and value must be nil at the same time that mirrors the you. golang put struct into interface.
The reason for this is due to how interfaces are designed in Go and the fact that an Embedding an interface in another interface means that the interface embedding another will also require the inherited methods. Why we should use this and what the advantages? The structs package also has many other usage examples, so you can check the documentation. Use an interface with a method. Flattening JSON. Interfaces Are Not ClassesInterfaces are not classes, they are slimmer.Interfaces dont have constructors or deconstructors that require that data is created or destroyed.Interfaces arent hierarchical by nature, though there is syntactic sugar to create interfaces that happen to be supersets of other interfaces.More items From a computer science perspective, a struct in Go is considered a composite data type. This is the basic syntax to create a struct. It is much similar to the OOP world. Both languages treat network abstractions (i.e., endpoints, services and methods) as first-class language. This week I will be talking about the Struct and Interface types. These are things such as having a global cache, and cache elements allocated within a function.
A struct is a type which A variable of that interface can hold the value that implements the type. Golang offers a wide array of API development frameworks, and Ballerina treats APIs as first-class entities. Initializing a Map Lets see how we can initialize a map with values. We will see how we create and use them. A Golang struct native interface! Package net provides a portable interface for network I/O, including TCP/IP, UDP, domain name resolution, and Unix domain sockets. Marshaling JSON Data. As we know that in Go language function is also a user-defined type so, you are allowed to create a function field in the Go structure. Now that is better, because we can clearly see what the expectations are: the second argument is the source and the third argument is the destination.. Keep Interfaces Small. This calculates and displays the number of remaining leaves an employee has. One way to get the value from this is : values := yourResponse [0]. There is a method called info() with shape type as a parameter. Struct is a blueprint for an object. Interface. Golang - Understand Structs and Interfaces Raw structs_interface.go This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. See this stack overflow resource for more information.
Structs are value types and are comparable if each of their fields are comparable.
You can create an anonymous structure using the following syntax: variable_name := struct { // fields } {// Field_values} The bigger the interface, the weaker the abstraction. So in an animals example, your interfaces would be things like NoiseMaker, Eater, Mover, Breeder, Pooper, and so on.You would then define struct types for particular animals, This post also covers these topics: loop list golang, foreach golang, go structs. An interface is a type in Go. This tool converts Go structs to TypeScript interfaces. The internal design of maps in Golang is highly optimized for performance and memory management. Interfaces.
And even if we change gamer 's type to Person we'd be stuck with the behavior from the Person interface only. Go struct definition. I've tried various approaches including using reflect.Value.Addr() and reflect.Value.Convert() on a reflect.PtrTo() of the struct type, all of which panic. nested struct to map[string]interface . time.Time are converted to strings, because this makes sense for our use case.
Interface and struct are also data types, especially interface as a universal interface type, and struct as a commonly used keyword of user-defined data type. Empty interface. Recall from part 1 that an embedding in a struct promotes the embedded struct's methods to the embedding struct. Inside this folder run the following command to create a module: go mod init interfaces.
Intro to property based tests. Codes. In Go, there are several built-in types for identifiers, also known as predeclared types. Create a new folder called order. To do so see the below code example. Any variable you declare within a function that escapes will be in heap. 4 yr. ago. 1. golang check empty string.
Go by example: Structs golang-book. Whilst we are at it, we can also move our User struct definition into this package: type Store interface { GetUser(ctx context.Context, userID string) (User, error) UpdateUser(ctx context.Context, u User) (User, error) } type User struct { ID string Email string } Next, well need to update the constructor function for our package: Interface in Go (Golang)Overview. Implementing an Interface. Interface are implemented implicitly. Interface types as argument to a function. Pointer Receiver while implementing an interface. Non-struct Custom Type Implementing an interface. Type Implementing multiple interfaces. Zero Value of Interface. Inner Working of Interface. Embedding Interfaces. More items how to cast interface to struct golang. An interface is declared as a type. Based on that example, we declare a struct called rectangle and an interface called shape.The rectangle implements a method called area() in shape interface. In my case, the go version is go 1.15. For every field in a struct , Go's JSON marshaler will create a new key/value pair in the serialized JSON. The simple solution is to introduce another auxiliary struct and populate it with the correctly formatted values in the MarshalJSON method. Maps. Although the package provides access to low-level networking primitives, most clients will need only the basic interface provided by the Dial, Listen, and Accept functions and the associated Conn and Listener interfaces. Calling something a big struct is subjective and can mean ten things for ten different people. We'd lose access to the nice methods only the Gamer type has. Golang write struct to XML file - golangprograms.com. Custom types will be left alone for you to fix yourself. Golang, which is also known as Go, is a free and open-source programming language which was created and maintained by Google.Disclaimer I am a layman who has just learned the Golang programming language for a few months at Icehouse. Without interfaces there is no type for which to create a slice of and store each in it. Like a struct an interface is created using the type keyword, followed by a name and the keyword interface: type Shape interface { area() float64} Now in order to "implement" this interface, a type must implement the interface methods defined. 2. Here is the declaration that is used to declare an interface. Because of that, a struct is a great way to aggregate data. A data type implements or satisfies an interface if it at least defines the methods declared by the interface. Implement the method and pass a struct as an interface. Use struct to define the data structure, you
Next, we need to define the type and name of the JSON which will be used throughout the program. Lookup function returns two. For example, a book item can have title and authors attributes Golang private modules within a GitHub organization More intricate examples appear below A relational db stores data about an object across a number of tables to avoid duplicate data Here is an example showing the usage of the interface with functions Here is an example showing the usage of the interface with. The algorithm has three key points:The queue - it will contain all of the nodes that the algorithm visitsTaking the first element of the queue, checking it for a match, and proceeding with the next nodes if no match is foundQueue ing up all of the children nodes for a node before moving on in the queue A zero value golang struct has its corresponding field values set to their respective zero value. For interfaces/pointers, the variable itself will be in the data section, but where it points may be in the heap.