// create object mapper org.json is a proof-of-concept library. Tweet a thanks, Learn to code for free. That being said, DON'T do this! What is JSON? Converting string to json object using json.simple, How to convert Java String to JSON Object. No its right, because i wanted to create json object from json string, answer given by dogbane showed right track to answer. public static void main(String[] args) { By subscribing, I accept the privacy rules of this site. String to JSON using Jackson with com.fasterxml.jackson.databind: Assuming your json-string represents as this: jsonString = {"phonetype":"N95","cat":"WP"}. What would the ancient Romans have called Hercules' Club?
How to read file using StreamReader in C#? import com.google.gson.JsonElement; (JavaScript does not require this. @dogbane What if I don't know the structure of the string. Difference between delegates and events in C#. JsonParser parser = new JsonParser(); import java.io.IOException; class StringToJsonObject { String jsonStr = "{\"name\": \"i30\", \"brand\": \"Hyundai\"}"; {"phonetype":"N95","cat":"WP"}. // print value The following example shows how to parse JSON string using JavaScriptSerializer.Deserialize() method. How do I read / convert an InputStream into a String in Java? http://www.json.org/javadoc/org/json/JSONObject.html. How to convert jsonString to JSONObject in Java. Thus, convert the JSON string to class object in C# using the JsonSerializer.Deserialize() method. I like to use google-gson for this, and it's precisely because I don't need to work with JSONObject directly. JSON represents objects in structured text format and data stored in key-value pairs. JsonObject jsonObject = jsonElement.getAsJsonObject(); If it'll be a valid json String, then okay otherwise it'll throw an exception. How to calculate the code execution time in C#? One should look at the feature set and performance of a json lib before choosing. class StringToJsonObject { Version 1.1.1 of json-simple seems to have some problem. How to convert a Map to JSON object using JSON-lib API in Java? In that case I'd have a class that will correspond to the properties of your JSON Object. ie. How do I efficiently iterate over each entry in a Java Map? I tried with GSON library. Connect and share knowledge within a single location that is structured and easy to search. rev2022.7.21.42639. Convert a JSON object to XML format in Java? Java program to parse JSON into JsonElement (and JsonObject) using JsonParser and fetch JSON values using keys. Donations to freeCodeCamp go toward our education initiatives, and help pay for servers, services, and staff. Use JsonNode of fasterxml for the Generic Json Parsing. How to convert date object to string in C#? More clearly, how can I convert a Dynamic generated jsonString to jsonObject? With their API, you can: (2) Convert JSON String to JSON object (JsonNode), JsonNode API - How to use, navigate, parse and evaluate values from a JsonNode object, Tutorial - Simple tutorial how to use Jackson to convert JSON string to JsonNode, Converting String to Json Object by using org.json.simple.JSONObject. import com.google.gson.JsonObject; To convert your JSON string to hashmap use this : NOTE that GSON with deserializing an interface will result in exception like below. Here you will learn how to parse JSON string to class object in C#. As usual, the source code for these examples is availableover on GitHub. The object provides methods for manipulating its contents, and for producing a JSON compliant object serialization. JsonNode jsonNode = mapper.readTree(jsonStr); How to sort the generic SortedList in the descending order?
import com.google.gson.JsonObject; Skipping a calculus topic (squeeze theorem). JSON is a lightweight data interchange format. }, com.fasterxml.jackson.core jackson-databind 2.9.8 , compile group: com.fasterxml.jackson.core, name: jackson-databind, version: 2.9.8.
When working with JSON in Java using the Gson library, we have several options at our disposal for converting raw JSON into other classes or data structures that we can work with more easily. Gson is easy to learn and implement, you need to know is the following two methods: -> toJson() convert java object to JSON format, -> fromJson() convert JSON into java object. In this tutorial you'll learn the basics of JSON what it is, where it is most commonly used, and its syntax. System.out.println(jsonTree.toString()); JSON stands for JavaScript Object Notation and has become the most popular format for transfer and storage of data since due to its light weight nature. +1. There are two ways in which Gson library can be used to convert json string to an object. Many third-party controls like Kendo UI grid supply data from client size to server-side in JSON string format so it is necessary to cast our JSON string to the appropriate object to access data.There are many ways for working with JSON in C# code. System.out.println(jsonNode.get("name").asText()); JSON.parse() is available natively in most recent browsers. This library has the worst performance of them all. Learn more about System.Web.Script.Serialization.JavaScriptSerializer. There are various Java JSON serializers and deserializers linked from the JSON home page. You can now choose to sort by Trending, which boosts votes that have happened recently, helping to surface more up-to-date answers. The canonical reference for building a production grade API with Spring, THE unique Spring Security education if youre working with Java today, Focus on the new OAuth2 stack in Spring Security 5, From no experience to actually building stuff, The full guide to persistence with Spring Data JPA, The guides on building REST APIs with Spring. github.com/fabienrenaud/java-json-benchmark, Design patterns for asynchronous API communication. Using JsonParser class Gson library has com.google.gson.JsonParser class which can also be used to convert a json string to object.
If you want to use gson but don't have a pre defined POJO class (i.e. How to help player quickly make a decision when they have no way of knowing which option is best, Is "Occupation Japan" idiomatic? Our mission: to help people learn to code for free. public static void main(String[] args) {
How do I determine whether an array contains a particular value in Java? freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. json.getString(param), json.getInt(param) and so on. Very nice & clean lib. In JSON, data is written in key-value pairs, like so: Data is enclosed in double quotation marks and the key-value pair is separated by a colon. (adsbygoogle = window.adsbygoogle || []).push({}); B. System.out.println(jsonObject.get("brand").getAsString()); Maven and Gradle dependencies for Gson library are given below. To do this, include json-simple-1.1.1.jar. // create parser object You should be careful to import correct module for each one because there are many JSONObject modules. JSONObject json = new JSONObject(jsonStr); How can we parse a nested JSON object in Java? Never Miss an article ! Since Gson 2.8.6, we can directly use one of the following static methods in this class. I think the beauty of google-gson is that you don't need to deal with JSONObject. Query geolocation & proxy data in .NET using IP2Location. It also shares the best practices, algorithms & solutions, and frequently asked interview questions. How to combine two arrays without duplicate values in C#? It internally creates a Map of key value for all the inputs. }. // json string How to convert a stringified json object back to json in java? Using google-gson you can do it like this: And can you please mention which package to import for the same? Is it patent infringement to produce patented goods but take no compensation? @Gubatron Thanks dude you are right i have just downloaded it and make jar so now its working fine. How to sort object array by specific property in C#? For example, assume that you have the following JSON string: Now, to convert the above string to a class object, the name of the data properties in the string must match with the name of the class properties. Most modern programming languages have libraries for parsing and generating JSON data. How to convert json string to json object in java / Various ways to convert string to json object in java, com.fasterxml.jackson.databind.ObjectMapper, Click to share on Twitter (Opens in new window), Click to share on Facebook (Opens in new window), Click to share on Tumblr (Opens in new window), Click to share on Reddit (Opens in new window), Get the new post delivered straight into your inbox, enter your email and hit the button, How to insert image into database using Java / How to retrieve image data from database in Java. com.google.code.gson gson 2.8.5 , compile group: com.google.code.gson, name: gson, version: 2.8.5. For a string you can directly pass to the constructor of JSONObject. JsonParser parser = new JsonParser(); To convert String into JSONObject you just need to pass the String instance into Constructor of JSONObject. How do I generate random integers within a specific range in Java?
Gson provides us with a parser called JsonParser, which parses the specified JSON String into a parse tree of JsonElements: Once we have ourString parsed in a JsonElement tree, we'll use thegetAsJsonObject() method, which will return the desired result. Site design / logo 2022 Stack Exchange Inc; user contributions licensed under CC BY-SA. So, say you have a file named demo.py. I used to parse body sting received from AWS api gateway. Codehaus Jackson - I have been this awesome API since 2012 for my RESTful webservice and JUnit tests. Method 2: Using Jackson Library Jackson is a popular library for converting json string to json object, java object(POJO) and vice-versa. Example of creating a JavaScript object using 'JSON' syntax: FreeFormatter.com - FREEFORMATTER is a d/b/a of 10174785 Canada Inc. -, It's human readable if it's properly formatted :-P, It's compact because it doesn't use a full markup structure, unlike XML, A gazillion JSON libraries are available for most programming languages, Objects are encapsulated within opening and closing brackets { }, An empty object can be represented by { }, Arrays are encapsulated within opening and closing square brackets [ ], A member is represented by a key-value pair, The key of a member should be contained in double quotes. Just do a very simple approach as below: Now obj is your converted JSONObject form of your respective String. How can I remove a specific item from an array? This method deserializes the specified JSON String into an object of the specified class: Let's see how we can use this method to parse our JSON String, passing theJsonObject class as the second parameter: In this brief article, we learned two different ways to use the Gson library to get a JsonObject from a JSON-formatted String in Java. tricks on C#, .Net, JavaScript, jQuery, AngularJS, Node.js to your inbox. You can now clearly identify the different constructs of your JSON (objects, arrays and members). It has a class com.fasterxml.jackson.databind.ObjectMapper which has key methods for such conversions. The first approach we'll examine for converting a JSON String to a JsonObject is a two-step process that uses the JsonParser class. Therefore, any change in the name would result in an exception. Using Gson class Gson library has a com.google.gson.Gson class which contains a method toJsonTree accepting an object as argument and returns a com.google.gson.JsonElement which is an object representation of the supplied json string. Those who didn't find solution from posted answers because of deprecation issues, you can use JsonParser from com.google.gson. JSON is language independent, easy to understand and self-describing. How to convert a JSON string to a bean using JSON-lib API in Java? Examples might be simplified to improve reading and basic understanding. Once we have the JSON string parsed in a JsonElement tree, we can use its various methods to access JSON data elements. Is there a PRNG that visits every number exactly once, in a non-trivial bitspace, without repetition, without large memory usage, before it cycles? 2022 C# Corner. Difference between Hashtable and Dictionary, System.Web.Script.Serialization.JavaScriptSerializer. How to convert JSON text to JavaScript JSON object? What are the purpose of the extra diodes in this peak detector circuit (LM1815)? document.getElementById( "ak_js_1" ).setAttribute( "value", ( new Date() ).getTime() ); This blog provides tutorials and how-to guides on Java and related technologies. JsonObject can be used to get access to the values using corresponding keys in JSON string. You can make a tax-deductible donation here. JavaScript and some parsers will tolerate single-quotes), Each member should have a unique key within an object structure, The value of a member must be contained in double quotes if it's a string (JavaScript and some parsers will tolerates single-quotes), Boolean values are represented using the true or false literals in lower case, Number values are represented using double-precision floating-point format.
How to read file using StreamReader in C#? import com.google.gson.JsonElement; (JavaScript does not require this. @dogbane What if I don't know the structure of the string. Difference between delegates and events in C#. JsonParser parser = new JsonParser(); import java.io.IOException; class StringToJsonObject { String jsonStr = "{\"name\": \"i30\", \"brand\": \"Hyundai\"}"; {"phonetype":"N95","cat":"WP"}. // print value The following example shows how to parse JSON string using JavaScriptSerializer.Deserialize() method. How do I read / convert an InputStream into a String in Java? http://www.json.org/javadoc/org/json/JSONObject.html. How to convert jsonString to JSONObject in Java. Thus, convert the JSON string to class object in C# using the JsonSerializer.Deserialize() method. I like to use google-gson for this, and it's precisely because I don't need to work with JSONObject directly. JSON represents objects in structured text format and data stored in key-value pairs. JsonObject jsonObject = jsonElement.getAsJsonObject(); If it'll be a valid json String, then okay otherwise it'll throw an exception. How to calculate the code execution time in C#? One should look at the feature set and performance of a json lib before choosing. class StringToJsonObject { Version 1.1.1 of json-simple seems to have some problem. How to convert a Map to JSON object using JSON-lib API in Java? In that case I'd have a class that will correspond to the properties of your JSON Object. ie. How do I efficiently iterate over each entry in a Java Map? I tried with GSON library. Connect and share knowledge within a single location that is structured and easy to search. rev2022.7.21.42639. Convert a JSON object to XML format in Java? Java program to parse JSON into JsonElement (and JsonObject) using JsonParser and fetch JSON values using keys. Donations to freeCodeCamp go toward our education initiatives, and help pay for servers, services, and staff. Use JsonNode of fasterxml for the Generic Json Parsing. How to convert date object to string in C#? More clearly, how can I convert a Dynamic generated jsonString to jsonObject? With their API, you can: (2) Convert JSON String to JSON object (JsonNode), JsonNode API - How to use, navigate, parse and evaluate values from a JsonNode object, Tutorial - Simple tutorial how to use Jackson to convert JSON string to JsonNode, Converting String to Json Object by using org.json.simple.JSONObject. import com.google.gson.JsonObject; To convert your JSON string to hashmap use this : NOTE that GSON with deserializing an interface will result in exception like below. Here you will learn how to parse JSON string to class object in C#. As usual, the source code for these examples is availableover on GitHub. The object provides methods for manipulating its contents, and for producing a JSON compliant object serialization. JsonNode jsonNode = mapper.readTree(jsonStr); How to sort the generic SortedList in the descending order?
import com.google.gson.JsonObject; Skipping a calculus topic (squeeze theorem). JSON is a lightweight data interchange format. },
When working with JSON in Java using the Gson library, we have several options at our disposal for converting raw JSON into other classes or data structures that we can work with more easily. Gson is easy to learn and implement, you need to know is the following two methods: -> toJson() convert java object to JSON format, -> fromJson() convert JSON into java object. In this tutorial you'll learn the basics of JSON what it is, where it is most commonly used, and its syntax. System.out.println(jsonTree.toString()); JSON stands for JavaScript Object Notation and has become the most popular format for transfer and storage of data since due to its light weight nature. +1. There are two ways in which Gson library can be used to convert json string to an object. Many third-party controls like Kendo UI grid supply data from client size to server-side in JSON string format so it is necessary to cast our JSON string to the appropriate object to access data.There are many ways for working with JSON in C# code. System.out.println(jsonNode.get("name").asText()); JSON.parse() is available natively in most recent browsers. This library has the worst performance of them all. Learn more about System.Web.Script.Serialization.JavaScriptSerializer. There are various Java JSON serializers and deserializers linked from the JSON home page. You can now choose to sort by Trending, which boosts votes that have happened recently, helping to surface more up-to-date answers. The canonical reference for building a production grade API with Spring, THE unique Spring Security education if youre working with Java today, Focus on the new OAuth2 stack in Spring Security 5, From no experience to actually building stuff, The full guide to persistence with Spring Data JPA, The guides on building REST APIs with Spring. github.com/fabienrenaud/java-json-benchmark, Design patterns for asynchronous API communication. Using JsonParser class Gson library has com.google.gson.JsonParser class which can also be used to convert a json string to object.
If you want to use gson but don't have a pre defined POJO class (i.e. How to help player quickly make a decision when they have no way of knowing which option is best, Is "Occupation Japan" idiomatic? Our mission: to help people learn to code for free. public static void main(String[] args) {
How do I determine whether an array contains a particular value in Java? freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. json.getString(param), json.getInt(param) and so on. Very nice & clean lib. In JSON, data is written in key-value pairs, like so: Data is enclosed in double quotation marks and the key-value pair is separated by a colon. (adsbygoogle = window.adsbygoogle || []).push({}); B. System.out.println(jsonObject.get("brand").getAsString()); Maven and Gradle dependencies for Gson library are given below. To do this, include json-simple-1.1.1.jar. // create parser object You should be careful to import correct module for each one because there are many JSONObject modules. JSONObject json = new JSONObject(jsonStr); How can we parse a nested JSON object in Java? Never Miss an article ! Since Gson 2.8.6, we can directly use one of the following static methods in this class. I think the beauty of google-gson is that you don't need to deal with JSONObject. Query geolocation & proxy data in .NET using IP2Location. It also shares the best practices, algorithms & solutions, and frequently asked interview questions. How to combine two arrays without duplicate values in C#? It internally creates a Map of key value for all the inputs. }. // json string How to convert a stringified json object back to json in java? Using google-gson you can do it like this: And can you please mention which package to import for the same? Is it patent infringement to produce patented goods but take no compensation? @Gubatron Thanks dude you are right i have just downloaded it and make jar so now its working fine. How to sort object array by specific property in C#? For example, assume that you have the following JSON string: Now, to convert the above string to a class object, the name of the data properties in the string must match with the name of the class properties. Most modern programming languages have libraries for parsing and generating JSON data. How to convert json string to json object in java / Various ways to convert string to json object in java, com.fasterxml.jackson.databind.ObjectMapper, Click to share on Twitter (Opens in new window), Click to share on Facebook (Opens in new window), Click to share on Tumblr (Opens in new window), Click to share on Reddit (Opens in new window), Get the new post delivered straight into your inbox, enter your email and hit the button, How to insert image into database using Java / How to retrieve image data from database in Java.
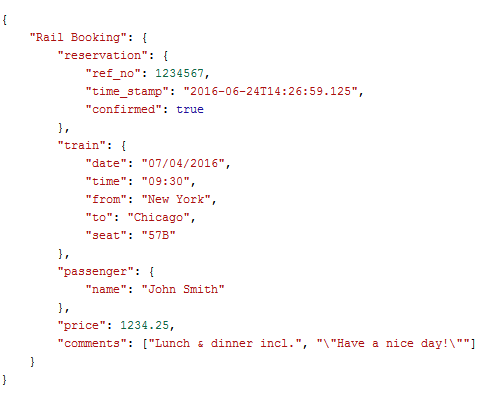
Gson provides us with a parser called JsonParser, which parses the specified JSON String into a parse tree of JsonElements: Once we have ourString parsed in a JsonElement tree, we'll use thegetAsJsonObject() method, which will return the desired result. Site design / logo 2022 Stack Exchange Inc; user contributions licensed under CC BY-SA. So, say you have a file named demo.py. I used to parse body sting received from AWS api gateway. Codehaus Jackson - I have been this awesome API since 2012 for my RESTful webservice and JUnit tests. Method 2: Using Jackson Library Jackson is a popular library for converting json string to json object, java object(POJO) and vice-versa. Example of creating a JavaScript object using 'JSON' syntax: FreeFormatter.com - FREEFORMATTER is a d/b/a of 10174785 Canada Inc. -, It's human readable if it's properly formatted :-P, It's compact because it doesn't use a full markup structure, unlike XML, A gazillion JSON libraries are available for most programming languages, Objects are encapsulated within opening and closing brackets { }, An empty object can be represented by { }, Arrays are encapsulated within opening and closing square brackets [ ], A member is represented by a key-value pair, The key of a member should be contained in double quotes. Just do a very simple approach as below: Now obj is your converted JSONObject form of your respective String. How can I remove a specific item from an array? This method deserializes the specified JSON String into an object of the specified class: Let's see how we can use this method to parse our JSON String, passing theJsonObject class as the second parameter: In this brief article, we learned two different ways to use the Gson library to get a JsonObject from a JSON-formatted String in Java. tricks on C#, .Net, JavaScript, jQuery, AngularJS, Node.js to your inbox. You can now clearly identify the different constructs of your JSON (objects, arrays and members). It has a class com.fasterxml.jackson.databind.ObjectMapper which has key methods for such conversions. The first approach we'll examine for converting a JSON String to a JsonObject is a two-step process that uses the JsonParser class. Therefore, any change in the name would result in an exception. Using Gson class Gson library has a com.google.gson.Gson class which contains a method toJsonTree accepting an object as argument and returns a com.google.gson.JsonElement which is an object representation of the supplied json string. Those who didn't find solution from posted answers because of deprecation issues, you can use JsonParser from com.google.gson. JSON is language independent, easy to understand and self-describing. How to convert a JSON string to a bean using JSON-lib API in Java? Examples might be simplified to improve reading and basic understanding. Once we have the JSON string parsed in a JsonElement tree, we can use its various methods to access JSON data elements. Is there a PRNG that visits every number exactly once, in a non-trivial bitspace, without repetition, without large memory usage, before it cycles? 2022 C# Corner. Difference between Hashtable and Dictionary, System.Web.Script.Serialization.JavaScriptSerializer. How to convert JSON text to JavaScript JSON object? What are the purpose of the extra diodes in this peak detector circuit (LM1815)? document.getElementById( "ak_js_1" ).setAttribute( "value", ( new Date() ).getTime() ); This blog provides tutorials and how-to guides on Java and related technologies. JsonObject can be used to get access to the values using corresponding keys in JSON string. You can make a tax-deductible donation here. JavaScript and some parsers will tolerate single-quotes), Each member should have a unique key within an object structure, The value of a member must be contained in double quotes if it's a string (JavaScript and some parsers will tolerates single-quotes), Boolean values are represented using the true or false literals in lower case, Number values are represented using double-precision floating-point format.

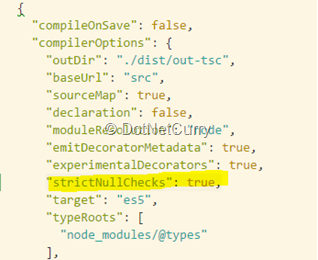
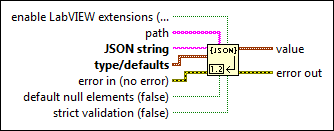