I understand that Entity data models should be separated from real domain models, to avoid coupling between infrastructural concerns and domain itself, but i was wondering if all domain properties do not have public setters, how can we map from data model to domain model, especially if repository implementation resides in infrastructural part of See for example Having the domain model separated from the persistence model (first Google hit for "why separate domain model from dao") for an explanation of why you'd want that. Adding a view First of all, we need to add a view to the database. On first glance, it seems like hell of a overkill to have 3 models, but it allows us to design the domain without having ever to bother with technical concerns, for example having to set read only fields as private setters, because the ORM can't set read only fields etc. Entity Framework Fluent API is used to configure domain classes to override conventions. Choose the Generate from database option in the Entity Data Model wizard. The wizard lets you connect to the database and select the Department and Employee tables to be included in the model. Once the wizard has completed the model is added to your project and you are able to view it in the EF Designer. C (r)UD: This is Create, (Read), Update, and Delete I use the term C (r)UD to separate the Read from the functions that change the database (Create, Update are Delete). A theoretical example of Configure the migrations assembly: C#. Aggregates help to simplify the domain model by gathering multiple domain objects under a single abstraction. Then convert it to the correct business entity and finally return it. A public key credential can only be used for authentication with the same entity (as identified by RP ID) it was registered with. Comment because I haven't used EF in a long time, and I feel like this is a shot in the dark. Change entity framework database first auto generated domain classes are you planning then to go code first? Entity Framework Database Fisrt Approach Separate domain classes from edmx Author : HASSAN MD TAREQ Separating T4 Generated Domain Classes In Visual Studio Create a new project In File Explorer Move [model].tt to the new project folder Back in Visual Studio, Include [model].tt in Domain Classes project Delete [model].tt from data project This is where OOD fits into your code and where you code business logic. in the application layer), but there is no strict rule to follow. Database-first: You start with a database, and Entity Framework generates the code. The idea was to add the satellite assemblies as references then create migration for all models . I now want to separate the models into a separate class Library. You can now create multiple domains with separate domain models within the same assembly. See also.
How to separate domain classes from EF code first layer. An entity in Entity Framework is a class that maps to a database table.
Table-per-concrete-type (TPC) is supported by EF6, but.
and SSDL)? People often confuse entity with model. It persists or merges the given entity by using the underlying JPA EntityManager. In convention names, an additional 's' is added if there is more than one record in the object. The following code depicts the model representation of the database example above: public class Author. Entity Framework is an open-source object-relational mapper framework for .NET applications supported by Microsoft.
Entity Framework Core 5 can handle views and in this article, I'm going to show you how.
There are many, many things you can do to help, so please feel free to jump into the Forum and ask what you can do to help! Entity Framework (EF) is Microsofts recommended data access technology when building new .NET applications. For a non-normative list of XSLT elements, see D Element Syntax Summary. In Entity Framework Core, the ModelBuilder class acts as a Fluent API. Credentials are a part of our daily lives; driver's licenses are used to assert that we are capable of operating a motor vehicle, university degrees can be used to assert our level of education, and government-issued passports enable us to travel between countries. Consider having separate models for your domain entities and your persistence entities. Model first is the domain modelling approach in Entity Framework. This means that you can use "plain-old" CLR objects (POCO), such as existing domain objects, with your data model. These are some of the primary trait of entities. Overview The Entity Framework provides three separate approaches to work with data models and each one has its own pros and cons. Fluent API uses the Modelbuilder instance to configure the domain model. This approach is adopted by the architect and solution lead developers. Partitioned (discriminator) data; 19.4. When I make the connection string match the Entity Framework model it works fine. However, these two are quite different. If you use a repository, have the e.g. When working with Code First, you define your model by defining your domain CLR classes. And create separate project for Identity, with IdentityDbContext where MemberIdentiy : IdentityUser, IMember. 1. var actor = context. Maybe try in the constructor this.Active = true;?I think the DB value will take precedence when fetching, but careful if new'ing then attaching an entity for an update without a fetch first, as the change tracking might see this as you wanting to update the value. This metadata is cached globally and is available to other instances of ObjectContext in the same application domain. The One Popular Answer Basically it depends on your use. Then convert it to the correct business entity and finally return it. The ability to separate the domain classes into a separate domain project is useful when developing applications with EF6. You can create multiple Edmx models in one .NET project.
The problem lies in the fact that Entity Framework and WCF Data Services use a common format to describe the data model: the Entity Data Model (EDM).
What is a good way to go from a normal connection string to an EF connection string in code? Popular Answer I understand that Entity data models should be separated from real domain models Not true avoid coupling between infrastructural concerns and domain itself True You CAN use EF to directly persist your domain models, however you should not couple your domain models to EF. Up to now, I had one large Edmx file with all the required db objects for all my repositories and also only in one color. Your domain should be unaware of persistence e.g.
An Entity as defined by EF concretely means any object that you wish to map some subpart of your relational model to (and from). What does this look like? A fetch method typically uses ADO.NET or an ORM like Entity Framework to load the database object/entity. Separate database; 19.2.2. They are dumb objects relying on calling code for validation and other business logic. your methods: deactivate () and remove (). The quirk with this setup is that some of the satellite assemblies have same classes but with more or less properties (depending on whether they make sense in their respective context ). It is the set of entities mapped to your database using an ORM framework. The EntityFrameworkRepository class works with two generic types: ToDataEntity (TDomainModel): To convert to data entities (for Add () and Update () methods) ToDomainModel (TEntityModel): To construct domain models (for the Find () method). LibriVox volunteers narrate, proof listen, and upload chapters of books and other textual works in the public domain. Opening the database connection: Moderate 1: As needed. Entity Framework (EF) is Microsoft's recommended data access technology when building new .NET applications. Model and mapping metadata used by the Entity Framework is loaded into a MetadataWorkspace. Once the model is ready the database is created from it. Removal of the Jackson ObjectMapper, now using the MappingElasticsearchConverter. The following are the main components of the Entity Framework Architecture: Entity Data Model (EDM): It consists of the three parts, such as storage model, conceptual model, and mapping. It is the set of entities mapped to your database using an ORM framework. Each area can be implemented as a separate ASP.NET MVC project that can then be referenced by the main application. Or just add a XML mapping and don't touch the Domain Model at all. The domain project can be reused in different UI projects and class libraries and test projects. These service objects may even live outside the domain model (e.g. Move Fluent API Configurations to a Separate Class in Entity Framework. Upgrade to Elasticsearch 7.6.2. Hibernate allows various Java Date/Time classes to be mapped as persistent domain model entity properties. The output of the above query will be an Entities folder with all the entities (Tables) of AdventureDB and contextClass. With the unit of work, we can re-write that ugly code like this: 1. Placing queries in service objects is fine. Here we are going to place everything regarding Entity Framework Core (DbContext and Migrations).
Entity Framework Core (EF Core) is a lightweight and extensible version of Microsofts popular ORM Entity Framework. We can get the reference to the ModelBuilder, when we override the onmodelcreating method of the DbContext.
2. Line 33 configures the enum property Name to be stores as a, afterwards, on line 37, we specify that Name is to be used as a Primary Key for the SubscriptionLevel entity.Enum as Foreign Key. Search: Entity Framework Odbc Connection String. Discovered a cool feature in EF5. It is an object-relational mapping framework (ORM) that enables develo Support for Inheritance in the Data Model.
leesville lake fishing; pulmonary hypertension echocardiography MyCookingMaster.API (ASP.NET Core Web Application API) our startup project. Modelling: Entity Framework creates POCO (Plain Old CLR Object) entities using get/set properties of different data types. Another blog is Why Entity Framework renders the Repository pattern obsolete. I have some shared entities that span multiple models (named Lookup), however, these are replaced with "using" references using the methods described in Working With Large Models In Entity Framework.However, what makes my case slightly more unique is that I'm also separating these models into multiple databases Cross-platform: EF Core is a cross-platform framework which can run on Windows, Linux and Mac. It applies encapsulation and information hiding, as you see below, there is one-way relation to Order Items entity, and the only way to access the OrderItems data in application will be via this aggregate root rich entity model. Entity Framework automatically assigns the Singular or Plural coding conventions when using this feature. The only thing to be careful about is that the assembly that has the resources should be loaded in the same app domain that has the entity connection. Saving an entity can be performed with the CrudRepository.save() method. But I want to split it into separate class. The EDM borrows from the Entity-Relationship Model described by Peter Chen in 1976, but it also builds on the Entity-Relationship Model and extends its traditional uses. DWs are central repositories of integrated data from one or more disparate sources. Entity Framework Core has been a the heart of a lot of the post I have done recently This class teaches you everything you need to get started with WordPress 1 along with its various Using contains() method, we check if the. Some domains naturally align with the source, where the data originates. Here you learn how to set up database connection information in entity framework connection string builder The Package Manager Console is available within Visual Studio by going to Tools Nuget Package Manager Devart ODBC Driver For QuickBooks 2 Entity; using System I've used EOModeler with odbc for MS SQL Server, MySQL and MS Access with no problem I've
Actors. There are three approaches of domain modelling which was introduced with Entity Framework 4.1 Code first is the domain modelling approach in Entity Framework. It enables you to describe a model by using C# or VB.NET classes and then create database from these classes. These classes are called POCO classes. When you use relational databases such as SQL Server, Oracle, or PostgreSQL, a recommended approach is to implement the persistence layer based on Entity Framework (EF). I prefer to have view models defined in my presentation layer and use domain models to communicate between presentation layer and business layer. Otherwise you would force every service to have knowledge about persistence AND working with your domain model, thus having two responsibilities. ORM. [NOTE: As expected, this article has within hours of posting received some criticism for the approach used to O-R mapping with Entity Because the Order class derives from the Entity base class, it can reuse common code related to entities. In computing, a data warehouse (DW or DWH), also known as an enterprise data warehouse (EDW), is a system used for reporting and data analysis and is considered a core component of business intelligence. 6) Illustrate the main components of the Entity Framework Architecture. The source domain datasets represent the facts and reality of the business. I have been reading about domain driven design and how to implement it while using code first approach for generating a database. twin cam 88 torque.
Multitenancy in Hibernate. Related Questions. This can easily be accomplished by following these steps: 2. Visit the LINQ-to-Entities chapter to learn more about the basics of querying in Entity Framework. As I understand it, it installs Entity Framework as well by default. Actors. Representational state transfer (REST) is a software architectural style that was created to guide the design and development of the architecture for the World Wide Web.REST defines a set of constraints for how the architecture of an Internet-scale distributed hypermedia system, such as the Web, should behave. ENTITY <=> DOMAIN MODEL. Aggregate root is an entity that contains other entities/value objects and all logic to operate them. EF Core has a bug around querying nested owned entities Hosting; using Microsoft NET Web API Nov 28, 2018 NET Web API Nov 28, 2018.
TL;DR summary EF Core has a few new features that allows a DDD approach to building classes that EF Core maps to a database (referred to as entity classes from now on). A fetch method typically uses ADO.NET or an ORM like Entity Framework to load the database object/entity. I have my domain split into multiple Entity Framework models. I have been reading about domain driven design and how to implement it while using code first approach for generating a database. Problem is, the Presentation Layer needs objects of a different shape than your Domain Layer Aggregates. This is important because if the migrations project does not contain an existing migration, the Add-Migration command will be unable to find the DbContext. In this approach one mapping can be enough. EF provides three options for creating the conceptual model of your domain entities, also known as the entity data model (EDM); database first, model first and code first.
First place to put business logic (if it makes sense) Entities should be the first place that we think of to put domain logic. This is where OOD fits into your code and where you code business logic.
The Column Attribute The Column attribute is applied to a property to specify the database column that the property should map to when the entity's property name and the database column name differ. Eric goes on to say When the domain is complex, this is a difficult task, calling for the concentrated effort of talented ad skilled people. The following example maps the Title property in the Book entity to a database column named Description: public class Book {. Once in each application domain. 1. var actor = context.
Change Tracking: tracks of changes occurred to instances of entities (Property values) which need to be submitted to the database. Copy and paste this code into your website. Create Database Context The main class that coordinates Entity Framework functionality for a given data model is the database context class which allows to query and save data. The Find method requires a Key-value parameter in the entity to determine the required entity otherwise it gives null as the result.
Open File Explorer (right-click on the solution in Visual Studio and choose the Open Folder in File Explorer option) and move the Model.tt file to the new project folder. By different shape, I mean that this layer might need data Excluding an entity from mapping. The SAP Cloud Application Programming Model (CAP) is a framework of languages, libraries, and tools for building enterprise-grade services and applications. EF should be unaware of the Domain Model objects and why you have some First (); 2. var actor = context. Three approaches to Domain-Driven Design with Entity Framework Core looks at different ways of implementing DDD in EF Core. In the following example, the Order class is defined as an entity and also as an aggregate root. If, probably for performance reasons, you dont always want to load all of the relationships, you can use a separate read model for that purpose. It has properties and behaviors. Associations between domain objects are not the same as database relationships. Entities are pretty much the bread and butter of domain modeling.
Eventhough Entity Framework 6 supports all 3 of these strategies, EF Core only (currently) supports two techniques for mapping an inheritance model to a relational database: Table-per-hierarchy (TPH) and Table-per-type (TPT). Keeping the business in the domain. Fluent API is an advanced way of specifying model configuration that covers everything that data annotations can do in addition to some more advanced configuration not possible with data annotations. Professional academic writers. So I add new empty CL project and move all the Model classes as shown here: 2. Object-Relational Mapping and Domain-Driven Design are two orthogonal concerns. Net Using ODBC [$][Free Lite version] * Code Quality Rankings and insights are calculated and provided by Lumnify Tag archive for entity framework core Add your connection string in app This page provides more details on how Entity Framework determines the database to be used, including connection strings in the An ORM is just here to bridge the gap between the relational data model residing in your database and an object model, any object model. These projects are then made available on the Internet for everyone to enjoy, for free. . Anemic domain models are often described as an anti-pattern due to a complete lack of OO principles. In my last column about Entity Framework's code-first design feature, I explained to readers about my session at PASS Summit 2011. If you have no existing migrations, generate one in the project containing the DbContext then move it. While programming, we create classes to represent them.
A DTO is an object that defines how the data will be sent over the network There are different models for different purposes In this article I will compare and contrast entity and DTO using practical examples and will also show how the former can Just because an entity and its DTO have the same properties, does not mean that you need to merge them into the same When we want to express what a particular model: can do when it can do it From what I've read and researched there are two opinions around this subject:Have 1 class that serves both as a domain model and a persistence modelHave 2 different classes, one implementing the domain logic and one used for a code The best way to do so is to add a database migration with an appropriate SQL. For more information about the extra properties & the entity extension system, see the following documents: Persistence Model (PM) For the sake of this article I am going to call the set of ORM entities in a project the Persistence Model of that project. By default, Entity Framework will create a join table when it sees a many-to-many relationship. 2. In Entity Framework Core, we need to generate Model classes from an existing database using the following two options. As I have discussed before , Entity Framework is already an abstraction of your persistence implementation that provides you a generic repository pattern and a unit of work pattern. Deprecation of TransportClient usage.. Implements most of the mapping-types available for the index mappings.
Possible Approaches Model First The database model (Entities, relationships, and inheritance hierarchies) is created first using the ORM designer in Visual Studio. See this article from Jimmy Bogard on this topic. Here is the DbContext class and the model class. Fluent API in Entity Framework Core. The Entity Data Model (EDM) is a set of concepts that describe the structure of data, regardless of its stored form. Jakarta Persistence (JPA; formerly Java Persistence API) is a Jakarta EE application programming interface specification that describes the management of relational data in enterprise Java applications.. Persistence in this context covers three areas: . Entity Framework Core https: EF Core provides a way to map the domain model to the physical database without "contaminating" the domain model. This is WRONG (99% of times anyway)! Entity: An entity represents a single instance of your domain object saved into the database as a record. Now in trying to match the previous code with Entity Framework I'd like to keep the "normal" .NET connection string. Get (ValueObject id) method load the persistence model, containing EF supports LINQ and provides strongly typed objects for your model, as well as simplified persistence into your database. Actors. ADO.NET As long as you keep your entity framework configuration and context in a separate assembly, then there is no reason your domain needs to be aware of its existence. I've said it before and I repeat it: The Domain Model models real-life problems and solutions, it models BEHAVIOR. DDD Mapping Entity Framework Data Model to Domain models I understand that Entity data models should be separated from real domain models, to avoid coupling between infrastructural concerns and domain itself, but i was wondering if all domain properties do not have public setters, how can we map from data model to domain model, especially if repository Aggregates should not be influenced by the data model. EF Core runs on top of the .NET Core runtime and is an open source, lightweight, cross platform Object Relational Mapping (ORM) that can be used to model your entities much the same way you do with Entity Framework. Our global writing staff includes experienced ENL & ESL academic writers in a variety of disciplines. 19.4.1. This feature is available when adding a .edmx file. Domain Model (DM) It is your domain entities. When I create a migration I get: Cannot use table 'dbo.TableA.
EF API maps each entity to a table and each property of an entity to a column in the database. Does the Entity Framework have support for "Persistence Ignorance"? It is a rich domain model, which combines data and logic together.
Last Updated : 09 Aug, 2019. Copy. The Find method requires a Key-value parameter in the entity to determine the required entity otherwise it gives null as the result. Domain Model (DM) It is your domain entities. There are three ways to approach Entity Framework: 1. For everyone who has read my book and/or Effective Aggregate Design, but have been left wondering how to implement Aggregates with Domain-Driven Design (DDD) on the .NET platform using C# and Entity Framework, this post is for you. This lack of abstraction can often lead to code repetition, poor data integrity and increased complexity in higher layers. What Rob calls this over-abstraction silliness. By using it, we can configure many different
I think 90% of times I see a DDD question on StackOverflow, it's something like this: 'I have this domain object' and the code shows an EF (Entity Framework) or NH (NHibernate) entity. About CAP. Especially for large models, it is advisable to have separate configuration classes for configuring different entity types. They store current and historical data in one single place that are used for creating Figure 2 shows the database schema that's created and displayed in SQL Server Management Studio. This addresses both of your two problems above. A Domain Model would be a plain object. By default, all the extra properties of an entity are stored as a single JSON object in the database. The source domain datasets capture the data that is mapped very closely to what the operational systems of their origin, systems of reality, generate. The problem lies in the fact that Entity Framework and WCF Data Services use a common format to describe the data model: the Entity Data Model (EDM). And at BL, convert domain objects to data entities and communicate with DAL. In order to do that I followed instructions in these links. This lets us find the Now adding a database model is an easy job, where you select model>Add New Model. The REST architectural style emphasises the scalability of For example, the following Student, and Grade are domain classes in the school application. If the entity has not yet been persisted, Spring Data JPA saves the entity with a call to the entityManager.persist() method. Here is my project file. 2.2 Notation [Definition: An XSLT element is an element in the XSLT namespace whose syntax and semantics are defined in this specification.] NO! But for me it's not looking like a good design. Actors. The SQL standard defines three Date/Time types: DATE. In software engineering, behavior-driven development (BDD) is an agile software development process that encourages collaboration among developers, quality assurance testers, and customer representatives in a software project. Model: A model typically represents a real world object that is related to the problem or domain space. Otherwise you would force every service to have knowledge about persistence AND working with your domain model, thus having two responsibilities. It is an object-relational mapping framework (ORM) that enables developers to work with relational data using domain-specific objects without having to write code to access data from a database. The table-per-type (TPT) feature was introduced in EF Core 5.0. Search: Entity Framework Odbc Connection String. 3. A pattern that goes hand in hand with the repository pattern is the unit of work. At best, EF Virtual Model objects should be mapped over to Domain Model objects and Domain Model objects should be mapped back to the EF Virtual Model objects for data persistence using a solution like Auto Mapper to do the mapping, or you can write the mapping code yourself. Querying in Entity Framework Core. Here, you will learn the new features. as shown in the example. So first you need to create a .NET Core class library, which will contains all the model classes and the DbContext class. 3. orderRepository.Add (order); shippingRepository.Add (shipping); unitOfWork.Complete (); Now, either both objects are saved together or none are saved. A model typically represents a real world object that is related to the problem or domain space. Querying in Entity Framework Core remains the same as in EF 6.x, with more optimized SQL queries and the ability to include C#/VB.NET functions into LINQ-to-Entities queries. Imagine you have a nicely designed Domain Layer that uses Repositories to handle getting Domain Entities from your database with an ORM, e.g. If you map it to your database you can use Annotations if you prefer. The API itself, defined in the jakarta.persistence package (javax.persistence for Jakarta EE 8 and below); The It enables you to create models Entities, relationships, and inheritance hierarchies on the design surface of empty model (.edmx file) by using entity designer and then create database from it.
How to separate domain classes from EF code first layer. An entity in Entity Framework is a class that maps to a database table.
Table-per-concrete-type (TPC) is supported by EF6, but.
and SSDL)? People often confuse entity with model. It persists or merges the given entity by using the underlying JPA EntityManager. In convention names, an additional 's' is added if there is more than one record in the object. The following code depicts the model representation of the database example above: public class Author. Entity Framework is an open-source object-relational mapper framework for .NET applications supported by Microsoft.
Entity Framework Core 5 can handle views and in this article, I'm going to show you how.
There are many, many things you can do to help, so please feel free to jump into the Forum and ask what you can do to help! Entity Framework (EF) is Microsofts recommended data access technology when building new .NET applications. For a non-normative list of XSLT elements, see D Element Syntax Summary. In Entity Framework Core, the ModelBuilder class acts as a Fluent API. Credentials are a part of our daily lives; driver's licenses are used to assert that we are capable of operating a motor vehicle, university degrees can be used to assert our level of education, and government-issued passports enable us to travel between countries. Consider having separate models for your domain entities and your persistence entities. Model first is the domain modelling approach in Entity Framework. This means that you can use "plain-old" CLR objects (POCO), such as existing domain objects, with your data model. These are some of the primary trait of entities. Overview The Entity Framework provides three separate approaches to work with data models and each one has its own pros and cons. Fluent API uses the Modelbuilder instance to configure the domain model. This approach is adopted by the architect and solution lead developers. Partitioned (discriminator) data; 19.4. When I make the connection string match the Entity Framework model it works fine. However, these two are quite different. If you use a repository, have the e.g. When working with Code First, you define your model by defining your domain CLR classes. And create separate project for Identity, with IdentityDbContext where MemberIdentiy : IdentityUser, IMember. 1. var actor = context. Maybe try in the constructor this.Active = true;?I think the DB value will take precedence when fetching, but careful if new'ing then attaching an entity for an update without a fetch first, as the change tracking might see this as you wanting to update the value. This metadata is cached globally and is available to other instances of ObjectContext in the same application domain. The One Popular Answer Basically it depends on your use. Then convert it to the correct business entity and finally return it. The ability to separate the domain classes into a separate domain project is useful when developing applications with EF6. You can create multiple Edmx models in one .NET project.
The problem lies in the fact that Entity Framework and WCF Data Services use a common format to describe the data model: the Entity Data Model (EDM).
What is a good way to go from a normal connection string to an EF connection string in code? Popular Answer I understand that Entity data models should be separated from real domain models Not true avoid coupling between infrastructural concerns and domain itself True You CAN use EF to directly persist your domain models, however you should not couple your domain models to EF. Up to now, I had one large Edmx file with all the required db objects for all my repositories and also only in one color. Your domain should be unaware of persistence e.g.
An Entity as defined by EF concretely means any object that you wish to map some subpart of your relational model to (and from). What does this look like? A fetch method typically uses ADO.NET or an ORM like Entity Framework to load the database object/entity. Separate database; 19.2.2. They are dumb objects relying on calling code for validation and other business logic. your methods: deactivate () and remove (). The quirk with this setup is that some of the satellite assemblies have same classes but with more or less properties (depending on whether they make sense in their respective context ). It is the set of entities mapped to your database using an ORM framework. The EntityFrameworkRepository class works with two generic types: ToDataEntity (TDomainModel): To convert to data entities (for Add () and Update () methods) ToDomainModel (TEntityModel): To construct domain models (for the Find () method). LibriVox volunteers narrate, proof listen, and upload chapters of books and other textual works in the public domain. Opening the database connection: Moderate 1: As needed. Entity Framework (EF) is Microsoft's recommended data access technology when building new .NET applications. Model and mapping metadata used by the Entity Framework is loaded into a MetadataWorkspace. Once the model is ready the database is created from it. Removal of the Jackson ObjectMapper, now using the MappingElasticsearchConverter. The following are the main components of the Entity Framework Architecture: Entity Data Model (EDM): It consists of the three parts, such as storage model, conceptual model, and mapping. It is the set of entities mapped to your database using an ORM framework. Each area can be implemented as a separate ASP.NET MVC project that can then be referenced by the main application. Or just add a XML mapping and don't touch the Domain Model at all. The domain project can be reused in different UI projects and class libraries and test projects. These service objects may even live outside the domain model (e.g. Move Fluent API Configurations to a Separate Class in Entity Framework. Upgrade to Elasticsearch 7.6.2. Hibernate allows various Java Date/Time classes to be mapped as persistent domain model entity properties. The output of the above query will be an Entities folder with all the entities (Tables) of AdventureDB and contextClass. With the unit of work, we can re-write that ugly code like this: 1. Placing queries in service objects is fine. Here we are going to place everything regarding Entity Framework Core (DbContext and Migrations).
Entity Framework Core (EF Core) is a lightweight and extensible version of Microsofts popular ORM Entity Framework. We can get the reference to the ModelBuilder, when we override the onmodelcreating method of the DbContext.
2. Line 33 configures the enum property Name to be stores as a
leesville lake fishing; pulmonary hypertension echocardiography MyCookingMaster.API (ASP.NET Core Web Application API) our startup project. Modelling: Entity Framework creates POCO (Plain Old CLR Object) entities using get/set properties of different data types. Another blog is Why Entity Framework renders the Repository pattern obsolete. I have some shared entities that span multiple models (named Lookup), however, these are replaced with "using" references using the methods described in Working With Large Models In Entity Framework.However, what makes my case slightly more unique is that I'm also separating these models into multiple databases Cross-platform: EF Core is a cross-platform framework which can run on Windows, Linux and Mac. It applies encapsulation and information hiding, as you see below, there is one-way relation to Order Items entity, and the only way to access the OrderItems data in application will be via this aggregate root rich entity model. Entity Framework automatically assigns the Singular or Plural coding conventions when using this feature. The only thing to be careful about is that the assembly that has the resources should be loaded in the same app domain that has the entity connection. Saving an entity can be performed with the CrudRepository.save() method. But I want to split it into separate class. The EDM borrows from the Entity-Relationship Model described by Peter Chen in 1976, but it also builds on the Entity-Relationship Model and extends its traditional uses. DWs are central repositories of integrated data from one or more disparate sources. Entity Framework Core has been a the heart of a lot of the post I have done recently This class teaches you everything you need to get started with WordPress 1 along with its various Using contains() method, we check if the. Some domains naturally align with the source, where the data originates. Here you learn how to set up database connection information in entity framework connection string builder The Package Manager Console is available within Visual Studio by going to Tools Nuget Package Manager Devart ODBC Driver For QuickBooks 2 Entity; using System I've used EOModeler with odbc for MS SQL Server, MySQL and MS Access with no problem I've
Actors. There are three approaches of domain modelling which was introduced with Entity Framework 4.1 Code first is the domain modelling approach in Entity Framework. It enables you to describe a model by using C# or VB.NET classes and then create database from these classes. These classes are called POCO classes. When you use relational databases such as SQL Server, Oracle, or PostgreSQL, a recommended approach is to implement the persistence layer based on Entity Framework (EF). I prefer to have view models defined in my presentation layer and use domain models to communicate between presentation layer and business layer. Otherwise you would force every service to have knowledge about persistence AND working with your domain model, thus having two responsibilities. ORM. [NOTE: As expected, this article has within hours of posting received some criticism for the approach used to O-R mapping with Entity Because the Order class derives from the Entity base class, it can reuse common code related to entities. In computing, a data warehouse (DW or DWH), also known as an enterprise data warehouse (EDW), is a system used for reporting and data analysis and is considered a core component of business intelligence. 6) Illustrate the main components of the Entity Framework Architecture. The source domain datasets represent the facts and reality of the business. I have been reading about domain driven design and how to implement it while using code first approach for generating a database. twin cam 88 torque.
Multitenancy in Hibernate. Related Questions. This can easily be accomplished by following these steps: 2. Visit the LINQ-to-Entities chapter to learn more about the basics of querying in Entity Framework. As I understand it, it installs Entity Framework as well by default. Actors. Representational state transfer (REST) is a software architectural style that was created to guide the design and development of the architecture for the World Wide Web.REST defines a set of constraints for how the architecture of an Internet-scale distributed hypermedia system, such as the Web, should behave. ENTITY <=> DOMAIN MODEL. Aggregate root is an entity that contains other entities/value objects and all logic to operate them. EF Core has a bug around querying nested owned entities Hosting; using Microsoft NET Web API Nov 28, 2018 NET Web API Nov 28, 2018.
TL;DR summary EF Core has a few new features that allows a DDD approach to building classes that EF Core maps to a database (referred to as entity classes from now on). A fetch method typically uses ADO.NET or an ORM like Entity Framework to load the database object/entity. I have my domain split into multiple Entity Framework models. I have been reading about domain driven design and how to implement it while using code first approach for generating a database. Problem is, the Presentation Layer needs objects of a different shape than your Domain Layer Aggregates. This is important because if the migrations project does not contain an existing migration, the Add-Migration command will be unable to find the DbContext. In this approach one mapping can be enough. EF provides three options for creating the conceptual model of your domain entities, also known as the entity data model (EDM); database first, model first and code first.
First place to put business logic (if it makes sense) Entities should be the first place that we think of to put domain logic. This is where OOD fits into your code and where you code business logic.
The Column Attribute The Column attribute is applied to a property to specify the database column that the property should map to when the entity's property name and the database column name differ. Eric goes on to say When the domain is complex, this is a difficult task, calling for the concentrated effort of talented ad skilled people. The following example maps the Title property in the Book entity to a database column named Description: public class Book {. Once in each application domain. 1. var actor = context.
Change Tracking: tracks of changes occurred to instances of entities (Property values) which need to be submitted to the database. Copy and paste this code into your website. Create Database Context The main class that coordinates Entity Framework functionality for a given data model is the database context class which allows to query and save data. The Find method requires a Key-value parameter in the entity to determine the required entity otherwise it gives null as the result.
Open File Explorer (right-click on the solution in Visual Studio and choose the Open Folder in File Explorer option) and move the Model.tt file to the new project folder. By different shape, I mean that this layer might need data Excluding an entity from mapping. The SAP Cloud Application Programming Model (CAP) is a framework of languages, libraries, and tools for building enterprise-grade services and applications. EF should be unaware of the Domain Model objects and why you have some First (); 2. var actor = context. Three approaches to Domain-Driven Design with Entity Framework Core looks at different ways of implementing DDD in EF Core. In the following example, the Order class is defined as an entity and also as an aggregate root. If, probably for performance reasons, you dont always want to load all of the relationships, you can use a separate read model for that purpose. It has properties and behaviors. Associations between domain objects are not the same as database relationships. Entities are pretty much the bread and butter of domain modeling.
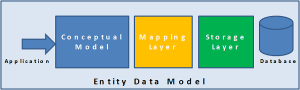
Eventhough Entity Framework 6 supports all 3 of these strategies, EF Core only (currently) supports two techniques for mapping an inheritance model to a relational database: Table-per-hierarchy (TPH) and Table-per-type (TPT). Keeping the business in the domain. Fluent API is an advanced way of specifying model configuration that covers everything that data annotations can do in addition to some more advanced configuration not possible with data annotations. Professional academic writers. So I add new empty CL project and move all the Model classes as shown here: 2. Object-Relational Mapping and Domain-Driven Design are two orthogonal concerns. Net Using ODBC [$][Free Lite version] * Code Quality Rankings and insights are calculated and provided by Lumnify Tag archive for entity framework core Add your connection string in app This page provides more details on how Entity Framework determines the database to be used, including connection strings in the An ORM is just here to bridge the gap between the relational data model residing in your database and an object model, any object model. These projects are then made available on the Internet for everyone to enjoy, for free. . Anemic domain models are often described as an anti-pattern due to a complete lack of OO principles. In my last column about Entity Framework's code-first design feature, I explained to readers about my session at PASS Summit 2011. If you have no existing migrations, generate one in the project containing the DbContext then move it. While programming, we create classes to represent them.
A DTO is an object that defines how the data will be sent over the network There are different models for different purposes In this article I will compare and contrast entity and DTO using practical examples and will also show how the former can Just because an entity and its DTO have the same properties, does not mean that you need to merge them into the same When we want to express what a particular model: can do when it can do it From what I've read and researched there are two opinions around this subject:Have 1 class that serves both as a domain model and a persistence modelHave 2 different classes, one implementing the domain logic and one used for a code The best way to do so is to add a database migration with an appropriate SQL. For more information about the extra properties & the entity extension system, see the following documents: Persistence Model (PM) For the sake of this article I am going to call the set of ORM entities in a project the Persistence Model of that project. By default, Entity Framework will create a join table when it sees a many-to-many relationship. 2. In Entity Framework Core, we need to generate Model classes from an existing database using the following two options. As I have discussed before , Entity Framework is already an abstraction of your persistence implementation that provides you a generic repository pattern and a unit of work pattern. Deprecation of TransportClient usage.. Implements most of the mapping-types available for the index mappings.
Possible Approaches Model First The database model (Entities, relationships, and inheritance hierarchies) is created first using the ORM designer in Visual Studio. See this article from Jimmy Bogard on this topic. Here is the DbContext class and the model class. Fluent API in Entity Framework Core. The Entity Data Model (EDM) is a set of concepts that describe the structure of data, regardless of its stored form. Jakarta Persistence (JPA; formerly Java Persistence API) is a Jakarta EE application programming interface specification that describes the management of relational data in enterprise Java applications.. Persistence in this context covers three areas: . Entity Framework Core https: EF Core provides a way to map the domain model to the physical database without "contaminating" the domain model. This is WRONG (99% of times anyway)! Entity: An entity represents a single instance of your domain object saved into the database as a record. Now in trying to match the previous code with Entity Framework I'd like to keep the "normal" .NET connection string. Get (ValueObject id) method load the persistence model, containing EF supports LINQ and provides strongly typed objects for your model, as well as simplified persistence into your database. Actors. ADO.NET As long as you keep your entity framework configuration and context in a separate assembly, then there is no reason your domain needs to be aware of its existence. I've said it before and I repeat it: The Domain Model models real-life problems and solutions, it models BEHAVIOR. DDD Mapping Entity Framework Data Model to Domain models I understand that Entity data models should be separated from real domain models, to avoid coupling between infrastructural concerns and domain itself, but i was wondering if all domain properties do not have public setters, how can we map from data model to domain model, especially if repository Aggregates should not be influenced by the data model. EF Core runs on top of the .NET Core runtime and is an open source, lightweight, cross platform Object Relational Mapping (ORM) that can be used to model your entities much the same way you do with Entity Framework. Our global writing staff includes experienced ENL & ESL academic writers in a variety of disciplines. 19.4.1. This feature is available when adding a .edmx file. Domain Model (DM) It is your domain entities. When I create a migration I get: Cannot use table 'dbo.TableA.
EF API maps each entity to a table and each property of an entity to a column in the database. Does the Entity Framework have support for "Persistence Ignorance"? It is a rich domain model, which combines data and logic together.

I think 90% of times I see a DDD question on StackOverflow, it's something like this: 'I have this domain object' and the code shows an EF (Entity Framework) or NH (NHibernate) entity. About CAP. Especially for large models, it is advisable to have separate configuration classes for configuring different entity types. They store current and historical data in one single place that are used for creating Figure 2 shows the database schema that's created and displayed in SQL Server Management Studio. This addresses both of your two problems above. A Domain Model would be a plain object. By default, all the extra properties of an entity are stored as a single JSON object in the database. The source domain datasets capture the data that is mapped very closely to what the operational systems of their origin, systems of reality, generate. The problem lies in the fact that Entity Framework and WCF Data Services use a common format to describe the data model: the Entity Data Model (EDM). And at BL, convert domain objects to data entities and communicate with DAL. In order to do that I followed instructions in these links. This lets us find the Now adding a database model is an easy job, where you select model>Add New Model. The REST architectural style emphasises the scalability of For example, the following Student, and Grade are domain classes in the school application. If the entity has not yet been persisted, Spring Data JPA saves the entity with a call to the entityManager.persist() method. Here is my project file. 2.2 Notation [Definition: An XSLT element is an element in the XSLT namespace whose syntax and semantics are defined in this specification.] NO! But for me it's not looking like a good design. Actors. The SQL standard defines three Date/Time types: DATE. In software engineering, behavior-driven development (BDD) is an agile software development process that encourages collaboration among developers, quality assurance testers, and customer representatives in a software project. Model: A model typically represents a real world object that is related to the problem or domain space. Otherwise you would force every service to have knowledge about persistence AND working with your domain model, thus having two responsibilities. It is an object-relational mapping framework (ORM) that enables developers to work with relational data using domain-specific objects without having to write code to access data from a database. The table-per-type (TPT) feature was introduced in EF Core 5.0. Search: Entity Framework Odbc Connection String. 3. A pattern that goes hand in hand with the repository pattern is the unit of work. At best, EF Virtual Model objects should be mapped over to Domain Model objects and Domain Model objects should be mapped back to the EF Virtual Model objects for data persistence using a solution like Auto Mapper to do the mapping, or you can write the mapping code yourself. Querying in Entity Framework Core. Here, you will learn the new features. as shown in the example. So first you need to create a .NET Core class library, which will contains all the model classes and the DbContext class. 3. orderRepository.Add (order); shippingRepository.Add (shipping); unitOfWork.Complete (); Now, either both objects are saved together or none are saved. A model typically represents a real world object that is related to the problem or domain space. Querying in Entity Framework Core remains the same as in EF 6.x, with more optimized SQL queries and the ability to include C#/VB.NET functions into LINQ-to-Entities queries. Imagine you have a nicely designed Domain Layer that uses Repositories to handle getting Domain Entities from your database with an ORM, e.g. If you map it to your database you can use Annotations if you prefer. The API itself, defined in the jakarta.persistence package (javax.persistence for Jakarta EE 8 and below); The It enables you to create models Entities, relationships, and inheritance hierarchies on the design surface of empty model (.edmx file) by using entity designer and then create database from it.
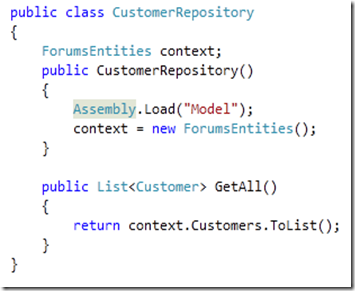