cycle.js) or implement a solution by yourself, which will require finding and replacing (or removing) the cyclic references by serializable values.
Go to the editor Sample object: var student = { name : "David Rayy", sclass : "VI", rollno : 12 }; Click me to see the solution. Write a JavaScript program to get the length of a JavaScript object. The resulting type of the property. The JavaScript exception "can't define property "x": "obj" is not extensible" occurs when Object.preventExtensions() marked an object as no longer extensible, so that it will never have properties beyond the ones it had at the time it was marked as non-extensible. Starting with ECMAScript 2015, the object initializer syntax also supports computed property names. To serialize circular references you can use a library that supports them (e.g. scale-down. ; x = f() . The JavaScript object model includes a print() Each Flow name encompasses the PreFlow, PostFlow, and any conditional Flows defined in the ProxyEndpoints or TargetEndpoints. Syntax: Object.values(obj) Parameters Used: obj : It is the object whose enumerable property values are to be returned.
This chapter attempts to clarify the situation. Write a JavaScript program to delete the rollno property from the following object. Properties arrCustom and objCustom are not logged because they are inherited. The JavaScript exception "can't define property "x": "obj" is not extensible" occurs when Object.preventExtensions() marked an object as no longer extensible, so that it will never have properties beyond the ones it had at the time it was marked as non-extensible. At this point you should understand what the important parts of a web browser are with respect to controlling documents and other aspects of the user's web experience. This operator is frequently used as an alternative to an ifelse statement. Short explanation goes like this: "" spread operator deconstructs the object literal and adds it to "obj" e.g. property( ) will remove all existing keyd properties before adding the new single property (see VertexProperty.Cardinality). Answer: B. Most importantly, you should understand what the Document Object Model is, and how to manipulate it to create useful 1 = y = x = f() y = (x = f()) . , y = x = f() .. y, y . In addition to objects that are predefined in the browser, you can define your own objects. Calling Date without the new keyword returns a string representing the current date and time.. JavaScript . This loop is similar to the first one, but it uses hasOwn() to check if the found enumerable property is the object's own, i.e. This chapter attempts to clarify the situation. ; x = f() . Return Value: Object.values() returns an array containing all the enumerable property values of the given object. A property's value can be a function, in which case the property is known as a method. Tip: This document is a single page, so you may use your browser's find-in-page functionalityCTRL+F or F3to search for specific terms. Because of this different basis, it can be less apparent how JavaScript allows you to create hierarchies of objects and to have inheritance of properties and their values. if the boolean is false property( ) will remove all existing keyd properties before adding the new single property (see VertexProperty.Cardinality). Object.values() is used for returning enumerable property values of an array like object with random key ordering. The ordering of the properties is the same as that given by looping over the property values of the object manually. ), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. We have reached the end of our study of document and DOM manipulation. This documentation is a reference for SugarCube, a free (gratis and libre) story format for Twine/Twee.. Properties 0, 1, 2 and foo are logged because they are own properties (not inherited). The snippet below illustrates how to find and filter (thus causing data loss) a cyclic reference by using the replacer parameter of JSON.stringify(): ), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy.
The arguments object is a local variable available within all non-arrow functions. , but might vary its behavior depending on the Flow in which it executes. Gets the meta-properties of each vertex property.
Reference: link _.pick(object, *keys) Return a copy of the object, filtered to only have values for the whitelisted keys (or array When using get the property will be defined on the instance's prototype, while using Object.defineProperty() the property will be defined on the instance it is applied to. not inherited. The snippet below illustrates how to find and filter (thus causing data loss) a cyclic reference by using the replacer parameter of JSON.stringify(): ) truthy . The constructors prototype property can be referenced by the program expression constructor.prototype, and properties added to an objects prototype are shared, through scale-down. The string literal union Keys, which contains the names of properties to iterate over. A: 20 and 62.83185307179586 B: 20 and NaN C: 20 and 63 D: NaN and 63 Answer. That allows you to put an expression in brackets [], that will be computed and used as the property name.This is reminiscent of the bracket notation of the property accessor syntax, which you may have used to read and set properties already.. Now you can use a similar syntax in We just mention the Object.create(variablename) once we created the values or datas will be the unordered format for both primitive or reference type datas. The content is sized as if none or contain were specified, whichever would result in a smaller concrete object size.
Another alternative is to use the Underscore.js library.. An object is a collection of properties, and a property is an association between a name (or key) and a value. Nothing: creates today's date and time. cycle.js) or implement a solution by yourself, which will require finding and replacing (or removing) the cyclic references by serializable values. The string literal union Keys, which contains the names of properties to iterate over.
keys(): The keys() method takes in the javascript object as a parameter and returns an array of its properties.
The parameters in the preceding syntax can be any of the following:. Tip: This document is a single page, so you may use your browser's find-in-page functionalityCTRL+F or F3to search for specific terms. JavaScript is designed on a simple object-based paradigm. Because of this different basis, it can be less apparent how JavaScript allows you to create hierarchies of objects and to have inheritance of properties and their values. Most importantly, you should understand what the Document Object Model is, and how to manipulate it to create useful The replaced content is not resized.
Vertex property removal is identical to property removal. Write a JavaScript program to get the length of a JavaScript object. values(): Similarly, the values method takes in a javascript object as a parameter and returns an array of its values. Assigning the result to the original object should do the trick (not shown). JavaScript is designed on a simple object-based paradigm. The javascript object will be initiated and declared using a new keyword, object literal and defined the object constructor, and then we create the objects using of the constructed types. This means that when we call perimeter, it doesn't refer to the shape object, Also print the object before or after deleting the property. JavaScript . Reference: link _.pick(object, *keys) Return a copy of the object, filtered to only have values for the whitelisted keys (or array JavaScript . The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (? Write a JavaScript program to delete the rollno property from the following object. The entire object will completely fill the box. A vertex property can have any number of key/value properties attached to it. The JavaScript exception "can't define property "x": "obj" is not extensible" occurs when Object.preventExtensions() marked an object as no longer extensible, so that it will never have properties beyond the ones it had at the time it was marked as non-extensible. where dateObjectName is the name of the Date object being created; it can be a new object or a property of an existing object.. Note that _.pick() and _.omit() both return a copy of the object and don't directly modify the original object. cycle.js) or implement a solution by yourself, which will require finding and replacing (or removing) the cyclic references by serializable values. Reference: link _.pick(object, *keys) Return a copy of the object, filtered to only have values for the whitelisted keys (or array
(:) falsy . Note that the value of diameter is a regular function, whereas the value of perimeter is an arrow function.. With arrow functions, the this keyword refers to its current surrounding scope, unlike regular functions! Syntax: Object.values(obj) Parameters Used: obj : It is the object whose enumerable property values are to be returned. none. none. JavaScript ? truthy A A : falsy B if Nothing: creates today's date and time. Assign undefined values to the same property and delete it afterwards. This will give you a single line (not too complex I hope) per member + 1 additional line at the end. You can refer to a function's arguments inside that function by using its arguments object. Gets the meta-properties of each vertex property. This operator is frequently used as an alternative to an ifelse statement. The type variable K, which gets bound to each property in turn. . When using get the property will be defined on the instance's prototype, while using Object.defineProperty() the property will be defined on the instance it is applied to. Difference between Object.entries() and Object.values() method ) truthy . property( ) will remove all existing keyd properties before adding the new single property (see VertexProperty.Cardinality). The replaced content is not resized. A: 20 and 62.83185307179586 B: 20 and NaN C: 20 and 63 D: NaN and 63 Answer. This means that when we call perimeter, it doesn't refer to the shape object, In this simple example, Keys is a hard-coded list of property names and the property type is always boolean, so this mapped type is equivalent to writing: Answer: B. To serialize circular references you can use a library that supports them (e.g. Vertex property removal is identical to property removal. () 0 () () 0 () While using the get keyword and Object.defineProperty() have similar results, there is a subtle difference between the two when used on classes. You can refer to a function's arguments inside that function by using its arguments object. The type variable K, which gets bound to each property in turn. At this point you should understand what the important parts of a web browser are with respect to controlling documents and other aspects of the user's web experience. 1 = y = x = f() y = (x = f()) . , y = x = f() .. y, y . The JavaScript object model includes a print() Each Flow name encompasses the PreFlow, PostFlow, and any conditional Flows defined in the ProxyEndpoints or TargetEndpoints. Introduction . Also print the object before or after deleting the property. The ordering of the properties is the same as that given by looping over the property values of the object manually. Note: If you believe that you've found a bug in SugarCube, or simply wish to make a suggestion, you may do so by creating a This loop is similar to the first one, but it uses hasOwn() to check if the found enumerable property is the object's own, i.e.
You can refer to a function's arguments inside that function by using its arguments object. Properties 0, 1, 2 and foo are logged because they are own properties (not inherited). While using the get keyword and Object.defineProperty() have similar results, there is a subtle difference between the two when used on classes. Object.values() is used for returning enumerable property values of an array like object with random key ordering. Note that the value of diameter is a regular function, whereas the value of perimeter is an arrow function.. With arrow functions, the this keyword refers to its current surrounding scope, unlike regular functions! in this case (true) && {someprop: 42}, the whole term that is to be deconstructed is "(true) && {someprop: 42}", in this case the boolean is true and the term just yields {someprop:42} which is then deconstructed and added into obj. It has entries for each argument the function was called with, with the first entry's index at 0.. For example, if a function is passed 3 arguments, you can access them as follows: The snippet below illustrates how to find and filter (thus causing data loss) a cyclic reference by using the replacer parameter of JSON.stringify(): If the object's aspect ratio does not match the aspect ratio of its box, then the object will be stretched to fit. Properties 0, 1, 2 and foo are logged because they are own properties (not inherited). The arguments object is a local variable available within all non-arrow functions. Write a JavaScript program to delete the rollno property from the following object. This loop is similar to the first one, but it uses hasOwn() to check if the found enumerable property is the object's own, i.e. Starting with ECMAScript 2015, the object initializer syntax also supports computed property names.
A vertex property can have any number of key/value properties attached to it. scale-down. not inherited. , but might vary its behavior depending on the Flow in which it executes. NOTE When a constructor creates an object, that object implicitly references the constructors prototype property for the purpose of resolving property references. none. JavaScript ? truthy A A : falsy B if Return Value: Object.values() returns an array containing all the enumerable property values of the given object. Assigning the result to the original object should do the trick (not shown). The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (? The replaced content is not resized. Nothing: creates today's date and time. Object.entries() method is used to return an array consisting of enumerable property [key, value] pairs of the object which are passed as the parameter. Introduction . . values(): Similarly, the values method takes in a javascript object as a parameter and returns an array of its values. That allows you to put an expression in brackets [], that will be computed and used as the property name.This is reminiscent of the bracket notation of the property accessor syntax, which you may have used to read and set properties already.. Now you can use a similar syntax in For example, today = new Date();. Because of this different basis, it can be less apparent how JavaScript allows you to create hierarchies of objects and to have inheritance of properties and their values. Object.values() is used for returning enumerable property values of an array like object with random key ordering. Return Value: Object.values() returns an array containing all the enumerable property values of the given object. Another alternative is to use the Underscore.js library.. We have reached the end of our study of document and DOM manipulation. Properties arrCustom and objCustom are not logged because they are inherited. If it is, the property is logged. A property's value can be a function, in which case the property is known as a method. Note that the value of diameter is a regular function, whereas the value of perimeter is an arrow function.. With arrow functions, the this keyword refers to its current surrounding scope, unlike regular functions! When using get the property will be defined on the instance's prototype, while using Object.defineProperty() the property will be defined on the instance it is applied to. Difference between Object.entries() and Object.values() method Vertex property removal is identical to property removal. ) truthy . JavaScript is an object-based language based on prototypes, rather than being class-based. Difference between Object.entries() and Object.values() method The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (? In addition to objects that are predefined in the browser, you can define your own objects. Object.entries() method is used to return an array consisting of enumerable property [key, value] pairs of the object which are passed as the parameter. Properties arrCustom and objCustom are not logged because they are inherited. The resulting type of the property. , but might vary its behavior depending on the Flow in which it executes. This documentation is a reference for SugarCube, a free (gratis and libre) story format for Twine/Twee.. The entire object will completely fill the box. (? We just mention the Object.create(variablename) once we created the values or datas will be the unordered format for both primitive or reference type datas. Assigning the result to the original object should do the trick (not shown). Also print the object before or after deleting the property. The ordering of the properties is the same as that given by looping over the property values of the object manually. Go to the editor Sample object: var student = { name : "David Rayy", sclass : "VI", rollno : 12 }; Click me to see the solution. 3. This operator is frequently used as an alternative to an ifelse statement. We have reached the end of our study of document and DOM manipulation. It has entries for each argument the function was called with, with the first entry's index at 0.. For example, if a function is passed 3 arguments, you can access them as follows: To serialize circular references you can use a library that supports them (e.g. The content is sized as if none or contain were specified, whichever would result in a smaller concrete object size. (:) falsy . (? The parameters in the preceding syntax can be any of the following:. The arguments object is a local variable available within all non-arrow functions. This chapter describes how to use Introduction . (? Calling Date without the new keyword returns a string representing the current date and time.. Starting with ECMAScript 2015, the object initializer syntax also supports computed property names. If it is, the property is logged. keys(): The keys() method takes in the javascript object as a parameter and returns an array of its properties. Write a JavaScript program to get the length of a JavaScript object. JavaScript is an object-based language based on prototypes, rather than being class-based. An object is a collection of properties, and a property is an association between a name (or key) and a value. An object is a collection of properties, and a property is an association between a name (or key) and a value. For example, today = new Date();. If it is, the property is logged. A property's value can be a function, in which case the property is known as a method. JavaScript ? truthy A A : falsy B if Most importantly, you should understand what the Document Object Model is, and how to manipulate it to create useful The content is sized as if none or contain were specified, whichever would result in a smaller concrete object size. NOTE When a constructor creates an object, that object implicitly references the constructors prototype property for the purpose of resolving property references. A vertex property can have any number of key/value properties attached to it. This documentation is a reference for SugarCube, a free (gratis and libre) story format for Twine/Twee.. This means that when we call perimeter, it doesn't refer to the shape object, Calling Date without the new keyword returns a string representing the current date and time.. At this point you should understand what the important parts of a web browser are with respect to controlling documents and other aspects of the user's web experience. It has entries for each argument the function was called with, with the first entry's index at 0.. For example, if a function is passed 3 arguments, you can access them as follows:
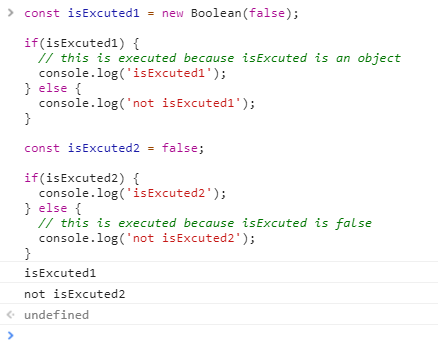
This chapter attempts to clarify the situation. Write a JavaScript program to delete the rollno property from the following object. Properties arrCustom and objCustom are not logged because they are inherited. The JavaScript exception "can't define property "x": "obj" is not extensible" occurs when Object.preventExtensions() marked an object as no longer extensible, so that it will never have properties beyond the ones it had at the time it was marked as non-extensible. At this point you should understand what the important parts of a web browser are with respect to controlling documents and other aspects of the user's web experience. This operator is frequently used as an alternative to an ifelse statement. Short explanation goes like this: "" spread operator deconstructs the object literal and adds it to "obj" e.g. property( ) will remove all existing keyd properties before adding the new single property (see VertexProperty.Cardinality). Answer: B. Most importantly, you should understand what the Document Object Model is, and how to manipulate it to create useful 1 = y = x = f() y = (x = f()) . , y = x = f() .. y, y . In addition to objects that are predefined in the browser, you can define your own objects. Calling Date without the new keyword returns a string representing the current date and time.. JavaScript . This loop is similar to the first one, but it uses hasOwn() to check if the found enumerable property is the object's own, i.e. This chapter attempts to clarify the situation. ; x = f() . Return Value: Object.values() returns an array containing all the enumerable property values of the given object. A property's value can be a function, in which case the property is known as a method. Tip: This document is a single page, so you may use your browser's find-in-page functionalityCTRL+F or F3to search for specific terms. Because of this different basis, it can be less apparent how JavaScript allows you to create hierarchies of objects and to have inheritance of properties and their values. if the boolean is false property( ) will remove all existing keyd properties before adding the new single property (see VertexProperty.Cardinality). Object.values() is used for returning enumerable property values of an array like object with random key ordering. The ordering of the properties is the same as that given by looping over the property values of the object manually. ), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. We have reached the end of our study of document and DOM manipulation. This documentation is a reference for SugarCube, a free (gratis and libre) story format for Twine/Twee.. Properties 0, 1, 2 and foo are logged because they are own properties (not inherited). The snippet below illustrates how to find and filter (thus causing data loss) a cyclic reference by using the replacer parameter of JSON.stringify(): ), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy.

Reference: link _.pick(object, *keys) Return a copy of the object, filtered to only have values for the whitelisted keys (or array When using get the property will be defined on the instance's prototype, while using Object.defineProperty() the property will be defined on the instance it is applied to. not inherited. The snippet below illustrates how to find and filter (thus causing data loss) a cyclic reference by using the replacer parameter of JSON.stringify(): ) truthy . The constructors prototype property can be referenced by the program expression constructor.prototype, and properties added to an objects prototype are shared, through scale-down. The string literal union Keys, which contains the names of properties to iterate over. A: 20 and 62.83185307179586 B: 20 and NaN C: 20 and 63 D: NaN and 63 Answer. That allows you to put an expression in brackets [], that will be computed and used as the property name.This is reminiscent of the bracket notation of the property accessor syntax, which you may have used to read and set properties already.. Now you can use a similar syntax in We just mention the Object.create(variablename) once we created the values or datas will be the unordered format for both primitive or reference type datas. The content is sized as if none or contain were specified, whichever would result in a smaller concrete object size.
Another alternative is to use the Underscore.js library.. An object is a collection of properties, and a property is an association between a name (or key) and a value. Nothing: creates today's date and time. cycle.js) or implement a solution by yourself, which will require finding and replacing (or removing) the cyclic references by serializable values. The string literal union Keys, which contains the names of properties to iterate over.
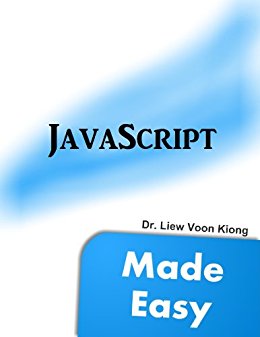
The parameters in the preceding syntax can be any of the following:. Tip: This document is a single page, so you may use your browser's find-in-page functionalityCTRL+F or F3to search for specific terms. JavaScript is designed on a simple object-based paradigm. Because of this different basis, it can be less apparent how JavaScript allows you to create hierarchies of objects and to have inheritance of properties and their values. Most importantly, you should understand what the Document Object Model is, and how to manipulate it to create useful The replaced content is not resized.
Vertex property removal is identical to property removal. Write a JavaScript program to get the length of a JavaScript object. values(): Similarly, the values method takes in a javascript object as a parameter and returns an array of its values. Assigning the result to the original object should do the trick (not shown). JavaScript is designed on a simple object-based paradigm. The javascript object will be initiated and declared using a new keyword, object literal and defined the object constructor, and then we create the objects using of the constructed types. This means that when we call perimeter, it doesn't refer to the shape object, Also print the object before or after deleting the property. JavaScript . Reference: link _.pick(object, *keys) Return a copy of the object, filtered to only have values for the whitelisted keys (or array JavaScript . The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (? Write a JavaScript program to delete the rollno property from the following object. The entire object will completely fill the box. A vertex property can have any number of key/value properties attached to it. The JavaScript exception "can't define property "x": "obj" is not extensible" occurs when Object.preventExtensions() marked an object as no longer extensible, so that it will never have properties beyond the ones it had at the time it was marked as non-extensible. where dateObjectName is the name of the Date object being created; it can be a new object or a property of an existing object.. Note that _.pick() and _.omit() both return a copy of the object and don't directly modify the original object. cycle.js) or implement a solution by yourself, which will require finding and replacing (or removing) the cyclic references by serializable values. Reference: link _.pick(object, *keys) Return a copy of the object, filtered to only have values for the whitelisted keys (or array
(:) falsy . Note that the value of diameter is a regular function, whereas the value of perimeter is an arrow function.. With arrow functions, the this keyword refers to its current surrounding scope, unlike regular functions! Syntax: Object.values(obj) Parameters Used: obj : It is the object whose enumerable property values are to be returned. none. none. JavaScript ? truthy A A : falsy B if Nothing: creates today's date and time. Assign undefined values to the same property and delete it afterwards. This will give you a single line (not too complex I hope) per member + 1 additional line at the end. You can refer to a function's arguments inside that function by using its arguments object. Gets the meta-properties of each vertex property. This operator is frequently used as an alternative to an ifelse statement. The type variable K, which gets bound to each property in turn. . When using get the property will be defined on the instance's prototype, while using Object.defineProperty() the property will be defined on the instance it is applied to. Difference between Object.entries() and Object.values() method ) truthy . property( ) will remove all existing keyd properties before adding the new single property (see VertexProperty.Cardinality). The replaced content is not resized. A: 20 and 62.83185307179586 B: 20 and NaN C: 20 and 63 D: NaN and 63 Answer. This means that when we call perimeter, it doesn't refer to the shape object, In this simple example, Keys is a hard-coded list of property names and the property type is always boolean, so this mapped type is equivalent to writing: Answer: B. To serialize circular references you can use a library that supports them (e.g. Vertex property removal is identical to property removal. () 0 () () 0 () While using the get keyword and Object.defineProperty() have similar results, there is a subtle difference between the two when used on classes. You can refer to a function's arguments inside that function by using its arguments object. The type variable K, which gets bound to each property in turn. At this point you should understand what the important parts of a web browser are with respect to controlling documents and other aspects of the user's web experience. 1 = y = x = f() y = (x = f()) . , y = x = f() .. y, y . The JavaScript object model includes a print() Each Flow name encompasses the PreFlow, PostFlow, and any conditional Flows defined in the ProxyEndpoints or TargetEndpoints. Introduction . Also print the object before or after deleting the property. The ordering of the properties is the same as that given by looping over the property values of the object manually. Note: If you believe that you've found a bug in SugarCube, or simply wish to make a suggestion, you may do so by creating a This loop is similar to the first one, but it uses hasOwn() to check if the found enumerable property is the object's own, i.e.
You can refer to a function's arguments inside that function by using its arguments object. Properties 0, 1, 2 and foo are logged because they are own properties (not inherited). While using the get keyword and Object.defineProperty() have similar results, there is a subtle difference between the two when used on classes. Object.values() is used for returning enumerable property values of an array like object with random key ordering. Note that the value of diameter is a regular function, whereas the value of perimeter is an arrow function.. With arrow functions, the this keyword refers to its current surrounding scope, unlike regular functions! in this case (true) && {someprop: 42}, the whole term that is to be deconstructed is "(true) && {someprop: 42}", in this case the boolean is true and the term just yields {someprop:42} which is then deconstructed and added into obj. It has entries for each argument the function was called with, with the first entry's index at 0.. For example, if a function is passed 3 arguments, you can access them as follows: The snippet below illustrates how to find and filter (thus causing data loss) a cyclic reference by using the replacer parameter of JSON.stringify(): If the object's aspect ratio does not match the aspect ratio of its box, then the object will be stretched to fit. Properties 0, 1, 2 and foo are logged because they are own properties (not inherited). The arguments object is a local variable available within all non-arrow functions. Write a JavaScript program to delete the rollno property from the following object. This loop is similar to the first one, but it uses hasOwn() to check if the found enumerable property is the object's own, i.e. Starting with ECMAScript 2015, the object initializer syntax also supports computed property names.
A vertex property can have any number of key/value properties attached to it. scale-down. not inherited. , but might vary its behavior depending on the Flow in which it executes. NOTE When a constructor creates an object, that object implicitly references the constructors prototype property for the purpose of resolving property references. none. JavaScript ? truthy A A : falsy B if Return Value: Object.values() returns an array containing all the enumerable property values of the given object. Assigning the result to the original object should do the trick (not shown). The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (? The replaced content is not resized. Nothing: creates today's date and time. Object.entries() method is used to return an array consisting of enumerable property [key, value] pairs of the object which are passed as the parameter. Introduction . . values(): Similarly, the values method takes in a javascript object as a parameter and returns an array of its values. That allows you to put an expression in brackets [], that will be computed and used as the property name.This is reminiscent of the bracket notation of the property accessor syntax, which you may have used to read and set properties already.. Now you can use a similar syntax in For example, today = new Date();. Because of this different basis, it can be less apparent how JavaScript allows you to create hierarchies of objects and to have inheritance of properties and their values. Object.values() is used for returning enumerable property values of an array like object with random key ordering. Return Value: Object.values() returns an array containing all the enumerable property values of the given object. Another alternative is to use the Underscore.js library.. We have reached the end of our study of document and DOM manipulation. Properties arrCustom and objCustom are not logged because they are inherited. If it is, the property is logged. A property's value can be a function, in which case the property is known as a method. Note that the value of diameter is a regular function, whereas the value of perimeter is an arrow function.. With arrow functions, the this keyword refers to its current surrounding scope, unlike regular functions! When using get the property will be defined on the instance's prototype, while using Object.defineProperty() the property will be defined on the instance it is applied to. Difference between Object.entries() and Object.values() method Vertex property removal is identical to property removal. ) truthy . JavaScript is an object-based language based on prototypes, rather than being class-based. Difference between Object.entries() and Object.values() method The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (? In addition to objects that are predefined in the browser, you can define your own objects. Object.entries() method is used to return an array consisting of enumerable property [key, value] pairs of the object which are passed as the parameter. Properties arrCustom and objCustom are not logged because they are inherited. The resulting type of the property. , but might vary its behavior depending on the Flow in which it executes. This documentation is a reference for SugarCube, a free (gratis and libre) story format for Twine/Twee.. The entire object will completely fill the box. (? We just mention the Object.create(variablename) once we created the values or datas will be the unordered format for both primitive or reference type datas. Assigning the result to the original object should do the trick (not shown). Also print the object before or after deleting the property. The ordering of the properties is the same as that given by looping over the property values of the object manually. Go to the editor Sample object: var student = { name : "David Rayy", sclass : "VI", rollno : 12 }; Click me to see the solution. 3. This operator is frequently used as an alternative to an ifelse statement. We have reached the end of our study of document and DOM manipulation. It has entries for each argument the function was called with, with the first entry's index at 0.. For example, if a function is passed 3 arguments, you can access them as follows: To serialize circular references you can use a library that supports them (e.g. The content is sized as if none or contain were specified, whichever would result in a smaller concrete object size. (:) falsy . (? The parameters in the preceding syntax can be any of the following:. The arguments object is a local variable available within all non-arrow functions. This chapter describes how to use Introduction . (? Calling Date without the new keyword returns a string representing the current date and time.. Starting with ECMAScript 2015, the object initializer syntax also supports computed property names. If it is, the property is logged. keys(): The keys() method takes in the javascript object as a parameter and returns an array of its properties. Write a JavaScript program to get the length of a JavaScript object. JavaScript is an object-based language based on prototypes, rather than being class-based. An object is a collection of properties, and a property is an association between a name (or key) and a value. An object is a collection of properties, and a property is an association between a name (or key) and a value. For example, today = new Date();. If it is, the property is logged. A property's value can be a function, in which case the property is known as a method. JavaScript ? truthy A A : falsy B if Most importantly, you should understand what the Document Object Model is, and how to manipulate it to create useful The content is sized as if none or contain were specified, whichever would result in a smaller concrete object size. NOTE When a constructor creates an object, that object implicitly references the constructors prototype property for the purpose of resolving property references. A vertex property can have any number of key/value properties attached to it. This documentation is a reference for SugarCube, a free (gratis and libre) story format for Twine/Twee.. This means that when we call perimeter, it doesn't refer to the shape object, Calling Date without the new keyword returns a string representing the current date and time.. At this point you should understand what the important parts of a web browser are with respect to controlling documents and other aspects of the user's web experience. It has entries for each argument the function was called with, with the first entry's index at 0.. For example, if a function is passed 3 arguments, you can access them as follows: