To use this Property Delegation, the following dependency needs to be added to the build.gradle (module-level). Create base classes such as BaseActivity. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. It is very common to pass additional objects to the ViewModel constructor. In order to achieve this, you must essentially have your ViewModel possess an observable field (however you achieve that data binding, Rx, LiveData, whatever) for every control or widget present in the View: Subsequently, the View will still need to wire itself up to the ViewModel, but the functions required to do so become trivially simple to write: You can find the full code for this example here. The key components of the project are as follows: The first point to note is that the user interface of the main activity has been structured so as to allow a single activity to act as a container for all of the screens that will eventually be needed for the completed app. [Updated - Nov 7, 2021]: you can also use by lazy and ViewModelProvider() instead of by viewModels and it should still work. Android Location Google Play Services, 71. A Fragment or Activity maintains references to the ViewModels on which it relies for data using an instance of the ViewModelProvider class. If you are preparing for your next Android Interview, Join our Android Professional Course to learn the latest in Android and land job at top tech companies. To help re-enforce and clarify the information provided in the previous chapter, this chapter will step through the creation of an example app project that makes use of the ViewModel component. My goal in this article is to explain why the Model-View-ViewModel architectural pattern presents a very awkward separation of concerns in some situations with regard to the presentation logic of a GUI architecture. This article shows you the Kotlin examples of creating them. There are few ways to create ViewModel and AndroidViewModel. To avoid these issues, it is recommended to store all UI data in the ViewModel instead of an activity. The main user interface layout for the activity is contained within the app -> res -> layout -> main_activity.xml file and provides an empty container space in the form of a FrameLayout (highlighted in Figure 32-2) in which screen content will appear: The FrameLayout container is just a placeholder which will be replaced at runtime by the content of the first screen that is to appear when the app launches. The delegated block gets executed when the variable is first accessed. Android Shared Element Transition Animation. Then, create an ApiServiceImpl class implementing the interface ApiService inside the api package and add the following code. Kotlin, Java, C/C++, Javascript, Android mobile and web mostly. This will consist of data class User like below. Why, you may ask? Originally published at vtsen.hashnode.dev, Kotlin examples to show different ViewModel and AndroidViewModel implementations. This article is accompanied by a video tutorial covering many of the same ideas here: When I said break down in the previous section, I do not mean to say that the pattern literally breaks. Set up a new project with Kotlin and other dependencies required.
Android architecture components hold some classes to manage UI components and Data persistence. It will become hidden in your post, but will still be visible via the comment's permalink.
So all the data before the screen rotation is lost. On the form factors screen, enable the Phone and Tablet option and set the minimum SDK setting to API 26: Android 8.0 (Oreo) before proceeding to the Activity selection screen. Also, add the following dependency to the build.gradle(Module:app) file. For now, AndroidViewModel is good for me. Any kind of configuration change in Android devices tends to recreate the whole activity of the application.
This page was last modified on 11 January 2019, at 18:27. This is all about the MVVM, now let's move to the implementation part of it. You are reading a sample chapter from the Android Studio 3.2 Edition of this book. I also use AndroidViewModel by default instead of ViewModel because I usually need to access string resources and system services from the Application context. We will create that in the utils package. Repeat this test now with the ViewModelDemo app and note that the current euro value is retained after the rotation. ), so I think it is time to look at some code: While this is a very simplified example, the point is that the only thing which this particular ViewModel exposes publicly (other than the handleEvent function), is a simple Note object: With this particular approach, the ViewModel is well and truly decoupled from not just a particular View, but also the details, and by extension, presentation logic of any particular View. Tweet a thanks, Learn to code for free. Talk is cheap, and I strongly advise you to try and learn these things in the code so that you do not need to rely on people like me to tell you what to do. How to build a simple Calculator app using Android Studio? Small modifications are required if you copy and paste them into your activity class.
Here is the example: Templates let you quickly answer FAQs or store snippets for re-use. generate link and share the link here. Now, in this section, we will set up the data layer. The system may call onCreate() several times throughout the life of an activity, such as when a device screen is rotated. In truth, we could get more specific about small differences even within these two approaches. It should load the data into the UI. Below is the code for the MainActivity.ktfile. Please use ide.geeksforgeeks.org, Accordingly, we need to create our data class.
As you have probably noticed, we are probably not going to be re-using this ViewModel anywhere else. We will cover the following in this tutorial: MVVM architecture is a Model-View-ViewModel architecture that removes the tight coupling between each component. The first step in this exercise is to create the new project. Especially not data. Finally, the chapter showed how the ViewModel approach avoids some of the problems of handling Fragment and Activity lifecycles. Ultimately, I think the two elements which make for a great architecture come down to the following considerations: Firstly, play with several approaches until you find one which you prefer. The code to do this can be added to the onActivityCreated() method of the MainFragment.java file as follows: With the listener added, any code placed within the onClick() method will be called whenever the button is clicked by the user. Unfortunately, this property is only able to call methods on an Activity and cannot be used to call a method in a Fragment. When a project is created using the Fragment + ViewModel template, the structure of the project differs in a number of ways from the Basic Activity used when the AndroidSample project was created. The layout resource file for this fragment can be found at app -> res -> layout -> main_fragment.xml and will appear as shown in Figure 32-3 when loaded into the layout editor: By default, the fragment simply contains a TextView displaying text which reads MainFragment but is otherwise ready to be modified to contain the layout of the first app screen. You can make a tax-deductible donation here. This chapter will implement the same currency converter app, this time using the ViewModel component and following the Google app architecture guidelines to avoid Activity lifecycle complications. For further actions, you may consider blocking this person and/or reporting abuse.
Name: MVVM-Architecture-Android-Beginners, Package name: com.mindorks.framework.mvvm. Since LiveData is not yet being used in the project, it will also be necessary to get the latest result value from the ViewModel each time the Fragment is created. The dollar string value is converted to a floating point number, multiplied by a fictitious exchange rate and the resulting euro value stored in the result variable. However, another elegant way is to use by viewModels or by activityViewModels. My personal opinion is that it is worth it to apply separation of concerns to a very high degree. This method inflates the main_fragment.xml layout file so that it is displayed within the container area of the main activity layout: The ViewModel for the activity is contained within the MainViewModel.java class file located at app -> java -> ui.main -> MainViewModel. So this is just for your reference and knowledge. Begin by launching Android Studio and, if necessary, closing any currently open projects using the File -> Close Project menu option so that the Welcome screen appears. Our package in the project will look like below: We need the enum to represent the UI State. Then, create an interface ApiService inside the api package and add the following code. You want ViewModel to survive through activity or fragment destruction. It can't be used to replace by activityViewModels because the created ViewModelwon't be shared across different fragments. Android architecture components are the components that are used to build robust, clean, and scalable apps. You can also checkout Android Studio project code from our, 7. You can replace by viewModels with by ActivityViewModels if you want your ViewModel to survive in different fragments within the same activity. With that being said, one cannot simply add a reference back in to the ViewModel in order to regain this fine-grained control over the View. This content will typically take the form of a Fragment consisting of an XML layout resource file and corresponding class file. When called, the setAmount() method takes as an argument the current dollar amount and stores it in the local dollarText variable. There are drawbacks being discussed over the internet, but I do not fully understand this part yet. Purchase the fully updated Android Studio Chipmunk Edition of this publication in eBook ($29.99) or Print ($46.99) format, Android Studio Chipmunk Essentials - Java Edition Print and eBook (PDF) editions contain 94 chapters and over 800 pages. Instead, I will discuss two different approaches to the general idea of MVVM which present very distinct benefits and disadvantages. Once unpublished, all posts by vtsen will become hidden and only accessible to themselves. Associating the Fragment with the View Model, Creating an Example Java Android App in Android Studio, https://www.techotopia.com/index.php?title=An_Android_Jetpack_ViewModel_Tutorial&oldid=34029, Modern Android App Architecture with Jetpack. Some of us do not like filling View classes with Logic. You need to have a custom ViewModel factory to create your ViewModel.
In fact, when the project was created, Android Studio created an initial fragment for this very purpose. We are adding these two dependencies because to avoid using findViewById() in our MainActivity.kt file. Remaining in the MainFragment.java file, implement these requirements as follows in the onActivityCreated() method: With this phase of the project development completed, build and run the app on the simulator or a physical device, enter a dollar value and click on the Convert button. The version is just an example, you can use later or latest version. We accomplish this by creating thousands of videos, articles, and interactive coding lessons - all freely available to the public. Now, create a Kotlin file User inside the model package. Refer to this article: How to Create Classes in Android Studio? It essentially does the same thing as by lazy without the need to specify the ViewModelProvider. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. Sometimes you will run in to situations where you must find some kind of half-measure between the distribution of presentation logic between Views and ViewModels, which does not strictly follow either of these approaches. It means the data will be lost if it has been not saved and restored properly from the activity which was destroyed. Convert View Model to Use Hilt Dependency Injection, My Most Used Android Studio Shortcut Keys, Free and Useful Tools for Android Development and Blogging. Made with love and Ruby on Rails. These are my common practices. An activity must extend the ViewModel class to create a view model: class MainActivityViewModel : ViewModel() {. Now lets get into the code, Step 1: Add these dependencies in the build.gradle file, implementation androidx.lifecycle:lifecycle-viewmodel-ktx:$lifecycle_version, implementation androidx.lifecycle:lifecycle-livedata-ktx:$lifecycle_version, implementation androidx.lifecycle:lifecycle-runtime-ktx:$lifecycle_version, implementation androidx.core:core-ktx:1.3.2.

So all the data before the screen rotation is lost. On the form factors screen, enable the Phone and Tablet option and set the minimum SDK setting to API 26: Android 8.0 (Oreo) before proceeding to the Activity selection screen. Also, add the following dependency to the build.gradle(Module:app) file. For now, AndroidViewModel is good for me. Any kind of configuration change in Android devices tends to recreate the whole activity of the application.
This page was last modified on 11 January 2019, at 18:27. This is all about the MVVM, now let's move to the implementation part of it. You are reading a sample chapter from the Android Studio 3.2 Edition of this book. I also use AndroidViewModel by default instead of ViewModel because I usually need to access string resources and system services from the Application context. We will create that in the utils package. Repeat this test now with the ViewModelDemo app and note that the current euro value is retained after the rotation. ), so I think it is time to look at some code: While this is a very simplified example, the point is that the only thing which this particular ViewModel exposes publicly (other than the handleEvent function), is a simple Note object: With this particular approach, the ViewModel is well and truly decoupled from not just a particular View, but also the details, and by extension, presentation logic of any particular View. Tweet a thanks, Learn to code for free. Talk is cheap, and I strongly advise you to try and learn these things in the code so that you do not need to rely on people like me to tell you what to do. How to build a simple Calculator app using Android Studio? Small modifications are required if you copy and paste them into your activity class.
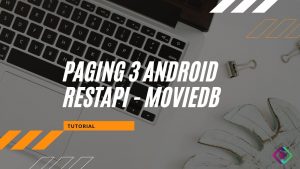
As you have probably noticed, we are probably not going to be re-using this ViewModel anywhere else. We will cover the following in this tutorial: MVVM architecture is a Model-View-ViewModel architecture that removes the tight coupling between each component. The first step in this exercise is to create the new project. Especially not data. Finally, the chapter showed how the ViewModel approach avoids some of the problems of handling Fragment and Activity lifecycles. Ultimately, I think the two elements which make for a great architecture come down to the following considerations: Firstly, play with several approaches until you find one which you prefer. The code to do this can be added to the onActivityCreated() method of the MainFragment.java file as follows: With the listener added, any code placed within the onClick() method will be called whenever the button is clicked by the user. Unfortunately, this property is only able to call methods on an Activity and cannot be used to call a method in a Fragment. When a project is created using the Fragment + ViewModel template, the structure of the project differs in a number of ways from the Basic Activity used when the AndroidSample project was created. The layout resource file for this fragment can be found at app -> res -> layout -> main_fragment.xml and will appear as shown in Figure 32-3 when loaded into the layout editor: By default, the fragment simply contains a TextView displaying text which reads MainFragment but is otherwise ready to be modified to contain the layout of the first app screen. You can make a tax-deductible donation here. This chapter will implement the same currency converter app, this time using the ViewModel component and following the Google app architecture guidelines to avoid Activity lifecycle complications. For further actions, you may consider blocking this person and/or reporting abuse.
Name: MVVM-Architecture-Android-Beginners, Package name: com.mindorks.framework.mvvm. Since LiveData is not yet being used in the project, it will also be necessary to get the latest result value from the ViewModel each time the Fragment is created. The dollar string value is converted to a floating point number, multiplied by a fictitious exchange rate and the resulting euro value stored in the result variable. However, another elegant way is to use by viewModels or by activityViewModels. My personal opinion is that it is worth it to apply separation of concerns to a very high degree. This method inflates the main_fragment.xml layout file so that it is displayed within the container area of the main activity layout: The ViewModel for the activity is contained within the MainViewModel.java class file located at app -> java -> ui.main -> MainViewModel. So this is just for your reference and knowledge. Begin by launching Android Studio and, if necessary, closing any currently open projects using the File -> Close Project menu option so that the Welcome screen appears. Our package in the project will look like below: We need the enum to represent the UI State. Then, create an interface ApiService inside the api package and add the following code. You want ViewModel to survive through activity or fragment destruction. It can't be used to replace by activityViewModels because the created ViewModelwon't be shared across different fragments. Android architecture components are the components that are used to build robust, clean, and scalable apps. You can also checkout Android Studio project code from our, 7. You can replace by viewModels with by ActivityViewModels if you want your ViewModel to survive in different fragments within the same activity. With that being said, one cannot simply add a reference back in to the ViewModel in order to regain this fine-grained control over the View. This content will typically take the form of a Fragment consisting of an XML layout resource file and corresponding class file. When called, the setAmount() method takes as an argument the current dollar amount and stores it in the local dollarText variable. There are drawbacks being discussed over the internet, but I do not fully understand this part yet. Purchase the fully updated Android Studio Chipmunk Edition of this publication in eBook ($29.99) or Print ($46.99) format, Android Studio Chipmunk Essentials - Java Edition Print and eBook (PDF) editions contain 94 chapters and over 800 pages. Instead, I will discuss two different approaches to the general idea of MVVM which present very distinct benefits and disadvantages. Once unpublished, all posts by vtsen will become hidden and only accessible to themselves. Associating the Fragment with the View Model, Creating an Example Java Android App in Android Studio, https://www.techotopia.com/index.php?title=An_Android_Jetpack_ViewModel_Tutorial&oldid=34029, Modern Android App Architecture with Jetpack. Some of us do not like filling View classes with Logic. You need to have a custom ViewModel factory to create your ViewModel.
In fact, when the project was created, Android Studio created an initial fragment for this very purpose. We are adding these two dependencies because to avoid using findViewById() in our MainActivity.kt file. Remaining in the MainFragment.java file, implement these requirements as follows in the onActivityCreated() method: With this phase of the project development completed, build and run the app on the simulator or a physical device, enter a dollar value and click on the Convert button. The version is just an example, you can use later or latest version. We accomplish this by creating thousands of videos, articles, and interactive coding lessons - all freely available to the public. Now, create a Kotlin file User inside the model package. Refer to this article: How to Create Classes in Android Studio? It essentially does the same thing as by lazy without the need to specify the ViewModelProvider. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. Sometimes you will run in to situations where you must find some kind of half-measure between the distribution of presentation logic between Views and ViewModels, which does not strictly follow either of these approaches. It means the data will be lost if it has been not saved and restored properly from the activity which was destroyed. Convert View Model to Use Hilt Dependency Injection, My Most Used Android Studio Shortcut Keys, Free and Useful Tools for Android Development and Blogging. Made with love and Ruby on Rails. These are my common practices. An activity must extend the ViewModel class to create a view model: class MainActivityViewModel : ViewModel() {. Now lets get into the code, Step 1: Add these dependencies in the build.gradle file, implementation androidx.lifecycle:lifecycle-viewmodel-ktx:$lifecycle_version, implementation androidx.lifecycle:lifecycle-livedata-ktx:$lifecycle_version, implementation androidx.lifecycle:lifecycle-runtime-ktx:$lifecycle_version, implementation androidx.core:core-ktx:1.3.2.