The development process of an Angular application is not different from other traditional web apps. The same value can be used in the HTML template using the following interpolation syntax. Get Placed at Top Product Companies with Scaler. Before starting a new project, Node.js must be installed on your machine. Angular CLI or the command-line interface is a very powerful and sophisticated tool that allows you to perform a lot of actions in an Angular project by using simple commands. Keep in mind that the sendFormData() method needs to be a member function inside the TypeScript class of the component otherwise you will run into errors. They work very well in combination with the Angular Router. Building a Web App with Angular and Bootstrap, Creating a Desktop App with Electron and Angular, Build a full-stack web application with Angular 7 and Nest.js. They are used to increase the reusability and modularity of apps. The Angular Router takes care of loading and unloading components in the placeholder RouterOutlet. You can follow this angular cheat sheet to build your application. Of course, this is just a very concise article so it cannot cover every single aspect of Angular. Secondly, we must tell Angular where to inject this class. Another structural directive is used to render HTML elements on the page based on an array or iterable. Angular CLI is the official utility for initializing and working with Angular projects. Services are not standalone.
If the guard returns true for the user, the route access if grant, else not. We can invoke this compoent using the selector as follows: Generally, a component's role is enabling the user experience. Interpolation is a way to use the properties in the components TypeScript class within the HTML template. Most commonly in components. Our entire codebase is formatted using this command. The text between the braces is often the name of a component property. We can create custom directives with the following command: To identify directives, classes are decorated with the @Directive() decorator.
Each component can contain more components which essentially makes an Angular app a tree of components. Angular apps have at least one NgModule class, which is referred to asthe root module. Here is an example. Prints the version info of the currently installed CLI. Starting from the Angular 9 release, the new compiler and runtime instructions are used by default instead of the older compiler and runtime, known as View Engine. Following are dependency injection configuration as part of Angulars DI framework: An element or component can be assigned an attribute directive or a structural directive to modify its behaviour. They're handy for reusing code in multiple components. For example: In this document, weve covered the basics of Angular, its features and some of the important cheat sheets. Ltd. is a Registered Education Ally (REA) of Scrum Alliance. Interpolation can also be used to concatenate strings, or perform simple mathematical operations right within the template. The following command is another simple example.
Angular components have a lifecycle that is administered by Angular. https://www.interviewbit.com/javascript-interview-questions/, https://www.interviewbit.com/angular-interview-questions/, https://www.interviewbit.com/angularjs-interview-questions/, https://www.interviewbit.com/blog/angular-architecture/, https://www.interviewbit.com/angular-8-interview-questions/, https://www.interviewbit.com/angular-mcq/, java interview questions for 5 years experience. Angular allows us to hook into these events by defining a set of methods in a component's class. What kind of decorator makes a class a service? We define the structure of the form using a form builder and then bind each form element to a form control. There are three directives at our disposal: We're not limited to directives defined by Angular. Most of the time, a class depends on other classes, rather than on itself, to create the required dependencies. JSON format is required to build model in AngularJS framework, Take popular mock tests for free with real life interview questions from top tech companies, Pair up with a peer like you and practise with hand-picked questions, Improve your coding skills with our resources, Compete in popular contests with top coders, Assess yourself and prepare for interviews, Take this "Angular Cheat Sheet" interview guide with you, By creating an account, I acknowledge that I have read and agree to InterviewBits. In 2010, AngularJS was the initial release and was known as AngularJS. The evaluated result is rendered on the page. Classes decorated with the @NgModule() decorator can register components, services, directives, and pipes. Security certifications & compliance. Structural Directives: Elements that are added or removed from the DOM in Angular's structure are referred to as structural directives. Please enjoy and if you'd like to submit any suggestions, feel free to email us at support@zerotomastery.io. We make sure your data is safe and secure. It comes in two types: The ng-for directive, which loops through a ul> or ol> element; and the ng-for-each directive, which iterates through a collection. We've tried to cover Angular CLI, Angular Lifecycle Hooks, Angular Routing, and a lot more in this post. Here are the most common structural directives in Angular: A directive that will conditionally create or remove elements from the template. Lets have a look at how we can allow a component to receive an input. So inputs and outputs are used to implement parent-child interaction within components. In the above snippet, the title property is bound to a variable called company_name in the template. Now, the component can accept an input called username as shown below.
A component controls a part of the application screen. It is performed only once after input values are set. Didnt receive confirmation instructions? Dependencies are created by external sources, such as services and other classes. More than memorizing syntax, do pay attention to practising them and solving problems. This approach is different from template-driven forms where we usengModeldirective to bind input fields to corresponding model properties.
Most HTML elements have events that they emit when certain conditions are met. A component has a life-cycle that begins from the moment Angular instantiates it, to when it's rendered and inserted into the DOM. Declares the class as a pipe and provides metadata about the pipe. This command will correct any form of linting errors. Here is an example implementation of the function, canActivate. A component is made up of an HTML template, a typescript class and a stylesheet file. Here is how you would define a simple form with two input fields, one for the email and another one for the password. Since modern browsers do not understand TypeScript, a TypeScript compiler or transpiler is required to convert the TypeScript code to regular JavaScript code. Lets have a look at some of the most common ways we use the Angular CLI. Unsubscribe at any time.
But actually, this continues with watching for any changes during the life of the component using change detection mechanisms. A dependency is a piece of information needed by a class to carry out its task. Data can be initialized in a component by calling this hook after input values are set. This option allows a service to be injectable in a single component class. The Angular CLI allows you to build the app. Too many versions, right? It is also executed when the input properties of the component change. Secondly, we can bind the [(ngModel)] directive on an HTML
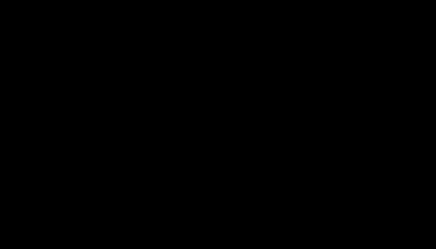
Each component can contain more components which essentially makes an Angular app a tree of components. Angular apps have at least one NgModule class, which is referred to asthe root module. Here is an example. Prints the version info of the currently installed CLI. Starting from the Angular 9 release, the new compiler and runtime instructions are used by default instead of the older compiler and runtime, known as View Engine. Following are dependency injection configuration as part of Angulars DI framework: An element or component can be assigned an attribute directive or a structural directive to modify its behaviour. They're handy for reusing code in multiple components. For example: In this document, weve covered the basics of Angular, its features and some of the important cheat sheets. Ltd. is a Registered Education Ally (REA) of Scrum Alliance. Interpolation can also be used to concatenate strings, or perform simple mathematical operations right within the template. The following command is another simple example.
Angular components have a lifecycle that is administered by Angular. https://www.interviewbit.com/javascript-interview-questions/, https://www.interviewbit.com/angular-interview-questions/, https://www.interviewbit.com/angularjs-interview-questions/, https://www.interviewbit.com/blog/angular-architecture/, https://www.interviewbit.com/angular-8-interview-questions/, https://www.interviewbit.com/angular-mcq/, java interview questions for 5 years experience. Angular allows us to hook into these events by defining a set of methods in a component's class. What kind of decorator makes a class a service? We define the structure of the form using a form builder and then bind each form element to a form control. There are three directives at our disposal: We're not limited to directives defined by Angular. Most of the time, a class depends on other classes, rather than on itself, to create the required dependencies. JSON format is required to build model in AngularJS framework, Take popular mock tests for free with real life interview questions from top tech companies, Pair up with a peer like you and practise with hand-picked questions, Improve your coding skills with our resources, Compete in popular contests with top coders, Assess yourself and prepare for interviews, Take this "Angular Cheat Sheet" interview guide with you, By creating an account, I acknowledge that I have read and agree to InterviewBits. In 2010, AngularJS was the initial release and was known as AngularJS. The evaluated result is rendered on the page. Classes decorated with the @NgModule() decorator can register components, services, directives, and pipes. Security certifications & compliance. Structural Directives: Elements that are added or removed from the DOM in Angular's structure are referred to as structural directives. Please enjoy and if you'd like to submit any suggestions, feel free to email us at support@zerotomastery.io. We make sure your data is safe and secure. It comes in two types: The ng-for directive, which loops through a ul> or ol> element; and the ng-for-each directive, which iterates through a collection. We've tried to cover Angular CLI, Angular Lifecycle Hooks, Angular Routing, and a lot more in this post. Here are the most common structural directives in Angular: A directive that will conditionally create or remove elements from the template. Lets have a look at how we can allow a component to receive an input. So inputs and outputs are used to implement parent-child interaction within components. In the above snippet, the title property is bound to a variable called company_name in the template. Now, the component can accept an input called username as shown below.
A component controls a part of the application screen. It is performed only once after input values are set. Didnt receive confirmation instructions? Dependencies are created by external sources, such as services and other classes. More than memorizing syntax, do pay attention to practising them and solving problems. This approach is different from template-driven forms where we usengModeldirective to bind input fields to corresponding model properties.
Most HTML elements have events that they emit when certain conditions are met. A component has a life-cycle that begins from the moment Angular instantiates it, to when it's rendered and inserted into the DOM. Declares the class as a pipe and provides metadata about the pipe. This command will correct any form of linting errors. Here is an example implementation of the function, canActivate. A component is made up of an HTML template, a typescript class and a stylesheet file. Here is how you would define a simple form with two input fields, one for the email and another one for the password. Since modern browsers do not understand TypeScript, a TypeScript compiler or transpiler is required to convert the TypeScript code to regular JavaScript code. Lets have a look at some of the most common ways we use the Angular CLI. Unsubscribe at any time.
But actually, this continues with watching for any changes during the life of the component using change detection mechanisms. A dependency is a piece of information needed by a class to carry out its task. Data can be initialized in a component by calling this hook after input values are set. This option allows a service to be injectable in a single component class. The Angular CLI allows you to build the app. Too many versions, right? It is also executed when the input properties of the component change. Secondly, we can bind the [(ngModel)] directive on an HTML