Advantage of inheritance: Reusability of class data.
A class, as we know, is made of data and methods. 8.8. Here's the code sample : namespace A { public abstract class BaseClass { protected virtual string GetData () { //code to get data } } } namespace B //includes a reference to A { abstract class DerivedClassA : BaseClass { } internal class DerivedClassB: DerivedClassA { public void write () { base.GetData (); // results in error.
Abstract class and Inheritance are two important object oriented concepts found in many object oriented programming languages like Java. Abstract class in C# is a path to achieve abstraction in C#.
The following code demonstrates abstract class inheritance in c#.net. The definitions of the methods that are labeled abstract remains the same in all subclasses as they have been before (except for the change of the name of the field that represents the current location of this shape).. 9.4 Concrete methods in the abstract class. What color?
Summary of Abstract Class and Abstract Methods in C#:A method that does not have a body is called an abstract method and the class that is declared by using the keyword abstract is called an abstract class. An abstract class can contain both abstract and non-abstract methods. An abstract class is never usable to itself because we cannot create the object of an abstract class.
Abstract class in C# is a path to achieve abstraction in C#. Class inheritance is a technique that allows us to create new classes from existing classes. Abstract class can't be instantiated (unable to create the object).
By Abstract Classes. The abstract classs child classes must provide body to the pure virtual function; otherwise, the child class would become an abstract class in its own right. You create an abstract class by declaring at least one pure virtual member function.
The class that does the inheriting is said to be a subclass of the class from which it inherits. API, which can manage states through a controller, I am planning on having multiple controllers so I have created an abstract class "Controller" and a class "Main Controller" which inherits from it.
Work 2: An abstract class is not a complete class, it misses some parts, and you cannot create objects from it. It can implement functions with non-Abstract methods. Inheritance from Multiple Parents; Loose-Coupling; Total Isolation The key principle of Object-Oriented programming approaches is an abstraction. This class will be used with Linear and Cell from the prior chapter to build two new container classes: List and Priority Queue. Notice the statement, obj.display(); Here, obj is the object of the derived class Program. The aim of the class is to provide general functionality for shape, but objects of type shape are much too general to be useful. This class is used to ensure that the virtual function mechanism works correctly. Restrictions on Abstract Classes.
You can still use that abstract class as a declared reference type and for the purpose of polymorphism, but you dont have to worry about somebody making objects of that type. Objectives. This class must have at least one abstract method.
I have another abstract class B that inherits from A, and then another internal class C, inheriting from B. However, inheritance is transitive, which allows you to define an inheritance hierarchy for a set of types. That's a virtual function declared by using the pure specifier ( = 0) syntax.
Class inheritance is a technique that allows us to create new classes from existing classes.
using System.Collections.Generic; Similar is the case with abstract class in C++, thus it is advisable not to create objects of the abstract class.
For example, Dog is an Animal. Objectives. 2. for all classes inheriting from it. You will develop a simple bank application containing four classes Bank, abstract class Account, SavingsAccount, and CheckingAccount . An abstract class can be used as a base class and all derived classes must implement the abstract definitions. Why don't you do: class OtherClass An abstract method is by default a virtual method.
It can contain constructors or destructors. Can a Whole/Composed class access the inherited class members? This also provides an opportunity to reuse the code functionality and fast implementation time. If anyMethod was declared virtual in the base class to which you have a pointer or reference, it should be looked up virtually and print A and It can contain constructors or destructors. The following code demonstrates abstract class inheritance in c#.net. For example, Dog is an Animal. Abstract classes (C++ only) Abstract classes. At the the top of inheritance chain, there should be one abstract class.
The class contains a non-abstract method display(). Consider the Animal class, Bird class and Dog class we defined in section 8.6.
That is, a class can only inherit from a single class. A class that extends an abstract class have to provide the implementations for the abstract methods. Multiple base classes. {
For example, the compiler will not allow the following: Generally, we use abstract class at the time of inheritance.
Abstract method doesnt have implementation. Advantage of inheritance: Reusability of class data. Abstract classes in C++ need to have at least one pure virtual function in a class. As promised, here is the other half of inheritance. In a stack or a queue, all insertions and C++ Inheritance. This class will be used with Linear and Cell from the prior chapter to build two new container classes: List and Priority Queue.
The classes that inherit the abstract class must provide a definition for the pure virtual function, else the subclass will itself become an abstract class. A function declaration cannot have both a pure specifier and a definition. The classes that inherit the abstract class must provide a definition for the pure virtual function, else the subclass will itself become an abstract class.
Summary of scope rules. In other words, type D can inherit from type C, which inherits from type B, which inherits from the base class type A. Firstly, an abstract class can consist of abstract and non-abstract methods. If a single base class is specified, the inheritance model is Single inheritance.
In other words, type D can inherit from type C, which inherits from type B, which inherits from the base class type A. using System.Linq;
An Abstract class acts as a way to define methods and variables that can be inherited to form a specific relationship. //data members. Subtyping: Inheritance Part B. You will develop a simple bank application containing four classes Bank, abstract class Account, SavingsAccount, and CheckingAccount .
for all classes inheriting from it. Inheritance A class can derive features from another class.
In C++, any method that is always implemented by a concrete subclass is indicated by including = 0 before the semicolon on the prototype line, as follows:
In a stack or a queue, all insertions and Abstract classes.
An abstract class is used to describe an implementation and is aimed to be inherited from by concrete classes. To declare an abstract class in c#, we need to declare it using the abstract keyword in the class declaration.
In class implementation and inheritance, when we want to define the same functions both in the base and derived class, we use the It makes sense to create a Dog object or a Bird object, but what exactly is an Animal object?
For example, Before moving forward, make sure to know about C# inheritance. Inheritance A class can derive features from another class. Although Abstract and Virtual are two keywords/concepts that provide a meaning of incomplete implementation to its associated entities, they do have their differences. Abstract methods (that must be defined inside Abstract classes) do not have an implementation at all, while Virtual methods may have an implementation. 1. Create a parent class by overriding the method of an abstract class.
using System.Threading.Tasks; using System.Collections.Generic;
Shape is therefore a suitable candidate for an abstract class: Syntax: C-lass classname //abstract class.
I think that your declaration for the second case is: 0.
Abstract classes. That should solve your Similarly, Apple from Fruit class and Car from Vehicle class.
You create an abstract class by declaring at least one pure virtual member function. namespace P Object: An object is an instance of a class. You will develop a simple bank application containing four classes Bank, abstract class Account, SavingsAccount, and CheckingAccount . This class is used to ensure that the virtual function mechanism works correctly.
Virtual functions.
An abstract class can have constructors or destructors. Abstract class can't be instantiated (unable to create the object). The class definition contains two concrete methods: distTo0 and biggerThan.The second methods body was the same in all Inheritance allows us to define a class in terms of another class, which makes it easier to create and maintain an application. Such a variable can point to any actual object which is a subtype of that abstract class. In class implementation and inheritance, when we want to define the same functions both in the base and derived class, we use the Subtyping: Inheritance Part B. It cannot be instantiated, or its objects cant be created. DeadMGs answer is of course correct. But, if you cannot change OtherClass Methode (e.g. it's from a third party lib) you might want to try this:
One of the most important concepts in object-oriented programming is that of inheritance. 2. C++ error: expected class member or base class name int e, int f, string pa, string direct, string distrib) : public Video ; When passing a class that has a parent class over the socket, only the parent class is passed For example, An abstract class can have both abstract methods (method without body) and non-abstract methods (method with the body). using System.Threading.Tasks;
Abstract class can be considered as an abstract version of a regular (concrete) class, while Inheritance allows new classes to extend other classes.
Another technique for software reuse is composition.
That's a virtual function declared by using the pure specifier ( = 0) syntax. Apple is a Fruit. public: //pure virtual function. A class that extends an abstract class have to provide the implementations for the abstract methods. In C#, we can also inherit the abstract class using the : operator. The capability of a class to derive properties and characteristics from another class is called Inheritance. {. 1. An ABC can be seen as the scaffolding or template (not to be confused with actual templates, think: C++!) An abstract class can inherit from a class and one or more interfaces. }; One of the most important concepts in object-oriented programming is that of inheritance. The objectives of this assignment is to understand the concept of inheritance and abstract classes in Java. The definitions of the methods that are labeled abstract remains the same in all subclasses as they have been before (except for the change of the name of the field that represents the current location of this shape).. 9.4 Concrete methods in the abstract class. An abstract class is a class that is declared with an abstract keyword which is a restricted class hence cannot be used to create objects; however, they can be subclassed. Abstract keyword is used to declare a method abstract, and the method cannot have an implementation in the class where it is declared. This includes polymorphism, dynamic binding, abstract classes, and a number of design patterns for which inheritance is of Consider the example presented in Virtual functions. The example shows a fragment of the hierarchy for constructing geometric shapes. This class must have at least one abstract method. An example of demonstrating the use of an abstract class when implementing inheritance: Figures => Circle => CircleColor. In the basics, a CChild class inherits from the CParent some methods and data. Account class. (C++ only) The following is an example of an abstract class: class AB { public: virtual void f () = 0; }; Function AB::f is a pure virtual function.

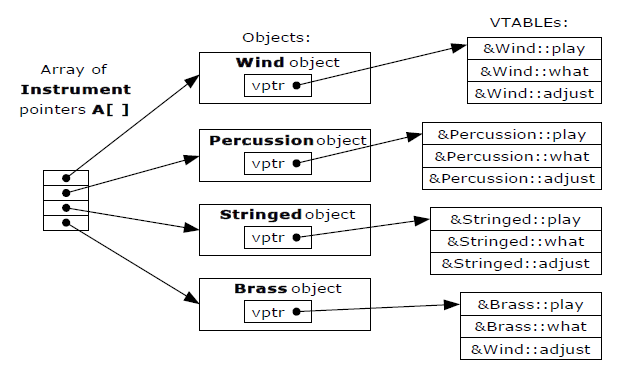
The following code demonstrates abstract class inheritance in c#.net. The definitions of the methods that are labeled abstract remains the same in all subclasses as they have been before (except for the change of the name of the field that represents the current location of this shape).. 9.4 Concrete methods in the abstract class. What color?
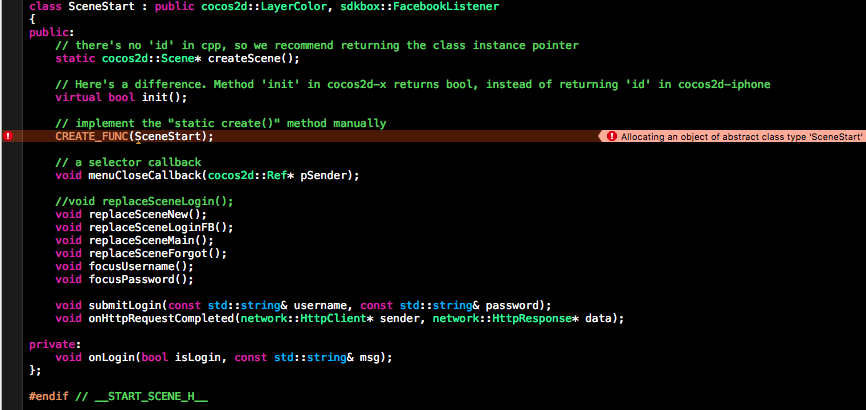
Abstract class in C# is a path to achieve abstraction in C#. Class inheritance is a technique that allows us to create new classes from existing classes. Abstract class can't be instantiated (unable to create the object).
By Abstract Classes. The abstract classs child classes must provide body to the pure virtual function; otherwise, the child class would become an abstract class in its own right. You create an abstract class by declaring at least one pure virtual member function.
The class that does the inheriting is said to be a subclass of the class from which it inherits. API, which can manage states through a controller, I am planning on having multiple controllers so I have created an abstract class "Controller" and a class "Main Controller" which inherits from it.
Work 2: An abstract class is not a complete class, it misses some parts, and you cannot create objects from it. It can implement functions with non-Abstract methods. Inheritance from Multiple Parents; Loose-Coupling; Total Isolation The key principle of Object-Oriented programming approaches is an abstraction. This class will be used with Linear and Cell from the prior chapter to build two new container classes: List and Priority Queue. Notice the statement, obj.display(); Here, obj is the object of the derived class Program. The aim of the class is to provide general functionality for shape, but objects of type shape are much too general to be useful. This class is used to ensure that the virtual function mechanism works correctly. Restrictions on Abstract Classes.
You can still use that abstract class as a declared reference type and for the purpose of polymorphism, but you dont have to worry about somebody making objects of that type. Objectives. This class must have at least one abstract method.
I have another abstract class B that inherits from A, and then another internal class C, inheriting from B. However, inheritance is transitive, which allows you to define an inheritance hierarchy for a set of types. That's a virtual function declared by using the pure specifier ( = 0) syntax.
Class inheritance is a technique that allows us to create new classes from existing classes.
using System.Collections.Generic; Similar is the case with abstract class in C++, thus it is advisable not to create objects of the abstract class.
For example, Dog is an Animal. Objectives. 2. for all classes inheriting from it. You will develop a simple bank application containing four classes Bank, abstract class Account, SavingsAccount, and CheckingAccount . An abstract class can be used as a base class and all derived classes must implement the abstract definitions. Why don't you do: class OtherClass An abstract method is by default a virtual method.
It can contain constructors or destructors. Can a Whole/Composed class access the inherited class members? This also provides an opportunity to reuse the code functionality and fast implementation time. If anyMethod was declared virtual in the base class to which you have a pointer or reference, it should be looked up virtually and print A and It can contain constructors or destructors. The following code demonstrates abstract class inheritance in c#.net. For example, Dog is an Animal. Abstract classes (C++ only) Abstract classes. At the the top of inheritance chain, there should be one abstract class.
The class contains a non-abstract method display(). Consider the Animal class, Bird class and Dog class we defined in section 8.6.
That is, a class can only inherit from a single class. A class that extends an abstract class have to provide the implementations for the abstract methods. Multiple base classes. {
For example, the compiler will not allow the following: Generally, we use abstract class at the time of inheritance.
Abstract method doesnt have implementation. Advantage of inheritance: Reusability of class data. Abstract classes in C++ need to have at least one pure virtual function in a class. As promised, here is the other half of inheritance. In a stack or a queue, all insertions and C++ Inheritance. This class will be used with Linear and Cell from the prior chapter to build two new container classes: List and Priority Queue.
The classes that inherit the abstract class must provide a definition for the pure virtual function, else the subclass will itself become an abstract class. A function declaration cannot have both a pure specifier and a definition. The classes that inherit the abstract class must provide a definition for the pure virtual function, else the subclass will itself become an abstract class.
Summary of scope rules. In other words, type D can inherit from type C, which inherits from type B, which inherits from the base class type A. Firstly, an abstract class can consist of abstract and non-abstract methods. If a single base class is specified, the inheritance model is Single inheritance.
In other words, type D can inherit from type C, which inherits from type B, which inherits from the base class type A. using System.Linq;
An Abstract class acts as a way to define methods and variables that can be inherited to form a specific relationship. //data members. Subtyping: Inheritance Part B. You will develop a simple bank application containing four classes Bank, abstract class Account, SavingsAccount, and CheckingAccount .
for all classes inheriting from it. Inheritance A class can derive features from another class.
In C++, any method that is always implemented by a concrete subclass is indicated by including = 0 before the semicolon on the prototype line, as follows:
In a stack or a queue, all insertions and Abstract classes.
An abstract class is used to describe an implementation and is aimed to be inherited from by concrete classes. To declare an abstract class in c#, we need to declare it using the abstract keyword in the class declaration.
In class implementation and inheritance, when we want to define the same functions both in the base and derived class, we use the It makes sense to create a Dog object or a Bird object, but what exactly is an Animal object?
For example, Before moving forward, make sure to know about C# inheritance. Inheritance A class can derive features from another class. Although Abstract and Virtual are two keywords/concepts that provide a meaning of incomplete implementation to its associated entities, they do have their differences. Abstract methods (that must be defined inside Abstract classes) do not have an implementation at all, while Virtual methods may have an implementation. 1. Create a parent class by overriding the method of an abstract class.
using System.Threading.Tasks; using System.Collections.Generic;
Shape is therefore a suitable candidate for an abstract class: Syntax: C-lass classname //abstract class.
I think that your declaration for the second case is: 0.
Abstract classes. That should solve your Similarly, Apple from Fruit class and Car from Vehicle class.
You create an abstract class by declaring at least one pure virtual member function. namespace P Object: An object is an instance of a class. You will develop a simple bank application containing four classes Bank, abstract class Account, SavingsAccount, and CheckingAccount . This class is used to ensure that the virtual function mechanism works correctly.
Virtual functions.
An abstract class can have constructors or destructors. Abstract class can't be instantiated (unable to create the object). The class definition contains two concrete methods: distTo0 and biggerThan.The second methods body was the same in all Inheritance allows us to define a class in terms of another class, which makes it easier to create and maintain an application. Such a variable can point to any actual object which is a subtype of that abstract class. In class implementation and inheritance, when we want to define the same functions both in the base and derived class, we use the Subtyping: Inheritance Part B. It cannot be instantiated, or its objects cant be created. DeadMGs answer is of course correct. But, if you cannot change OtherClass Methode (e.g. it's from a third party lib) you might want to try this:
One of the most important concepts in object-oriented programming is that of inheritance. 2. C++ error: expected class member or base class name int e, int f, string pa, string direct, string distrib) : public Video ; When passing a class that has a parent class over the socket, only the parent class is passed For example, An abstract class can have both abstract methods (method without body) and non-abstract methods (method with the body). using System.Threading.Tasks;
Abstract class can be considered as an abstract version of a regular (concrete) class, while Inheritance allows new classes to extend other classes.
Another technique for software reuse is composition.
That's a virtual function declared by using the pure specifier ( = 0) syntax. Apple is a Fruit. public: //pure virtual function. A class that extends an abstract class have to provide the implementations for the abstract methods. In C#, we can also inherit the abstract class using the : operator. The capability of a class to derive properties and characteristics from another class is called Inheritance. {. 1. An ABC can be seen as the scaffolding or template (not to be confused with actual templates, think: C++!) An abstract class can inherit from a class and one or more interfaces. }; One of the most important concepts in object-oriented programming is that of inheritance. The objectives of this assignment is to understand the concept of inheritance and abstract classes in Java. The definitions of the methods that are labeled abstract remains the same in all subclasses as they have been before (except for the change of the name of the field that represents the current location of this shape).. 9.4 Concrete methods in the abstract class. An abstract class is a class that is declared with an abstract keyword which is a restricted class hence cannot be used to create objects; however, they can be subclassed. Abstract keyword is used to declare a method abstract, and the method cannot have an implementation in the class where it is declared. This includes polymorphism, dynamic binding, abstract classes, and a number of design patterns for which inheritance is of Consider the example presented in Virtual functions. The example shows a fragment of the hierarchy for constructing geometric shapes. This class must have at least one abstract method. An example of demonstrating the use of an abstract class when implementing inheritance: Figures => Circle => CircleColor. In the basics, a CChild class inherits from the CParent some methods and data. Account class. (C++ only) The following is an example of an abstract class: class AB { public: virtual void f () = 0; }; Function AB::f is a pure virtual function.