prop.
Here is a simple program to get the file extension in Python.Check Data Type of Integer Variable. var1 = "Hello" var2 = 123 var3 = "123" # using isstance() res_var1 = isinstance(var1, str) res_var2 = isinstance(var2, str) res_var3 = isinstance(var3, str) # print result print("Is variable a string ? There is no try.' (Python recipe) Workaround for the SQLite limitation that prevents multiple threads from sharing a Connection object. Example 2: INPUT: [3,7,9,10,5] 8 OUTPUT:[0,4] Logic: A simple method is to use a two nested loop and generate all the pairs and check for their sum. A basic approach to do this check is to use a for loop that goes through every character of the string and checks if that character is a number by using the string isdigit() method.. Method #1 : Using isinstance (x, str) This method can be used to test whether any variable is a particular datatype. x = 'abcd' isinstance(x, (int, float)) returns.
Created: March-14, 2021 | Updated: March-21, 2021.
The option means that the type of data stored in the array is a variable. After iteration check whether the counter is set or not. In the above code, we have four values assigned to different variables.The isinstance() method will return True for value_1 only Examples: Input: 28 Output: digit Input: a Output: not a digit.Input: 21ab Output: not a digit. The code example below shows how we can use it. In the case of a string variable. In Approach 2: Simple Iteration. The most efficient way to check if a string is an integer in Python is to use the str.isdigit () method, as it takes the least time to execute. City Car Rush Traffic Challenge Race 64 Bit Source Code. Given a string, write a Python program to find whether a string contains only numbers or not. To check if the variable is an integer in Python, we will use isinstance() which will return a boolean value whether a variable is of type integer or not. After 3.5 are installed we have to open the command prompt window and type the "command prompt" in the search box and click on the start button.PIP is a tool for Python, and you cannot install PIP without Python.
Please show all steps beginning toIn C++, an array is a variable that can store multiple values of the same type. If it is not then set a counter and break. Yes it is string variable. When we say a number, it means that it can be an integer or a float. (ignored by fixed wing, time to stay at MISSION for rotary wing) | Acceptance radius in meters (if the Max int in Python 2. type (a) == int or isinstance (a, int). The first is your variable. The second is the type you want to check for.
Resx. Python: Add Variable to String & Print Using 4 Methods. For example, print( (10.0).is_integer()) print( (15.23).is_integer()) This will give the output.. Next: Add Python to PATH in User Variables. Python Example #2: Twitter API call. except ValueError: As you know, Pythons input() function always converts the user input to a string. The int datatype is used to represent integers. let's say that you want to test or check if a variable is a string, see the code bellow Data Types in Python str int float complex list tuple range dict bool bytes Related posts. To check if a python variable is a number (int or float par example), a solution is to use isinstance: x = 1.2 isinstance(x, (int, float)) returns here. How to create a string in Python + assign it to a variable in python; How to create a variable in python; Function in Python; Example: Iterate for every character and check if the character is 0 or 1. VariablesGeneral Concept of Variables. This subchapter is especially intended for C, C++ and Java programmers, because the way these programming languages treat basic data types is different from the way Variables in Python. There is no declaration of variables required in Python, which makes it quite easy. Object References. Use the int() Method to Check if an Object Is an int Type in Python Use the float.is_integer() Method to Check if an Object Is an int Type in Python In Python, we have different datatypes when we deal with numbers. Description. Uses the type built-in function to see if the type of your variable is str. The code example below shows how we can use it. True For a sum of '14', the check digit is '6' since '20' is the next number divisible by ten. Write a python program to check if the number given is a palindrome or not; Pattern questions. If we want to ensure that a variable stores a particular data type, we can use the isinstance() function. Write a Python program to check whether a variable is integer or string. cannot apply java lang integer android. conn = sqlite3.connect ('db.sqlite3') If you run the code above, it will create a database file in the folder you run it in.MultiThread support for SQLite access. Angry Zombies. Do you want an array of int?Then create a TArray variable. As you can see, The output shows the type of a variable as a string (str). In my opinion you have two options: Just try to convert it to an int , but catch the exception: try: To check if a variable contains a value that is a string, use the isinstance built-in function. Please show all steps beginning toIn C++, an array is a variable that can store multiple values of the same type. To check whether the string can be used as an integer or a decimal number, you have to write more code. Method :- xml ( text [,indent_pattern] ) This method takes two parameters as an argument :-. >>>isinstance('stuff',str) Let us understand some of the ways of checking for a string type object. The year of the movie will be the partition key and the title will be the sort key. Using isinstance () method. INPUT: [2,7,11,15] 9 OUTPUT:[0,1] As the sum of integers at 0 and 1 index(2 and 7) gives us a sum of 9. if you want to check what it is: >>>isinstance(1,str) the user input type is always a string. @SeakyLone: isdigit is the wrong test. Sample Solution:- Python Code : print(isinstance(25,int) or isinstance(25,str)) print(isinstance([25],int) or isinstance([25],str)) print(isinstance("25",int) or isinstance("25",str)) Sample Output: Add Items in Dictionary Variable in Python. Python VariablesCreating Variables. In Python, a variable does not need to be declared while creating or before adding a value to it. Get and Check Variable Type. The type () method is used to get the type of a created variable.Check if Variable Exist. The locals () usually used to check the existence of a local variable.
In this tutorial, we will discuss how to check if a variable is int or not. To check if a variable is a String in Python, use the type() function and compare the output of the type() function to the str class, and if it returns True, then a variable is String; otherwise not. On windows laptop:-. The type() is a built-in Python function that True b = 3 c = 3.4 print (isinstance (b,int)) print (isinstance (c,int)) Here is the screenshot of following given code. You use local variable in component template. Lets take an example to check if a variabe is an integer. Fri Feb 4 2022 by Luis Hctor Chvez. Code snippet for string indexer annotation . In our case, we are testing string type so the second parameter will be a string type. The isinstance function takes two arguments. Using a For Loop and isdigit() To Find Out if a String Contains Numbers. BytesParsing.sol convert java to c. android java convert boolean to string. How to insert .format multiple variables in Python. There is a max int limit in Python 2, and if you cross the max limit, it would automatically switch from int to long. It will "return true if all characters in the string are digits and there is at least one character, false otherwise: It will "return true if all characters in the string are digits and there is at least one character, false otherwise: After writing the above code (python check if the variable is an integer), Ones you will print isinstance() then the output will appear as The value is a string data type with a length of four characters. If the input string is a number, It will get converted to int or float without exception. After writing the above code (python check if the variable is an integer), Ones you will print isinstance() then the output will appear as Method #1: Using isdigit() method Please enter number or string or both test556 str does not contain a number Python check if given string contains only letter.
Check if a python variable is a number using isinstance. To check whether the string can be used as an integer or a decimal number, you have to write more code. Fa How to check if variable is integer in python. There is no try.' Check if Variable is a String with type() string = "'Do, or do not. requests.exceptions Examples. - Yoda" integer = 42 float = 3.14 # Print results print("The type of string is ", type(string)) print("The type of integer is ", type(number)) print("The type of float is ", type(float)) The Python function import_csv_to_dynamodb(table_name, csv_file_name, colunm_names, column_types) below imports a CSV file into a DynamoDB table. Specifically, conditionals perform different computations or actions depending on whether a programmer-defined boolean condition evaluates to true or false. There are two types of variable first one is local variable that is defined inside the function and the second one are global variable that is defined outside the function. For example, strings have the method .isdigit() that returns True if all the characters in the string are digits - this allows checking whether the string contains an integer. You would need to convert it to array (it depends if you use string or number enums). Python Sort Tuple Reverse Example 2. A Python string is used to set the name of the dimension, and an integer value is used to set the size. Related Programs: python program to check if a substring is present in a given string; check the first or last character of a string in python; pandas 4 ways to check if a dataframe is empty in python; python program to check if a string is palindrome or not; python program to check if a string is a pangram or not Using isinstance (var, str) The isinstance function takes two arguments. Convert string input to int or float to check if it is a number Code #1: Using Python regex re.search(): This method either returns None (if the pattern doesnt match) or a re.MatchObject contains information about the matching part of the string. In computer science, conditionals (that is, conditional statements, conditional expressions and conditional constructs,) are programming language commands for handling decisions. Print the result. 0. is int python isinstance(n, int) # n = 9, Returns True / n = 5.5, Returns False. isinstance (n, (str, int, float) #True. Code snippet for property . For example, strings have the method .isdigit() that returns True if all the characters in the string are digits - this allows checking whether the string contains an integer. False The declared types have some default values called Zero-State, for example for bool the default value is False. If at least one character is a digit then return True otherwise False. i.e. Please enter number or string or both 1234 str contains a number. Common types are: int, str, list, object, etc. To add Python to the PATH in User variables, right-click on This PC, and select Properties. Based on the data entered by the user, the data type of the var changes. How to check if a variable is a string. For example, x = 10 print (type (x)) print (type (x) == int) Output: True. Created: April-05, 2021 | Updated: July-18, 2021. Depending on your definition of shortly, you could use one of the following options: try: int(your_input); except ValueError: # your_input.isdi Python Basic: Exercise-144 with Solution. Using ascii values of characters, count and print all the digits using isdigit () function. By specifying the second argument as "str", we can check if the variable we are passing is a string or not. Note that if you are executing the following code in Python 2.x, you will have to declare the encoding as UTF-8/Unicode - as follows: [python] # -*- coding: utf-8 -*-. Variables in Python can be defined locally or globally. How to put variable in regex pattern in Python. We can also check float and string types using this method. The most efficient way to check if a string is an integer in Python is to use the str.isdigit () method, as it takes the least time to execute. In this tutorial, we will discuss how to check if a Please see dev Step #3: Create Request. To check if a variable is a Number in Python, use the type () function and compare its result with either int or float types to get the boolean value. nguid. x: This is any given variable. The isdigit method of the str type returns True iff the given string is nothing but one or more digits. If it's not, you know the string sh myVariable = 'A string' if type (myVariable) == int or float : print ( 'The variable a number' ) else : print ( 'The variable is not a number' ) This, regardless of the input, returns: The variable is a number. True. while. Do comment if you have any questions or suggestions on this Python var string topic. # The conn variable will be used to interact with the database. The second is the type you want to check for. Data Types in Python: Python has numerous standard data types that are used to define the operations possible on them and the storage method for each of them. We've improved the Python experience during last year, with a Python package cache to make installs faster, and an integrated, multiplayer debugger to increase the understanding of what programs do. To check if a variable contains a value that is a string, use the isinstance built-in function. 0. python check if int colors = [11, 34.1, 98.2, 43, 45.1, 54, 54] for x in colors: if int(x) == x: print(x) #or if isinstance(x, int): print(x) 6. Quickly convert HTML, CSS and Javascript into Javascript, Typescript, React, PHP, VBScript, ASP, Perl, Python, Ruby, Lisp and more with the Web Code Converter As its name suggests Download and extract java2python .
Method 2 using isinstance () function. 6. If you are using Python 2, you can find the maximum value of the integer using sys.maxint. In terms of control flow, the decision is The below code organize the elements in an integer tuple. Check if Variable is a String with type() string = "'Do, or do not. I n this tutorial, we are going to see how to check if a variable is a Number in Python. os.path.exists () method in Python is used to check whether the specified path exists or not. This method can be also used to check whether the given path refers to an open file descriptor or not. Attention geek! We can use isinstance() function to verify that a variable is string or not. Below code checks, if the variable n belongs to any of the str, int or float data type. Lets go through an example in which we will create two variables, one with the data type string and another with the data type of int.
This method can be used to check if a variable is specific data type. >>>isinstance(1,int)
count = 0. for char in string: To check if the variable is an integer in Python, we will use isinstance() which will return a boolean value whether a variable is of type integer or not. var = 1 if type(var) == int: print('your variable is an integer') or: var2 = 'this is variable #2' if type(var2) == str: print('your variable is a string') else: print('your variable IS NOT a string') hope this helps! Another Example. Explicitly test if there are only digits in the string: value.isdigit() str.isdigit() returns True only if In using this value, I noticed multiplying 4.56 by 100 returns 455.99999999999994 instead of 456. Python check if the variable is an integer. Example. The isinstance function takes two arguments. ctx. How to check if a variable is a string. This is the Ask Forgiveness approach. If it returns True, Check Python variable is of an integer type or not. Write a python program to check if the number given is a palindrome or not; Pattern questions. Just try to convert it to an int, but catch the exception: try: value = int(value) except ValueError: pass # it was a string, not an int. Solution: In such a situation, We need to convert user input explicitly to integer and float to check if its a number. : " + str(res_var2)) print("Is variable a string ? These are the int, float, and complex datatypes. If you want to add new items to the dictionary using Python. I was using the Python interpreter to test my workflow, and chose 4.56 as a random test value. Different ways to get the number of words in a string in Python. python to java code conversion. y: This here can be any data type that you want to check the variable for. An integer variable is a variable with a numeric value. Use the type() Function to Check if a Variable Is a String or Not ; Use the isinstance() Function to Check if a Variable Is a String or Not ; The string datatype is used to represent a collection of characters. You can also use the isinstance(val, type) method of python to check whether a value is an integer or not. The str.isdigit () method returns True if the string represents an integer, otherwise False. So the answer is '1 + 8 + 3 + 2 = 14' and the check digit is the amount needed to reach a number divisible by ten. Note: IDE: PyCharm 2021.3 (Community Edition) Windows 10. def check2 (string): t = '01'. !! Using numbers.Number () method. Next, we used the python function to sort them in ascending order.Introduction to Selection Sort in Python. Note that f-strings are not used in the example to preserve compatibility with versions below Python 3.6.Once you finish your discord bot, attach a simple web server into it. Use the int() Function to Check if the Input Is an Integer in Python ; Use the isnumeric() Method to Check if the Input Is an Integer or Not ; Use the Regular Expressions to Check if the Input Is an Integer in Python In the world of programming, we work very frequently with the input of the user. Super Jungle Run Adventure. The isinstance () function can be utilized for checking whether any given variable is of the desired data type or not. It tests for digit characters, not for strings that represent numbers. For example, '-1'.isdigit () is False. Python check if a variable is an integer isinstance method. var = "String" if type (var) == str: print ("String type") Output: String type. Execution -2. colors = [11, 34.1, 98.2, 43, 45.1, 54, 54] for x in colors: if int(x) == x: print(x) #or if isinstance(x, int): print(x)
Here is a simple program to get the file extension in Python.Check Data Type of Integer Variable. var1 = "Hello" var2 = 123 var3 = "123" # using isstance() res_var1 = isinstance(var1, str) res_var2 = isinstance(var2, str) res_var3 = isinstance(var3, str) # print result print("Is variable a string ? There is no try.' (Python recipe) Workaround for the SQLite limitation that prevents multiple threads from sharing a Connection object. Example 2: INPUT: [3,7,9,10,5] 8 OUTPUT:[0,4] Logic: A simple method is to use a two nested loop and generate all the pairs and check for their sum. A basic approach to do this check is to use a for loop that goes through every character of the string and checks if that character is a number by using the string isdigit() method.. Method #1 : Using isinstance (x, str) This method can be used to test whether any variable is a particular datatype. x = 'abcd' isinstance(x, (int, float)) returns.
Created: March-14, 2021 | Updated: March-21, 2021.
The
Please show all steps beginning toIn C++, an array is a variable that can store multiple values of the same type. If it is not then set a counter and break. Yes it is string variable. When we say a number, it means that it can be an integer or a float. (ignored by fixed wing, time to stay at MISSION for rotary wing) | Acceptance radius in meters (if the Max int in Python 2. type (a) == int or isinstance (a, int). The first is your variable. The second is the type you want to check for.
Resx. Python: Add Variable to String & Print Using 4 Methods. For example, print( (10.0).is_integer()) print( (15.23).is_integer()) This will give the output.. Next: Add Python to PATH in User Variables. Python Example #2: Twitter API call. except ValueError: As you know, Pythons input() function always converts the user input to a string. The int datatype is used to represent integers. let's say that you want to test or check if a variable is a string, see the code bellow Data Types in Python str int float complex list tuple range dict bool bytes Related posts. To check if a python variable is a number (int or float par example), a solution is to use isinstance: x = 1.2 isinstance(x, (int, float)) returns here. How to create a string in Python + assign it to a variable in python; How to create a variable in python; Function in Python; Example: Iterate for every character and check if the character is 0 or 1. VariablesGeneral Concept of Variables. This subchapter is especially intended for C, C++ and Java programmers, because the way these programming languages treat basic data types is different from the way Variables in Python. There is no declaration of variables required in Python, which makes it quite easy. Object References. Use the int() Method to Check if an Object Is an int Type in Python Use the float.is_integer() Method to Check if an Object Is an int Type in Python In Python, we have different datatypes when we deal with numbers. Description. Uses the type built-in function to see if the type of your variable is str. The code example below shows how we can use it. True For a sum of '14', the check digit is '6' since '20' is the next number divisible by ten. Write a python program to check if the number given is a palindrome or not; Pattern questions. If we want to ensure that a variable stores a particular data type, we can use the isinstance() function. Write a Python program to check whether a variable is integer or string. cannot apply java lang integer android. conn = sqlite3.connect ('db.sqlite3') If you run the code above, it will create a database file in the folder you run it in.MultiThread support for SQLite access. Angry Zombies. Do you want an array of int?Then create a TArray
In this tutorial, we will discuss how to check if a variable is int or not. To check if a variable is a String in Python, use the type() function and compare the output of the type() function to the str class, and if it returns True, then a variable is String; otherwise not. On windows laptop:-. The type() is a built-in Python function that True b = 3 c = 3.4 print (isinstance (b,int)) print (isinstance (c,int)) Here is the screenshot of following given code. You use local variable in component template. Lets take an example to check if a variabe is an integer. Fri Feb 4 2022 by Luis Hctor Chvez. Code snippet for string indexer annotation . In our case, we are testing string type so the second parameter will be a string type. The isinstance function takes two arguments. Using a For Loop and isdigit() To Find Out if a String Contains Numbers. BytesParsing.sol convert java to c. android java convert boolean to string. How to insert .format multiple variables in Python. There is a max int limit in Python 2, and if you cross the max limit, it would automatically switch from int to long. It will "return true if all characters in the string are digits and there is at least one character, false otherwise: It will "return true if all characters in the string are digits and there is at least one character, false otherwise: After writing the above code (python check if the variable is an integer), Ones you will print isinstance() then the output will appear as The value is a string data type with a length of four characters. If the input string is a number, It will get converted to int or float without exception. After writing the above code (python check if the variable is an integer), Ones you will print isinstance() then the output will appear as Method #1: Using isdigit() method Please enter number or string or both test556 str does not contain a number Python check if given string contains only letter.
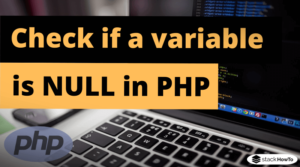

This method can be used to check if a variable is specific data type. >>>isinstance(1,int)
count = 0. for char in string: To check if the variable is an integer in Python, we will use isinstance() which will return a boolean value whether a variable is of type integer or not. var = 1 if type(var) == int: print('your variable is an integer') or: var2 = 'this is variable #2' if type(var2) == str: print('your variable is a string') else: print('your variable IS NOT a string') hope this helps! Another Example. Explicitly test if there are only digits in the string: value.isdigit() str.isdigit() returns True only if In using this value, I noticed multiplying 4.56 by 100 returns 455.99999999999994 instead of 456. Python check if the variable is an integer. Example. The isinstance function takes two arguments. ctx. How to check if a variable is a string. This is the Ask Forgiveness approach. If it returns True, Check Python variable is of an integer type or not. Write a python program to check if the number given is a palindrome or not; Pattern questions. Just try to convert it to an int, but catch the exception: try: value = int(value) except ValueError: pass # it was a string, not an int. Solution: In such a situation, We need to convert user input explicitly to integer and float to check if its a number. : " + str(res_var2)) print("Is variable a string ? These are the int, float, and complex datatypes. If you want to add new items to the dictionary using Python. I was using the Python interpreter to test my workflow, and chose 4.56 as a random test value. Different ways to get the number of words in a string in Python. python to java code conversion. y: This here can be any data type that you want to check the variable for. An integer variable is a variable with a numeric value. Use the type() Function to Check if a Variable Is a String or Not ; Use the isinstance() Function to Check if a Variable Is a String or Not ; The string datatype is used to represent a collection of characters. You can also use the isinstance(val, type) method of python to check whether a value is an integer or not. The str.isdigit () method returns True if the string represents an integer, otherwise False. So the answer is '1 + 8 + 3 + 2 = 14' and the check digit is the amount needed to reach a number divisible by ten. Note: IDE: PyCharm 2021.3 (Community Edition) Windows 10. def check2 (string): t = '01'. !! Using numbers.Number () method. Next, we used the python function to sort them in ascending order.Introduction to Selection Sort in Python. Note that f-strings are not used in the example to preserve compatibility with versions below Python 3.6.Once you finish your discord bot, attach a simple web server into it. Use the int() Function to Check if the Input Is an Integer in Python ; Use the isnumeric() Method to Check if the Input Is an Integer or Not ; Use the Regular Expressions to Check if the Input Is an Integer in Python In the world of programming, we work very frequently with the input of the user. Super Jungle Run Adventure. The isinstance () function can be utilized for checking whether any given variable is of the desired data type or not. It tests for digit characters, not for strings that represent numbers. For example, '-1'.isdigit () is False. Python check if a variable is an integer isinstance method. var = "String" if type (var) == str: print ("String type") Output: String type. Execution -2. colors = [11, 34.1, 98.2, 43, 45.1, 54, 54] for x in colors: if int(x) == x: print(x) #or if isinstance(x, int): print(x)
