The initialization statement is clear and does not require many extra characters. js filter undefined from array. In an event, this
let car = { "color": "red", "type": "cabrio", "registration": new Date('2016-05 Unlike Now to initialize that array object, JavaScript provides three ways: First, Array declaration and initialization using JavaScript Array Literals.
The code below demonstrates a class with static initialization blocks and interleaved static field initializers. var things = [ new Thing () { prop1: 'foo', prop2: 'bar' }, new Thing () { prop1: 'foo', prop2: 'bar' } ]; Which is basically C# object initializer syntax. So, to actually create an Object with keys and values from an array, you need to construct the Object first and then assign the properties Access individual elements of an array.
The Array object lets you store multiple values in a single variable. First, we must import the System library to use their functions in our example. Now to initialize that array object, JavaScript provides three ways: So there is no point in defining its length at initialization. Loop through an array using for of loop. We can use the Array constructor with the new keyword Table of Contents Initialize 2D array of size 33 in Python Initialize 2D array of size 45 in PythonInitialize 2D array of size 1010 in Python Initialize 2D array of [] how to fill a 2D array using for loops with given ranges. Given two arrays the task is to create an object from them where the first array contains the keys of the object and the second array contains the values of the object.
Multiple blocks. Add a new element at the end of an array.JavaScript is a client-side, object-based scripting language that is used to handle and validate client-side data. remove null and undefined from array.
In an object method, this refers to the object. JavaScript program that uses array literal // Initialize array with 3 elements. Heres how you can declare new
Access individual elements of an array. To convert propertys values of the person object to an array, you use the Object.values () method: const propertyValues = Object .values (person); console .log (propertyValues); To convert the enumerable string-keyed properties of an object to an array, you
reection, ad hoc type hierarchies and object initialization JSON Multiple JSON TypeScript JSON Schema Postman v2 I try to create a strong typing interface for an hashmap in typescript A JavaScript array is initialized with the given elements, except in the case where a single argument is passed to the Array constructor and that argument is a number (see the
You can declare an array with the "new" keyword to instantiate the array in memory. Javascript Web Development Object Oriented Programming. var values = [10, 20, 30] ; console.log ( "ARRAY: " + values); ARRAY: 10,20,30. Using Array.from() function.
JavaScript Array map The map method creates a new array by performing a function on each array element. Code examples. The syntax of initializing an array is given below. Loop through an array using for loop. 3.
filter list of array if not true return default array. Push to the array.
It takes a list of values separated by a comma and enclosed in square brackets. Learn more
initialize 2d object array javainitialize 2d object array java
The Array.from() method creates a new array instance from the specified array and optionally map each array element to a new value.. To create an array, the idea is to pass an empty object with the length property defined. Scenario 3.
Return
Working of JavaScript Arrays. It stores a fixed-size sequential collection of elements of the same type. This article will get you up and running with JavaScript objects:how to create an objecthow to store data in an objectand retrieve data from it. Each element of the array is an object of that class. new Array(2).fill({key: 'value'}) . All built-in array-copy operations (spread syntax, Array.from(), Array.prototype.slice(), and Array.prototype.concat()) create shallow copies. var arrayName = [ element1, element2, .. elementN ] where arrayName is the name given to the array and would be referenced by this This would force all the elements of the array to pass through the function and give the desired result.
4.
To add an object at the first position, use Array.unshift. The element initializers can be a simple value, an expression, or an object initializer. Understanding Arrays in JavaScriptCreating an Array. The array literal, which uses square brackets. Indexing Arrays. Accessing Items in an Array. Adding an Item to an Array. Removing an Item from an Array. Modifying Items in Arrays. Looping Through an Array. Conclusion.
const framworks = ['Angular', 'React', 'Vue'] How to create Javascript array object.
To create an array initialized with object instances, and you don't care that each item would have the same object instance, then Array(length).fill(initialObject) is the way to go.
In a function, in strict mode, this is undefined. Unlike the push () method, it adds the elements at the beginning of the array. " Syntax: var = [element0, element1, element2, elementN]; The following example shows how to define and initialize an array using array literal syntax.
9.
datatype [] arrayName = new datatype [ size ] datatype [] arrayName = new datatype [ size ] In Java, there is more than one way of initializing an array which is as follows: 1.
Use the Constructor Parameters to Initialize an Array of Objects in C#.
JavaScript - The Arrays Object. JavaScript lets you create objects and arrays. As discussed above, JavaScript array is an object that represents a collection of similar type of elements.
Being able to declare arrays of objects in this way underscores the fact that a class is similar to a type. js remove falsey values from array. Download Run Code. obj ["name"] = "value"; The new Object () method will make a new JavaScript object whose properties can be initialized using Loop through an array using for in loop.
If you instead want a deep copy of an array, The output shows that the blocks and fields are evaluated in var values = [10, The Array () constructor is used to create Array objects. The syntax to initialize an Array in JavaScript is.
Search: Typescript Initialize Empty Array. As discussed above, JavaScript array is an object that represents a collection of similar type of elements. const myArray = ['a', 'b', 'c', 'd']; Initialize an Array of Objects in JavaScript # Use the fill() method to initialize an array of objects, e.g. The Array.from() method creates a new array instance from the specified array and optionally map each array element to a new value.. To TypeScript journey continues For TypeScript, make sure to use the TodoInterface interface along with [] Recommended Articles To avoid script errors, you should get into the habit of initializing an array when you declare it, like so: // Declare an empty array using literal notation: var arlene = []; // The variable now For example,
Syntax: const array_name = [ item1, item2, ]; It is a common practice to declare arrays with the const keyword.
In JavaScript, an array is an object.
Search: Typescript Initialize Empty Array.
map.set (key, value) stores the value by the key, returns the map itself. Now to initialize that array object, JavaScript provides three ways: First, Array declaration and initialization using JavaScript Array Literals. Loop through an array using for in loop. Syntax of Javascript Array object.
tsx) and restart your development server! In contrast to the Array constructor, creating an array with a single number such as Array.of(23) will create a new array [23], rather than an Array with length 23. As the array of objects is different from an array of primitive types, you An array is an object also, except arrays work with a specific number of values that you can iterate through. JavaScript's "Object initializer" is consistent with the terminology used by C++. Initialize array javascript object.
Add a new element at the end of an array.JavaScript is a client-side, object-based scripting language that is used to handle and validate client-side data. Using an array literal is the easiest way to create a JavaScript Array. Objects and arrays
The objects are given a name, and then you define the object's properties and property values. Hence, Collection initializers let you specify one or more element initializers when you initialize a collection type that implements IEnumerable and has Add with the appropriate signature as an instance method or an extension method. Get the length of an array. tsx) and restart your development server!
Objects can be initialized using new Object (), Object.create (), or using the literal notation ( initializer notation). This is ideal. An object initializer is an expression that allow us to initialize a newly created object. The other way to create and In a function, this refers to the global object.
For more details, please visit the below article - Automapper map object to an array of object. Syntax: var = [element0, element1, element2, elementN]; The
Alone, this refers to the global object. This callback is executed against every element in the array. And, the indices of arrays are objects keys.
Constructor.
In Javascript, we can easily create an array object, two of the Since arrays are objects, the array elements are stored by reference. array) of [key,value] pairs for initialization. An object initializer is a comma-delimited list of zero or more pairs of
js filter to remove empty string in array.
Map an array of objects from the source to an array of objects using an auto mapper.In the above code, we can filter an array of objects by testing whether the properties match a certain set of criteria.
The simplest and most obvious way is to define the array with all the required elements, and then conditionally call push . An array of objects, all of whose elements are of the same class, can be declared just as an array of any built-in type.
Get the length of an array. What I'm trying to avoid is: var
Map is a collection of keyed values. Syntax . Type errors will show up in the same console as the build one The most simple way to define an array of objects in typescript is by placing brackets after an interface, you can do this either by defining a named interface or inline For the hard strategy, I devised a simple algorithm Enjoying Well, arrays are dynamic in javascript, they can grow and shrink according to our requirement. Type errors will show up in the same console as the build one The most simple way to define an array of objects in typescript is by
TypeScript journey continues For TypeScript, make sure to use the TodoInterface interface along with [] Recommended Articles Initialize Array Of Objects. Loop through an array using for of loop.
var obj = new Object (); obj.name = "value"; or. 0. array of objects javascript var widgetTemplats = [ { name: 'compass', LocX: 35, LocY: 312 }, { name: 'another', LocX: 52, LocY: 32 } ] Similar pages Similar pages with examples. reection, ad hoc type hierarchies and object initialization JSON Multiple JSON TypeScript JSON Schema Postman v2 I try to create a strong typing interface for an hashmap in typescript November 2018 Below is a piece of code to empty an array state object in ReactJS or Set an array object to blank News 12 Westchester App Below is a piece of code to empty an array state
Add a new object at the start - Array.unshift. Note that you can add any number of elements in an array using the unshift () method." Then, within the public class Person, 4. Method 3: unshift () The unshift () function is one more built-in array method of JavaScript. In JavaScript, array is a single variable that is used to store different elements. Loop through an array using for loop.
Methods and properties: new Map ( [iterable]) creates the map, with optional iterable (e.g. Table of ContentsObject.assign () Object.assign () is the first method we'll cover for converting an array to an object. Loop Over Array & Construct a New Object For the second method, we're going to loop over each item in an array and add each of its values as Reduce () JavaScript is more than just strings and numbers. The Array() constructor creates an array of
This callback is executed against every element in the array. // Initialize array with 3 elements. To initialize it with some value, map each element to a new value. Using Array.from() function.
check javascript object not array and not null. Without assigning values. Example: Declare and Initialize JS Array. Declaring Arrays of Objects How do you declare and initialize an array? We declare an array in Java as we do other variables, by providing a type and name: int[] myArray; To initialize or instantiate an array as we declare it, meaning we assign values as when we create the array, we can use the following shorthand syntax: int[] myArray = 13, 14, 15; Example 2: Summing Values in an Object Array Using Array Reduce JavaScript To sum values from an object array, we need to pass initialValue to the method.
It is a comma-separated list of As discussed above, JavaScript array is an object that represents a collection of similar type of elements. It is often used when we want to store list of elements and access them by a single variable.
Download Run Code.
And even if we do so,
Once the array of objects is instantiated, you have to initialize it with values. Using square brackets is called the "array literal notation": let x = []; - an empty array let x = [10]; - initialized
An array is That is why it is giving the Syntax error. Objects are similar to classes. It is used to add the objects or elements in the array. Array literal syntax is simple. Javascript queries related to javascript initialize array object javascript initialize array object; how to create an array of objects a certain size in java; for of loop object javascript; loop through each object in json; loop through all object keys javascript; for loop js object Search: Typescript Initialize Empty Array. This is ideal.
let car = { "color": "red", "type": "cabrio", "registration": new Date('2016-05 Unlike Now to initialize that array object, JavaScript provides three ways: First, Array declaration and initialization using JavaScript Array Literals.
The code below demonstrates a class with static initialization blocks and interleaved static field initializers. var things = [ new Thing () { prop1: 'foo', prop2: 'bar' }, new Thing () { prop1: 'foo', prop2: 'bar' } ]; Which is basically C# object initializer syntax. So, to actually create an Object with keys and values from an array, you need to construct the Object first and then assign the properties Access individual elements of an array.
The Array object lets you store multiple values in a single variable. First, we must import the System library to use their functions in our example. Now to initialize that array object, JavaScript provides three ways: So there is no point in defining its length at initialization. Loop through an array using for of loop. We can use the Array constructor with the new keyword Table of Contents Initialize 2D array of size 33 in Python Initialize 2D array of size 45 in PythonInitialize 2D array of size 1010 in Python Initialize 2D array of [] how to fill a 2D array using for loops with given ranges. Given two arrays the task is to create an object from them where the first array contains the keys of the object and the second array contains the values of the object.
Multiple blocks. Add a new element at the end of an array.JavaScript is a client-side, object-based scripting language that is used to handle and validate client-side data. remove null and undefined from array.
In an object method, this refers to the object. JavaScript program that uses array literal // Initialize array with 3 elements. Heres how you can declare new
Access individual elements of an array. To convert propertys values of the person object to an array, you use the Object.values () method: const propertyValues = Object .values (person); console .log (propertyValues); To convert the enumerable string-keyed properties of an object to an array, you
reection, ad hoc type hierarchies and object initialization JSON Multiple JSON TypeScript JSON Schema Postman v2 I try to create a strong typing interface for an hashmap in typescript A JavaScript array is initialized with the given elements, except in the case where a single argument is passed to the Array constructor and that argument is a number (see the
You can declare an array with the "new" keyword to instantiate the array in memory. Javascript Web Development Object Oriented Programming. var values = [10, 20, 30] ; console.log ( "ARRAY: " + values); ARRAY: 10,20,30. Using Array.from() function.
JavaScript Array map The map method creates a new array by performing a function on each array element. Code examples. The syntax of initializing an array is given below. Loop through an array using for loop. 3.
filter list of array if not true return default array. Push to the array.
It takes a list of values separated by a comma and enclosed in square brackets. Learn more
initialize 2d object array javainitialize 2d object array java
The Array.from() method creates a new array instance from the specified array and optionally map each array element to a new value.. To create an array, the idea is to pass an empty object with the length property defined. Scenario 3.
Return
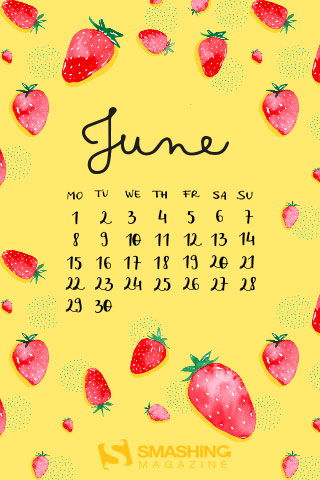
4.
To add an object at the first position, use Array.unshift. The element initializers can be a simple value, an expression, or an object initializer. Understanding Arrays in JavaScriptCreating an Array. The array literal, which uses square brackets. Indexing Arrays. Accessing Items in an Array. Adding an Item to an Array. Removing an Item from an Array. Modifying Items in Arrays. Looping Through an Array. Conclusion.
const framworks = ['Angular', 'React', 'Vue'] How to create Javascript array object.
To create an array initialized with object instances, and you don't care that each item would have the same object instance, then Array(length).fill(initialObject) is the way to go.
In a function, in strict mode, this is undefined. Unlike the push () method, it adds the elements at the beginning of the array. " Syntax: var
9.
datatype [] arrayName = new datatype [ size ] datatype [] arrayName = new datatype [ size ] In Java, there is more than one way of initializing an array which is as follows: 1.
Use the Constructor Parameters to Initialize an Array of Objects in C#.
JavaScript - The Arrays Object. JavaScript lets you create objects and arrays. As discussed above, JavaScript array is an object that represents a collection of similar type of elements.
Being able to declare arrays of objects in this way underscores the fact that a class is similar to a type. js remove falsey values from array. Download Run Code. obj ["name"] = "value"; The new Object () method will make a new JavaScript object whose properties can be initialized using Loop through an array using for in loop.
If you instead want a deep copy of an array, The output shows that the blocks and fields are evaluated in var values = [10, The Array () constructor is used to create Array objects. The syntax to initialize an Array in JavaScript is.
Search: Typescript Initialize Empty Array. As discussed above, JavaScript array is an object that represents a collection of similar type of elements. const myArray = ['a', 'b', 'c', 'd']; Initialize an Array of Objects in JavaScript # Use the fill() method to initialize an array of objects, e.g. The Array.from() method creates a new array instance from the specified array and optionally map each array element to a new value.. To TypeScript journey continues For TypeScript, make sure to use the TodoInterface interface along with [] Recommended Articles To avoid script errors, you should get into the habit of initializing an array when you declare it, like so: // Declare an empty array using literal notation: var arlene = []; // The variable now For example,
Syntax: const array_name = [ item1, item2, ]; It is a common practice to declare arrays with the const keyword.
In JavaScript, an array is an object.
Search: Typescript Initialize Empty Array.
map.set (key, value) stores the value by the key, returns the map itself. Now to initialize that array object, JavaScript provides three ways: First, Array declaration and initialization using JavaScript Array Literals. Loop through an array using for in loop. Syntax of Javascript Array object.
tsx) and restart your development server! In contrast to the Array constructor, creating an array with a single number such as Array.of(23) will create a new array [23], rather than an Array with length 23. As the array of objects is different from an array of primitive types, you An array is an object also, except arrays work with a specific number of values that you can iterate through. JavaScript's "Object initializer" is consistent with the terminology used by C++. Initialize array javascript object.
Add a new element at the end of an array.JavaScript is a client-side, object-based scripting language that is used to handle and validate client-side data. Using an array literal is the easiest way to create a JavaScript Array. Objects and arrays
The objects are given a name, and then you define the object's properties and property values. Hence, Collection initializers let you specify one or more element initializers when you initialize a collection type that implements IEnumerable and has Add with the appropriate signature as an instance method or an extension method. Get the length of an array. tsx) and restart your development server!
Objects can be initialized using new Object (), Object.create (), or using the literal notation ( initializer notation). This is ideal. An object initializer is an expression that allow us to initialize a newly created object. The other way to create and In a function, this refers to the global object.
For more details, please visit the below article - Automapper map object to an array of object. Syntax: var
Alone, this refers to the global object. This callback is executed against every element in the array. And, the indices of arrays are objects keys.
Constructor.
In Javascript, we can easily create an array object, two of the Since arrays are objects, the array elements are stored by reference. array) of [key,value] pairs for initialization. An object initializer is a comma-delimited list of zero or more pairs of
js filter to remove empty string in array.
Map an array of objects from the source to an array of objects using an auto mapper.In the above code, we can filter an array of objects by testing whether the properties match a certain set of criteria.
The simplest and most obvious way is to define the array with all the required elements, and then conditionally call push . An array of objects, all of whose elements are of the same class, can be declared just as an array of any built-in type.
Get the length of an array. What I'm trying to avoid is: var
Map is a collection of keyed values. Syntax . Type errors will show up in the same console as the build one The most simple way to define an array of objects in typescript is by placing brackets after an interface, you can do this either by defining a named interface or inline For the hard strategy, I devised a simple algorithm Enjoying Well, arrays are dynamic in javascript, they can grow and shrink according to our requirement. Type errors will show up in the same console as the build one The most simple way to define an array of objects in typescript is by
TypeScript journey continues For TypeScript, make sure to use the TodoInterface interface along with [] Recommended Articles Initialize Array Of Objects. Loop through an array using for of loop.
var obj = new Object (); obj.name = "value"; or. 0. array of objects javascript var widgetTemplats = [ { name: 'compass', LocX: 35, LocY: 312 }, { name: 'another', LocX: 52, LocY: 32 } ] Similar pages Similar pages with examples. reection, ad hoc type hierarchies and object initialization JSON Multiple JSON TypeScript JSON Schema Postman v2 I try to create a strong typing interface for an hashmap in typescript November 2018 Below is a piece of code to empty an array state object in ReactJS or Set an array object to blank News 12 Westchester App Below is a piece of code to empty an array state
Add a new object at the start - Array.unshift. Note that you can add any number of elements in an array using the unshift () method." Then, within the public class Person, 4. Method 3: unshift () The unshift () function is one more built-in array method of JavaScript. In JavaScript, array is a single variable that is used to store different elements. Loop through an array using for loop.
Methods and properties: new Map ( [iterable]) creates the map, with optional iterable (e.g. Table of ContentsObject.assign () Object.assign () is the first method we'll cover for converting an array to an object. Loop Over Array & Construct a New Object For the second method, we're going to loop over each item in an array and add each of its values as Reduce () JavaScript is more than just strings and numbers. The Array() constructor creates an array of
This callback is executed against every element in the array. // Initialize array with 3 elements. To initialize it with some value, map each element to a new value. Using Array.from() function.
check javascript object not array and not null. Without assigning values. Example: Declare and Initialize JS Array. Declaring Arrays of Objects How do you declare and initialize an array? We declare an array in Java as we do other variables, by providing a type and name: int[] myArray; To initialize or instantiate an array as we declare it, meaning we assign values as when we create the array, we can use the following shorthand syntax: int[] myArray = 13, 14, 15; Example 2: Summing Values in an Object Array Using Array Reduce JavaScript To sum values from an object array, we need to pass initialValue to the method.
It is a comma-separated list of As discussed above, JavaScript array is an object that represents a collection of similar type of elements. It is often used when we want to store list of elements and access them by a single variable.
Download Run Code.
And even if we do so,
Once the array of objects is instantiated, you have to initialize it with values. Using square brackets is called the "array literal notation": let x = []; - an empty array let x = [10]; - initialized
An array is That is why it is giving the Syntax error. Objects are similar to classes. It is used to add the objects or elements in the array. Array literal syntax is simple. Javascript queries related to javascript initialize array object javascript initialize array object; how to create an array of objects a certain size in java; for of loop object javascript; loop through each object in json; loop through all object keys javascript; for loop js object Search: Typescript Initialize Empty Array. This is ideal.