This example displays the dimensions of a box in both metric and English units. I am not aware a limit exists. I think you should be able to implement as many as you want. Extra tip: While learning to program you will have many 3) A class can implement multiple interfaces. In case of multiple interfaces with the same default method. Each interface specifies a well-defined set of operations that have public visibility. As you already know a class can implement any number of interfaces, but it can extend only one class. Here is an example of how to extends a class in java. Implement multiple interfaces: 7. This interface is found in java.lang package and contains only one method named compareTo (Object). An interface should be implemented by both the real class and the mock class to guarantee that the mock class accurately simulates the real class when used in a program. Initializing interface fields with non-constant initializers: 3. default p Multi Super Interfaces: 8. What does that mean, and what is it good for? It is a very core concept to object-oriented orthodoxy and I first learned about it when C++ gurus made "pure abstract classes" which are quite equivalent to To use an interface, another class must implement it by providing a body for all of the methods contained in the interface. Yes multiple classes can implement one interface irrespective of being in the same program or different. There is no such restriction. Interface on All the methods defined in an interface should be implemented. See the answer Classes can implement an interface while inheriting from another class at the same time; PHP - Using Interfaces. To use an interface, another class must implement it by providing a body for all of the methods contained in the interface.
3) A class can implement multiple interfaces. Answer (1 of 4): There is a misconception implicit in the question. Beginning with C# 8.0, an interface may define a default implementation for members. A class or struct that implements an interface shall adhere to its contract. You can declare abstract methods in Yes, multiple (any number of) classes can implement one interface. Multiple inheritance using classes is not supported in Java & leads to a very famous problem known as Diamond problem where as it is supported using interfaces. , Oops ! Yes multiple classes can implement one interface irrespective of being in the same program or different. A class can implement any number of interfaces but can extend only one class. // This method gets called by the runtime. While working with the interface, make sure the class implements all its abstract methods. Allows 2 incompatible interfaces to work together. See the example below where we implemented all the methods of all 3 interfaces. All methods in the interface are implicitly public and abstract. Does Java support multiple inheritance through interface? In TypeScript, a class can implement interfaces to enforce particular contracts (similar to languages like Java and C#). This problem has been solved! Obviously you are going to never run into these limitations in practice. While working with the interface, make sure the class implements all its abstract methods.
As you can see I have implemented the Comparable interface in my Author class because I want to sort the objects of this class. a. forces classes that implement it to declare all the abstract interface methods. To declare a class that implements an interface, you include an implements clause in the class declaration. Java allows a class to implement multiple interfaces. We use the interface keyword to create an interface in Java. c) can extend any number of classes but can implement at most one interface.
Each interface contains a method declaration. 13) If there are two or more same methods in two interfaces and a class implements both interfaces, implementation of the method once is enough. The best way to tackle this is to throw Not-implemented Exception in the concrete class. (C) An unlimited number of unrelated classes can implement the same interface. You can provide an empty implementation for all the methods of an interface in other class called Adaptor class. And you can extend that adaptor cl So, the general rule is extend one but implement many . Multiple interfaces: 5. Example: using System; interface G1 { void mymethod (); } interface G2 { void mymethod (); } class Geeks : G1, G2 { Interfaces can also be considered an abstract class which group similar methods without any implementation. as given in the first example? No, in java you have to implement all metho Interfaces. The given program is compiled and executed successfully on Microsoft Visual Studio. (B) A class can implement many interfaces but can have only one superclass. Abstract class should be used for classes which are closely related to each other. class Add implements Icalculate{ //addit No, in java you have to implement all methods of interface unless its abstract class as suggestion you can create two separate interfaces, for more detail see : not implementing all of the methods of interface.
This can be achieved using inheritance by either inheriting from a superclass (base class), or by implementing one or many interfaces (as @Oded said, many small interfaces instead of one big interface). By convention, the implements clause follows the extends clause, if there is one.. A Sample Interface, Relatable An interface is like an Apex class in which none of the methods have been implemented. In Line 18, you can see that the Square class will implement the two interfaces. Here is a work around and we can handle such situations with little bit decorating our interface like this: Now our interface will look like this: C#. Yes, a class can implement two two interfaces with the same method signature but that method should be implemented only once in the class.
Although there is a lesser understood benefit to using inheritance to be discussed later, the primary benefit is to share implementation between Multi Super Interfaces: 8. Before Java 8, Interfaces could have only abstract methods. In case of Abstract Class, you can take advantage of the default implementation. Interfaces are a tool for defining contracts between multiple subsystems of your application; so what really matters is how your application is divided into subsystems. It includes abstract methods: getType () and getVersion (). Yes multiple classes can implement one interface irrespective of being in the same program or different. 4) Many classes can implement the same interface. The given program is compiled d. can be instantiated. However, there is a work-around to this which involves the use of Interfaces. In this article. We can achieve multiple inheritances by the use of interfaces. In the interface body, define the needed properties, methods, and types; Implement an interface on a class with the implements keyword; Write the needed code for every property and method defined in the interface; Use an interface as a data type by writing the name of the interface after a colon like so games: IGame[] = [] # A class can implement any number of interfaces but can extend only one class. is declared in a file by itself and is saved in a file with the same name as the interface followed by the .java extension. 11) An interface can extend any interface but cannot implement it. You can use interfaces in class diagrams and component diagrams to specify a contract between the interface and the classifier that realizes the interface. An abstract class is nothing but a class that is declared using the abstract keyword. A class can implement several interfaces, thus providing similar features that are provided by multiple inheritance. Example: Sorting Custom object by implementing Comparable interface. Using the Code. ____________ can reduce the coupling between classes. Overview. Object-oriented programming in Java requires to "override" all methods, if you are implementing a method, otherwise you may use inheritance, so not Click Next. Multiple Inheritance in C# using Interfaces. Interface inheritance defines a new interface in terms of one or more existing interfaces. Unlike classes, interfaces are always completely abstract. An Interface in Java programming language is defined as an abstract type used to specify the behavior of a class. Its also called Java extends the class. Yes, Any number of classes can implement a single interface. In case of multiple interfaces with the same default method.
Interfaces can contain methods, properties, events, and indexers. An interface may inherit from multiple base interfaces, and a class or struct may implement multiple interfaces. Live Demo. An interface is like a class in which none of the methods have been implementedthe method signatures are there, but the body of each method is empty. Interfaces can provide a layer of abstraction to your code. Classes can implement an interface while inheriting from another class at the same Can an abstract class inherit an interface? Another option that comes to my mind is to implementing mixin in C#, but I'm not a pro at it. use an additional generic type parameter on the interface or a new interface implementing the non generic interface, implement an adapter/interceptor class to add the marker type and then ; use the generic type as name Ive written an article with more details: Dependency Injection in .NET: A way to work around missing named registrations By itself, implementing interfaces has no performance impact.
3. It therefore cannot inherit from a class. Which of the following is true about interfaces in java. Interfaces A and B both declare functions foo() and bar().Both of them implement foo(), but only B implements bar() (bar() is not marked as abstract in A, because this is the default for interfaces if the function has no body).Now, if you derive a concrete class C from A, you have to override bar() and provide an implementation.. is it possible? calculate(operand1:number,operand2:number):number When an Abstract Class Implements an Interface. In Java, an interface is not a class. Interface implementation is encoded in metadata in the InterfaceImpl table. is checks a given object implements or extends interface or class, It checks parent class hierarchy and returns true if it follows inheritance. runtimeType: Returns the class of an object and gives the only current class of an object not the inheritance tree class checking if an object implements interface in dart? Your class can implement more than one interface, so the implements keyword is followed by a comma-separated list of the interfaces implemented by the class. b) can extend any number of classes and can implement any number of interfaces. See the answer See the answer done loading. 3) A class can implement multiple interfaces. In Java, interfaces are declared using the interface keyword. An abstract class can inherit a class and multiple interfaces. It just defined the contract implementing by concrete classes. I would answer that whether you need an interface or not does not depend on how many classes will implement it. To use an interface, another class must implement it by providing a body for all of the methods contained in the interface. An interface defines a contract. Interface Usage Example: 7. For most intents and purposes you don't need to implement an interface on the derived class. An interface contains definitions for a group of related functionalities that a non-abstract class or a struct must implement. Now we are back to ConfigureServices method from the beginning of this article where for the same interface we register multiple class types. An interface or abstract class is the same. An interface may define static methods, which must have an implementation. Unlike classes, interfaces are always completely abstract. Use of interfaces helps a system stay decoupled and thus easier to refactor, change, and redeploy. An interface is a fully abstract class. An interface in Java is essentially a special kind of class. 1) To achieve security - hide certain details and only show the important details of an object (interface). In the Create new project window, select ASP.NET Core Web Application from the list of templates displayed. When one or more classes use the same interface, it is referred to as "polymorphism". 4) Many classes can implement the same interface. Program: The source code to implement multiple interfaces in the same class is given below. Interfaces make it easy to use a variety of different classes in the same way. Youre actually describing an abstract base class or ABC. Many OOP languages allow you to create a class with code in it, but mark one or more me extends keyword is used to inherit a class; while implements keyword is used to inherit the interfaces. A class can extend only one class; but can implement any number of interfaces. A subclass that extends a superclass may override some of the methods from superclass. A class must implement all the methods from interfaces. Any class can work together as long as the Adapter solves the issue that all classes must implement every method defined by the shared interface. Interface (If required): A class may implement any interface if required. 4) Many classes can implement the same interface.
It is possible, however, to define a class that does not implement all of the interface's methods, provided that the class is declared to be abstract. A Java interface contains static constants and abstract methods. To use interface in the java code, implements keyword is used.
Interfaces summary. Multiple inheritance using interface in java. A class can implement more than one interface at a time. December 14, 2018. A Java class. (So altogether, N + This shows that a class implementing an interface need not: 9. Another advantage is that multiple different representations of the abstract data type can coexist in the same program, as different classes implementing the interface. Interface Usage Example: 6. Does Java support multiple inheritance through interface? The interface in Java is a mechanism to achieve abstraction.There can be only abstract methods in the Java interface, not the method body. An interface in Java is defined as an abstract type that specifies class behavior. There is no such restriction. Because both are included, all members of both interfaces must be implemented by the Square class. Explicit interface implementation also allows the programmer to implement two interfaces that have the same member names and give each interface member a separate implementation. All methods in an interface are abstract, so they cannot be implemented in code and the abstract keyword is not necessary. When it comes to building applications with a graphical user interface (GUI), inheritance is arguably the most important mechanism for making it possible to quickly build an application. As much as it looked wrong in the first place, .NET Core dependency injection container can handle this king of interface implementation registration. 3. Answer (1 of 11): The short answer is NO. Can I avoid the above @Override and just use the classes without these Which of the following is true about interfaces in java. If you notice that many classes belong to a parent class with the same method actions but different behavior, then it's a good idea to use an interface. This shows that a class implementing an interface need not: 9. Find out whether interfaces are inherited: 10. public, static, final fields (i.e., constants) default and static methods with bodies 2) An instance of interface can be created. I would say that the limit should be a smaller number than 23 - more like 5 or 7, however, this does not include any number of interfaces that those interfaces inherit or any number of interfaces implemented by base classes. Share Improve this answer answered Nov 27, 2017 at 6:37 Keval 1,837 15 26 An Interface in Java programming language is defined as an abstract type used to specify the behavior of a class. I have written the logic of sorting in the compareTo() method, you can write logic based on the requirement. Question 1 options: a) can extend at most one class but can implement any number of interfaces. The preceding output creates more confusion about which method of which interface is implemented. (A) An interface cannot implement any non-default instance methods, whereas an abstract class can. c. is declared in a file by itself and is saved in a file with the same name as the interface followed by the .java extension. Any attempt to access old contracts would give a Java supports multiple inheritance through interfaces only. Answer (1 of 9): A straight-forward answer would be no. Yes, multiple (any number of) classes can implement one interface. Multiple inheritance using classes is not supported in Java & leads to a very fa An interface may not declare instance data such as fields, auto-implemented properties, or property-like events. Stereotypes are defined with the class keyword, << and >>.. You can also define notes using note left of, note right of, note top of, note bottom of keywords.. You can also define a note on the last defined class using note left, note right, note top, note bottom.. A note can be also define alone with the note keywords, then linked to other objects using the .. symbol. This shows that a class implementing an interface need not: 9. Where an Object ( class or Interface) acquires the property ( Methods, Variables, etc) of another object. (A) 1, 3 and 4 (B) 1, 2 and 4 (C) 2, 3 and 4 (D) 1, 2, 3 and 4 Answer: (A) Explanation: The instance of an interface cant be created because it acts as an abstract class. Interfaces. Share An interface in Java can contain abstract methods and static constants. public interface ICake where T : class { decimal GetPrice (); } So, we can implement as much as we want. Each interface contains a method declaration. .public, static, final fields (i.e., constants) .default and static methods with bodies 2) An instance of interface can be created. Inheritance And Implementation. The signature of the interface method and the same return type or subtype should be maintained when overriding the methods. Well go over how to implement multiple interfaces and scenarios where its appropriate to do so. In Find out whether interfaces are inherited: 10. You could create one class per interface and that would be To declare a class that implements an interface, you include an implements clause in the class declaration. It should be based on the requirements whether you want to go for abstract class or interface.if you are providing common functionality to differen Copy Code. Example class-implementing-interface.ts Interface only defines the common methods that should be included in the Implementation classes. 4) Many classes can implement the same interface. 0. When implementation interfaces, there are several rules . It only contains the method signatures, but the body of each method is empty. 2) C# does not support "multiple inheritance" (a class can only inherit from one base class). A class can implement multiple interfaces. Access Modifiers When an abstract data type is represented just as a single class, without an interface, its harder to have multiple representations. This program shows how we can create multiple methods in a class and how we can call one method from another method. Here we have create a Student class with member variables name, english, telugu, hindi, maths, science and social. In this example, we created 3 interfaces and then implemented them by using a class. Java supports multiple inheritance through interfaces only. Metadata tables typically can have no more than 2^24 members, so the total number of interfaces implemented by all types in an assembly must be less than about 16 million. This is the simple mathematical operation program demonstrating how multiple inheritance can be achieved in C# using Interface Concept. We can achieve multiple inheritances by the use of interfaces. Multiple inheritance is not supported because it leads to deadly diamond problem. Interface Usage Example: 6. So, we can implement as much as we want. Multi Super Interfaces: 8. Simply put, in Java, a class can inherit another class and multiple interfaces, while an interface can inherit other interfaces. Interface Collision: 4. interface Icalculate{ //interface The Box class implements two interfaces IEnglishDimensions and 12) A class can implement any number of interfaces. An interface cannot implement another interface in Java.
3) A class can implement multiple interfaces. Answer (1 of 4): There is a misconception implicit in the question. Beginning with C# 8.0, an interface may define a default implementation for members. A class or struct that implements an interface shall adhere to its contract. You can declare abstract methods in Yes, multiple (any number of) classes can implement one interface. Multiple inheritance using classes is not supported in Java & leads to a very famous problem known as Diamond problem where as it is supported using interfaces. , Oops ! Yes multiple classes can implement one interface irrespective of being in the same program or different. A class can implement any number of interfaces but can extend only one class. // This method gets called by the runtime. While working with the interface, make sure the class implements all its abstract methods. Allows 2 incompatible interfaces to work together. See the example below where we implemented all the methods of all 3 interfaces. All methods in the interface are implicitly public and abstract. Does Java support multiple inheritance through interface? In TypeScript, a class can implement interfaces to enforce particular contracts (similar to languages like Java and C#). This problem has been solved! Obviously you are going to never run into these limitations in practice. While working with the interface, make sure the class implements all its abstract methods.
As you can see I have implemented the Comparable interface in my Author class because I want to sort the objects of this class. a. forces classes that implement it to declare all the abstract interface methods. To declare a class that implements an interface, you include an implements clause in the class declaration. Java allows a class to implement multiple interfaces. We use the interface keyword to create an interface in Java. c) can extend any number of classes but can implement at most one interface.
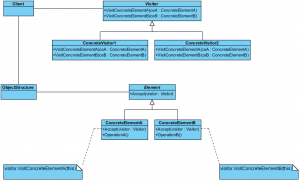
This can be achieved using inheritance by either inheriting from a superclass (base class), or by implementing one or many interfaces (as @Oded said, many small interfaces instead of one big interface). By convention, the implements clause follows the extends clause, if there is one.. A Sample Interface, Relatable An interface is like an Apex class in which none of the methods have been implemented. In Line 18, you can see that the Square class will implement the two interfaces. Here is a work around and we can handle such situations with little bit decorating our interface like this: Now our interface will look like this: C#. Yes, a class can implement two two interfaces with the same method signature but that method should be implemented only once in the class.

3. It therefore cannot inherit from a class. Which of the following is true about interfaces in java. Interfaces A and B both declare functions foo() and bar().Both of them implement foo(), but only B implements bar() (bar() is not marked as abstract in A, because this is the default for interfaces if the function has no body).Now, if you derive a concrete class C from A, you have to override bar() and provide an implementation.. is it possible? calculate(operand1:number,operand2:number):number When an Abstract Class Implements an Interface. In Java, an interface is not a class. Interface implementation is encoded in metadata in the InterfaceImpl table. is checks a given object implements or extends interface or class, It checks parent class hierarchy and returns true if it follows inheritance. runtimeType: Returns the class of an object and gives the only current class of an object not the inheritance tree class checking if an object implements interface in dart? Your class can implement more than one interface, so the implements keyword is followed by a comma-separated list of the interfaces implemented by the class. b) can extend any number of classes and can implement any number of interfaces. See the answer See the answer done loading. 3) A class can implement multiple interfaces. In Java, interfaces are declared using the interface keyword. An abstract class can inherit a class and multiple interfaces. It just defined the contract implementing by concrete classes. I would answer that whether you need an interface or not does not depend on how many classes will implement it. To use an interface, another class must implement it by providing a body for all of the methods contained in the interface. An interface defines a contract. Interface Usage Example: 7. For most intents and purposes you don't need to implement an interface on the derived class. An interface contains definitions for a group of related functionalities that a non-abstract class or a struct must implement. Now we are back to ConfigureServices method from the beginning of this article where for the same interface we register multiple class types. An interface or abstract class is the same. An interface may define static methods, which must have an implementation. Unlike classes, interfaces are always completely abstract. Use of interfaces helps a system stay decoupled and thus easier to refactor, change, and redeploy. An interface is a fully abstract class. An interface in Java is essentially a special kind of class. 1) To achieve security - hide certain details and only show the important details of an object (interface). In the Create new project window, select ASP.NET Core Web Application from the list of templates displayed. When one or more classes use the same interface, it is referred to as "polymorphism". 4) Many classes can implement the same interface. Program: The source code to implement multiple interfaces in the same class is given below. Interfaces make it easy to use a variety of different classes in the same way. Youre actually describing an abstract base class or ABC. Many OOP languages allow you to create a class with code in it, but mark one or more me extends keyword is used to inherit a class; while implements keyword is used to inherit the interfaces. A class can extend only one class; but can implement any number of interfaces. A subclass that extends a superclass may override some of the methods from superclass. A class must implement all the methods from interfaces. Any class can work together as long as the Adapter solves the issue that all classes must implement every method defined by the shared interface. Interface (If required): A class may implement any interface if required. 4) Many classes can implement the same interface.
It is possible, however, to define a class that does not implement all of the interface's methods, provided that the class is declared to be abstract. A Java interface contains static constants and abstract methods. To use interface in the java code, implements keyword is used.
Interfaces summary. Multiple inheritance using interface in java. A class can implement more than one interface at a time. December 14, 2018. A Java class. (So altogether, N + This shows that a class implementing an interface need not: 9. Another advantage is that multiple different representations of the abstract data type can coexist in the same program, as different classes implementing the interface. Interface Usage Example: 6. Does Java support multiple inheritance through interface? The interface in Java is a mechanism to achieve abstraction.There can be only abstract methods in the Java interface, not the method body. An interface in Java is defined as an abstract type that specifies class behavior. There is no such restriction. Because both are included, all members of both interfaces must be implemented by the Square class. Explicit interface implementation also allows the programmer to implement two interfaces that have the same member names and give each interface member a separate implementation. All methods in an interface are abstract, so they cannot be implemented in code and the abstract keyword is not necessary. When it comes to building applications with a graphical user interface (GUI), inheritance is arguably the most important mechanism for making it possible to quickly build an application. As much as it looked wrong in the first place, .NET Core dependency injection container can handle this king of interface implementation registration. 3. Answer (1 of 11): The short answer is NO. Can I avoid the above @Override and just use the classes without these Which of the following is true about interfaces in java. If you notice that many classes belong to a parent class with the same method actions but different behavior, then it's a good idea to use an interface. This shows that a class implementing an interface need not: 9. Find out whether interfaces are inherited: 10. public, static, final fields (i.e., constants) default and static methods with bodies 2) An instance of interface can be created. I would say that the limit should be a smaller number than 23 - more like 5 or 7, however, this does not include any number of interfaces that those interfaces inherit or any number of interfaces implemented by base classes. Share Improve this answer answered Nov 27, 2017 at 6:37 Keval 1,837 15 26 An Interface in Java programming language is defined as an abstract type used to specify the behavior of a class. I have written the logic of sorting in the compareTo() method, you can write logic based on the requirement. Question 1 options: a) can extend at most one class but can implement any number of interfaces. The preceding output creates more confusion about which method of which interface is implemented. (A) An interface cannot implement any non-default instance methods, whereas an abstract class can. c. is declared in a file by itself and is saved in a file with the same name as the interface followed by the .java extension. Any attempt to access old contracts would give a Java supports multiple inheritance through interfaces only. Answer (1 of 9): A straight-forward answer would be no. Yes, multiple (any number of) classes can implement one interface. Multiple inheritance using classes is not supported in Java & leads to a very fa An interface may not declare instance data such as fields, auto-implemented properties, or property-like events. Stereotypes are defined with the class keyword, << and >>.. You can also define notes using note left of, note right of, note top of, note bottom of keywords.. You can also define a note on the last defined class using note left, note right, note top, note bottom.. A note can be also define alone with the note keywords, then linked to other objects using the .. symbol. This shows that a class implementing an interface need not: 9. Where an Object ( class or Interface) acquires the property ( Methods, Variables, etc) of another object. (A) 1, 3 and 4 (B) 1, 2 and 4 (C) 2, 3 and 4 (D) 1, 2, 3 and 4 Answer: (A) Explanation: The instance of an interface cant be created because it acts as an abstract class. Interfaces. Share An interface in Java can contain abstract methods and static constants. public interface ICake