The point of variable names is to make reading code easier for the maintainer.Default values are a detail of the language specification (or sometimes even of the implementation) and may or may not match with your intent in using a Consider the following example. ON / OFF. What is the default value of a boolean in Java? public class Test { boolean booleanField; public static void main (String args[]) { System.out.println(new Test ().booleanField); // false} }
Note however that boolean b = false; will lead to two write operations: b will first be assigned its default value false then it will be assigned its initial value (which happens to be false as well). Very often, in programming, you will need a data type that can only have one of two values, like. The default value for a boolean is false. Boolean. Boolean Values. Example: Boolean.parseBoolean ("True") returns true. Here, we say simulate because unlike certain other OOP languages (like C++ and Scala), the Java specification doesn't support assigning a default value to a method parameter. 8 bytes. It returns false, if the Boolean value b is false ; It returns false, if the String The default value of a boolean in Java is false. Example 1. Example: boolean myBool = true; //Declaration of variable myBool that has value true boolean myBool1; //Default declaration, so myBool1 has default value i.e false Boolean expression. That means a boolean array is created with zero values in it. In Java, Boolean is a wrapper class that wraps the primitive datatype boolean type. Note: Every primitive has a wrapper class. default value of primitive boolean array is: false. Return Value: The valueOf() method returns a Boolean instance corresponding to 'b' or to String 's'. A Character [] using null as the "not set" value instead of '\u0000' (which is, after all, still a character)A MapSticking with char [] if you know you'll never, ever, ever want to consider an element with value '\u0000' as "set" Primitive Data Types. The default value of the primitive is a false value. March 20, 2022 + The default value of a boolean in Java is false. For boolean type, the default value is false, for float and double types default values are 0.0 and for remaining primitive types default value is 0.
Above program clearly depicts that for unassigned int data type variables in java, compiler assigns default value 0. Now, here default values are values assigned by the compiler to the variables which are declared but not initialized or given a value. For reference types (anything that holds an object in it) will have null as the default value. Above, firstly we have declared variables of specific type. public class Test { boolean booleanField; public static void main(String args []) { System.out.println ( new Test ().booleanField); // false } } The program with multiple unassigned boolean variable is given below: private boolean include = true; Java oriented plus Builder pattern Answer: Boolean is a primitive data type that takes either true or false values. int a = 5; int b = 3. Boolean values in Java. Something to consider: I suggest that the default value usually be false. The point of variable names is to make reading code easier for the maintainer.Default values are a detail of the language specification (or sometimes even of the implementation) and may or may not match with your intent in using a The default value for a boolean ( primitive ) is false . 2. String array default values: null null null null null Integer array default values: 0 0 0 0 0 Double array default values: 0.0 0.0 0.0 0.0 0.0 Boolean array default values: false false false false false Reference Array default values: null null null null null. This may mean that the name of the parameter gets switched. For type boolean, the default value is false. Checking some conditions such as a==b or ab can be considered as boolean examples. When we are creating a new array, the elements in the array will automatically be initialized by their default values. We can use the Arrays.fill() method in such cases. Following are the examples: Boolean - false. default value of Boolean object array is: null. Remember, to get the default values, you do not need to assign values to the variable. Example: default boolean value java Default values for variable types: Object = null int, double, long, (etc) = 0 boolean = false char = '\u0000' 1 byte. The default value of any Object, such as Boolean, is null. Java program to find the default value of a boolean array. Secondly, the boolean array is created with crurly braces with values inside it. A Boolean data type can only have a value of either true of false . The boolean returned represents the value true if the string argument is not null and is equal, ignoring case, to the string "true". Its size is 1-bit. You only have two options with you regarding the values of a Boolean type variable in java. Tinyint (1) field type for boolean data in MySQL table . When you extend an interface that contains a default method, you can do the following:Not mention the default method at all, which lets your extended interface inherit the default method.Redeclare the default method, which makes it abstract.Redefine the default method, which overrides it. Output. 1 bit.
Return Value : This method returns the primitive Boolean value of this Boolean object. 1. So your two statements are functionally equivalent in a single-threaded application . Java provides a richer set of primitive or basic or built-in data types than other languages like C and C++. equals method of Set returns true if the two sets have the same size and two 1 byte. Default value of boolean: false. Creating boolean Array. The default value of a boolean in Java is false. The boolean values can be declared with the boolean keyword. They are supported for primitive data types and the result of a comparison is a boolean value true or false. The default value of boolean is false.
either true or false.. Here, we say simulate because unlike certain other OOP languages (like C++ and Scala), the Java specification doesn't support assigning a default value to a method parameter. The wrapper data type (Boolean) works as a pointer and thus is initialized to null. So anything that returns the value true' or false can be considered as a boolean example. System.out.print (val + " "); } } Output. We can store, update or delete Boolean data by using Tinyint (1) field type. That way the behavior is "as expected in JS": a missing parameter is falsy. Here it comes my workaround: Non database portable solution @Column(columnDefinition="tinyint(1) default 1") private boolean include; Java oriented solution. To make the default value true, we need to use the Arrays.fill () method. In Java , the Boolean data type is a primitive data type, which means it comes packaged with the programming language. SELECT 1 IS TRUE, 0 IS FALSE , NULL IS UNKNOWN. As mentioned above, this data type should never be used for precise values, such as currency. default value of Boolean object array is: null. Lets be sure about it. Default value of boolean isValid: false . Char. 1. Any java expression that yields a boolean value is called a Boolean expression. Use this data type for simple flags that track true/false conditions. I am having an arraylist which is: Java Code: private List selectedCars = new ArrayList (); mh_sh_highlight_all (' java '); And one more for the carColors:. Returns the value of this Boolean object as a boolean primitive. boolean: The boolean data type has only two possible values: true and false. Boolean data can take values TRUE or FALSE or UNKNOWN. Double. Object - null. For primitive types like int , long , float the default value are zero ( 0 or 0.0 ). Output of the above program makes it clear that default value of boolean in java is false. Default value of Boolean (Wrapper): null. As far as i known there is no JPA native solution to provide default values. @Value("Default DBConfiguration") private String defaultName; @Value annotation argument can be a string only, but spring tries to convert it to the specified type. For this, JavaScript has a Bool The default value for a Boolean (object) is null. 2. This wrapper is added in the documentation in Java version 8. how to use boolean in java , its default value and boolean array with example programs. TRUE / FALSE. Value to a Boolean type is either true or false. The default value of the boolean elements in a Java boolean array is false . Boolean . As you can see default value of boolean is false and Boolean is null. March 20, 2022 + The default value of a boolean in Java is false. No, you absolutely should not choose variable names to conform to your language's default value of boolean in java. Overview. Double. However, there are cases where a default value for a boolean makes sense. However, it is more common to return boolean values from boolean expressions, for conditional testing (see below). The default value for a Boolean ( object ) is null . Example. What are the default values of boolean (primitive) and Boolean (primitive wrapper) in Java?. The boolean array can be created with empty curly braces. What is a Boolean value in Java? As an example, let's make some tea! Spring @Value Default Value. private boolean include = true; Java oriented plus Builder pattern We can coerce any expression in JavaScript to a boolean in one of 3 ways:Using the Boolean () wrapper function.Putting two exclamation points !! (the logical NOT ! operator, twice) in front of any expression, which calls the Boolean () wrapper function.Putting any expression inside of a conditional, such as an if statement, question mark ? operator, or loop with or without AND && or OR ||. Char. 2. The default value of a boolean in Java is false. JavabooleanBoolean() public class DefaultBooleanArrayValue { boolean [] primitiveBooleanArray = new boolean [3]; Boolean [] objectBooleanArray = new Boolean [3]; int length = primitiveBooleanArray.length; public static void main (String args []) { DefaultBooleanArrayValue obj = new DefaultBooleanArrayValue ();
8 bytes. In this quick tutorial, we'll investigate how can we provide default values for attributes when using the builder pattern with Lombok. In this case feel free to introduce a new enum and use the right value instead of the boolean. For boolean. The default value for a boolean (primitive) is false.. 5 please add official source link ; The default value of any Object, such as Boolean, is null.. replace () Parameterskey - key whose mapping is to be replacedoldValue (optional)- value to be replaced in the mappingnewValue - oldValue is replaced with this value For this purpose, Java provides a special data type, i.e., boolean, which can take the values true or false. We can assign default value to a class property using @Value annotation. To approach this problem. int - 0. double - 0.0. 1 bit. It returns a negative integer value -1, if x is false and y is true. static boolean val1; static double val2; static float val3; static int val4; static long val5; static String val6; Here it comes my workaround: Non database portable solution @Column(columnDefinition="tinyint(1) default 1") private boolean include; Java oriented solution. Lets verify it with another example program. Java boolean array is used to store boolean data type values only . What is the default value of a boolean in Java? In the above program, we have declared a variable with different datatypes. Default values.. Val1 = false Val2 = 0.0 Val3 = 0.0 Val4 = 0 Val5 = 0 Val6 = null. Java 8 Object Oriented Programming Programming. Java boolean keyword is used to declare a variable as a boolean type which represents only one of two possible values i.e. When an array is created without assigning it any elements, compiler assigns them the default value. Below are the default assigned values. In this short tutorial, we'll demonstrate the use of method overloading to simulate default parameters in Java. The resultant output of the program above is shown below. 1. To set a default value for primitive types such as boolean and int, we use the literal value: @Value("${some.key:true}") private boolean booleanWithDefaultValue; @Value("${some.key:42}") private int intWithDefaultValue; If we wanted to, we could use primitive wrappers instead by changing the types to Boolean and Integer. public class Test { boolean booleanField; public static void main (String args[]) { System.out.println(new Test ().booleanField); // false} }. As far as i known there is no JPA native solution to provide default values. The boolean array can be used to store boolean datatype values only and the default value of the boolean array is false.An array of booleans are initialized to false and arrays of reference types are initialized to null.In some cases, we need to initialize all values of the boolean array with true or false. Keyword Boolean with Variable Names. In this article, we will discuss what is Array default value in java. 4. Default value of int num: 0. vs. Boolean (Java Platform SE 7 ) Boolean has default init value of null. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type. Make sure to check out our intro to Lombok as well. 1. Parameters: NA. When we havent initialized the instance variables compiler initializes them with default values. Dependencies.
After construction of array, a new array of in heap memory according to the type of array.
For decimal values, this data type is generally the default choice. Every wrapper uses a reference which has a default of null. If you choose this option, you have to use dynamic-insert, so Hibernate doesnt include columns with null values. The default value for a boolean is false.. For example once I have had a specification which told me that I have to create a me And one more for the carColors:. Define your @Column annotation like that: @ Column (name = IS_ACTIVE, columnDefinition = boolean default true) Use columnDefinition to define default value. The result is true if and only if the argument is not null and is a Boolean object that represents a float with the same value as the float represented by this object. It returns true, if the defined Boolean value (b) or String value(s) is true. In this short tutorial, we'll demonstrate the use of method overloading to simulate default parameters in Java . Displaying initial values boolean (Initial Value) = false byte (Initial Value) = 0 short (Initial Value) = 0 int (Initial Value) = 0 long (Initial Value) = 0 float (Initial Value) = 0.0 double (Initial Value) = 0.0. All primitive or basic data types hold numeric data that is directly understood by system. To store Boolean data, MySQL uses Tinyint (1) field type. YES / NO. Arrays boolean has default init value of false. TLDR; The primitive data type (boolean) can only be true or false. We will now create few more unassigned boolean variables and try to print java boolean default value and see the output. Example: Boolean.parseBoolean ("yes") returns false. No, you absolutely should not choose variable names to conform to your language's default value of boolean in java. Note: Every primitive has a wrapper class. Pair Class Methods in Java. boolean isJavaFun = true; boolean isFishTasty = false; System.out.println(isJavaFun); // Outputs true System.out.println(isFishTasty); // Outputs false. Overview. In programming, we generally need to implement values that can only have one of two values, either true or false. Try it Yourself . No matter how many such variables we add and print their value the result will always be 0. @Builder.Default private boolean columnName = false; or @NotNull @Builder.Default @ColumnDefault("true") private Boolean columnName = true; @Builder.Default is to ensure we are having default values while constructing the object for this model, needed only when the entity model class is annotated with @Builder. There are eight built-in types supported by Java to support integer, floating-point, character, and boolean values. That's all for today, please mention in the comments in case you have any questions related to default value of Thats all about Java boolean default value.
Note however that boolean b = false; will lead to two write operations: b will first be assigned its default value false then it will be assigned its initial value (which happens to be false as well). Very often, in programming, you will need a data type that can only have one of two values, like. The default value for a boolean is false. Boolean. Boolean Values. Example: Boolean.parseBoolean ("True") returns true. Here, we say simulate because unlike certain other OOP languages (like C++ and Scala), the Java specification doesn't support assigning a default value to a method parameter. 8 bytes. It returns false, if the Boolean value b is false ; It returns false, if the String The default value of a boolean in Java is false. Example 1. Example: boolean myBool = true; //Declaration of variable myBool that has value true boolean myBool1; //Default declaration, so myBool1 has default value i.e false Boolean expression. That means a boolean array is created with zero values in it. In Java, Boolean is a wrapper class that wraps the primitive datatype boolean type. Note: Every primitive has a wrapper class. default value of primitive boolean array is: false. Return Value: The valueOf() method returns a Boolean instance corresponding to 'b' or to String 's'. A Character [] using null as the "not set" value instead of '\u0000' (which is, after all, still a character)A Map
Above program clearly depicts that for unassigned int data type variables in java, compiler assigns default value 0. Now, here default values are values assigned by the compiler to the variables which are declared but not initialized or given a value. For reference types (anything that holds an object in it) will have null as the default value. Above, firstly we have declared variables of specific type. public class Test { boolean booleanField; public static void main(String args []) { System.out.println ( new Test ().booleanField); // false } } The program with multiple unassigned boolean variable is given below: private boolean include = true; Java oriented plus Builder pattern Answer: Boolean is a primitive data type that takes either true or false values. int a = 5; int b = 3. Boolean values in Java. Something to consider: I suggest that the default value usually be false. The point of variable names is to make reading code easier for the maintainer.Default values are a detail of the language specification (or sometimes even of the implementation) and may or may not match with your intent in using a The default value for a boolean ( primitive ) is false . 2. String array default values: null null null null null Integer array default values: 0 0 0 0 0 Double array default values: 0.0 0.0 0.0 0.0 0.0 Boolean array default values: false false false false false Reference Array default values: null null null null null. This may mean that the name of the parameter gets switched. For type boolean, the default value is false. Checking some conditions such as a==b or ab can be considered as boolean examples. When we are creating a new array, the elements in the array will automatically be initialized by their default values. We can use the Arrays.fill() method in such cases. Following are the examples: Boolean - false. default value of Boolean object array is: null. Remember, to get the default values, you do not need to assign values to the variable. Example: default boolean value java Default values for variable types: Object = null int, double, long, (etc) = 0 boolean = false char = '\u0000' 1 byte. The default value of any Object, such as Boolean, is null. Java program to find the default value of a boolean array. Secondly, the boolean array is created with crurly braces with values inside it. A Boolean data type can only have a value of either true of false . The boolean returned represents the value true if the string argument is not null and is equal, ignoring case, to the string "true". Its size is 1-bit. You only have two options with you regarding the values of a Boolean type variable in java. Tinyint (1) field type for boolean data in MySQL table . When you extend an interface that contains a default method, you can do the following:Not mention the default method at all, which lets your extended interface inherit the default method.Redeclare the default method, which makes it abstract.Redefine the default method, which overrides it. Output. 1 bit.
Return Value : This method returns the primitive Boolean value of this Boolean object. 1. So your two statements are functionally equivalent in a single-threaded application . Java provides a richer set of primitive or basic or built-in data types than other languages like C and C++. equals method of Set returns true if the two sets have the same size and two 1 byte. Default value of boolean: false. Creating boolean Array. The default value of a boolean in Java is false. The boolean values can be declared with the boolean keyword. They are supported for primitive data types and the result of a comparison is a boolean value true or false. The default value of boolean is false.
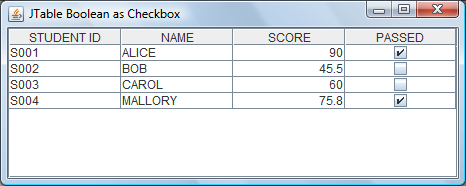
8 bytes. In this quick tutorial, we'll investigate how can we provide default values for attributes when using the builder pattern with Lombok. In this case feel free to introduce a new enum and use the right value instead of the boolean. For boolean. The default value for a boolean (primitive) is false.. 5 please add official source link ; The default value of any Object, such as Boolean, is null.. replace () Parameterskey - key whose mapping is to be replacedoldValue (optional)- value to be replaced in the mappingnewValue - oldValue is replaced with this value For this purpose, Java provides a special data type, i.e., boolean, which can take the values true or false. We can assign default value to a class property using @Value annotation. To approach this problem. int - 0. double - 0.0. 1 bit. It returns a negative integer value -1, if x is false and y is true. static boolean val1; static double val2; static float val3; static int val4; static long val5; static String val6; Here it comes my workaround: Non database portable solution @Column(columnDefinition="tinyint(1) default 1") private boolean include; Java oriented solution. Lets verify it with another example program. Java boolean array is used to store boolean data type values only . What is the default value of a boolean in Java? In the above program, we have declared a variable with different datatypes. Default values.. Val1 = false Val2 = 0.0 Val3 = 0.0 Val4 = 0 Val5 = 0 Val6 = null. Java 8 Object Oriented Programming Programming. Java boolean keyword is used to declare a variable as a boolean type which represents only one of two possible values i.e. When an array is created without assigning it any elements, compiler assigns them the default value. Below are the default assigned values. In this short tutorial, we'll demonstrate the use of method overloading to simulate default parameters in Java. The resultant output of the program above is shown below. 1. To set a default value for primitive types such as boolean and int, we use the literal value: @Value("${some.key:true}") private boolean booleanWithDefaultValue; @Value("${some.key:42}") private int intWithDefaultValue; If we wanted to, we could use primitive wrappers instead by changing the types to Boolean and Integer. public class Test { boolean booleanField; public static void main (String args[]) { System.out.println(new Test ().booleanField); // false} }. As far as i known there is no JPA native solution to provide default values. The boolean array can be used to store boolean datatype values only and the default value of the boolean array is false.An array of booleans are initialized to false and arrays of reference types are initialized to null.In some cases, we need to initialize all values of the boolean array with true or false. Keyword Boolean with Variable Names. In this article, we will discuss what is Array default value in java. 4. Default value of int num: 0. vs. Boolean (Java Platform SE 7 ) Boolean has default init value of null. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type. Make sure to check out our intro to Lombok as well. 1. Parameters: NA. When we havent initialized the instance variables compiler initializes them with default values. Dependencies.
After construction of array, a new array of in heap memory according to the type of array.
For decimal values, this data type is generally the default choice. Every wrapper uses a reference which has a default of null. If you choose this option, you have to use dynamic-insert, so Hibernate doesnt include columns with null values. The default value for a boolean is false.. For example once I have had a specification which told me that I have to create a me And one more for the carColors:. Define your @Column annotation like that: @ Column (name = IS_ACTIVE, columnDefinition = boolean default true) Use columnDefinition to define default value. The result is true if and only if the argument is not null and is a Boolean object that represents a float with the same value as the float represented by this object. It returns true, if the defined Boolean value (b) or String value(s) is true. In this short tutorial, we'll demonstrate the use of method overloading to simulate default parameters in Java . Displaying initial values boolean (Initial Value) = false byte (Initial Value) = 0 short (Initial Value) = 0 int (Initial Value) = 0 long (Initial Value) = 0 float (Initial Value) = 0.0 double (Initial Value) = 0.0. All primitive or basic data types hold numeric data that is directly understood by system. To store Boolean data, MySQL uses Tinyint (1) field type. YES / NO. Arrays boolean has default init value of false. TLDR; The primitive data type (boolean) can only be true or false. We will now create few more unassigned boolean variables and try to print java boolean default value and see the output. Example: Boolean.parseBoolean ("yes") returns false. No, you absolutely should not choose variable names to conform to your language's default value of boolean in java. Note: Every primitive has a wrapper class. Pair Class Methods in Java. boolean isJavaFun = true; boolean isFishTasty = false; System.out.println(isJavaFun); // Outputs true System.out.println(isFishTasty); // Outputs false. Overview. In programming, we generally need to implement values that can only have one of two values, either true or false. Try it Yourself . No matter how many such variables we add and print their value the result will always be 0. @Builder.Default private boolean columnName = false; or @NotNull @Builder.Default @ColumnDefault("true") private Boolean columnName = true; @Builder.Default is to ensure we are having default values while constructing the object for this model, needed only when the entity model class is annotated with @Builder. There are eight built-in types supported by Java to support integer, floating-point, character, and boolean values. That's all for today, please mention in the comments in case you have any questions related to default value of Thats all about Java boolean default value.