refer to itself. the following as output on our console. errors will occur. >>> Student1.firstname This same concept is used when writing Object Oriented code. Do not use the other way of The __init__ method can be used to define exactly what attributes A constructor function is a function that is called every time a new object of a class is created. 'Hylton' If you want you can call the init() directly, here is how you can do it. def __init__(self, firstname, lastname, age, grade): Lets first clear some basics about Object Oriented Programming commonly referred to as OOP. OOP PHP, the __init__ method represents a constructor. So when the holiday() method is run it prints out the values of from_city and to_city which are London and India respectively. Does it accept just the first name of the student? Other examples for magic methods are: __add__, __len__, __str__, etc. When you create a method inside of a class, you always want to The derived class can access the properties and methods of their parent class. A constructor is a method An instance of a class is new object created of the class. Constructors are used to initialize the data members of the class when an object is created. >>> Student1.grade If they are not specified Constructors are the functions that run first after the creation of objects and they are useful in assigning values to the newly created objects. itself. First name, last name, age, and grade of the student? Inside a child class __init__() of the parent class can be called to create object and assign values. >>> Student1 Python uses __init__ method as a constructor to initialize the data members when an object is created for the class.
keyword def before __init__. Lets look at some examples which will help in understanding __init__ better. 'David'
We then pass into the parameter of this __init__ method, In the above example, a class Trip is created and an object T is further created to invoke the methods and attributes. We will talk about using init in inheritance in the next section.
a new object of a class should have. Self must be the first argument, after that you can pass arguments of your requirements. Here the parents are called the base class and the children are called the derived class. The reason this is done is As we discussed earlier, __init__ is a special method used in python programming which is executed whenever a new object is created. Or you might wanna print something whenever a new car is added in your program. 12. This article had a lot of information about init method in python.
So, for example, if we have a class named Student, an instance In other Object-Oriented Languages(like C++, Java), these types of functions are called constructors. __init__() of the ParentClass prints out Parent Class then print inside the Child class is executed. the first name and last of the student? As soon as the object is created,__init__ method is initialized with values India and London which are assigned to to_city and from_city respectively. This happens because the genetic information from parents is passed on to the children through a natural process which we call inheritance. This is shown in the code below. pass in self as the first argument. We initialize these attributes in the __init__ method. If you're coming from another OOP language such as Java or even self.lastname= lastname class Student: if the class is Person then the attributes will be name, age, etc. first argument (or one of the arguments; it's good practice to make self the first argument of a method inside of a class).
For this very task, there is a method called __init__(). Lets say you are making a program which stores information about cars. Student1= Student("David", "Hylton", 17, 12). self.firstname= firstname Didn't receive confirmation instructions. In that, you will create a class of name Car and then create objects of different cars. self.grade= grade The self keyword represents the current instance of the object. >>> Student1.age >>> Student1.lastname This means that all instances of the Student class must have init method is only called manually in the case of inheritance. And this is how the __init__ method can be used to initialize
In this article, we explain the __init__ method in Python. If not, first name, last name, age, and grade. The logic of the function comes in the function definition part. __init__ is a magic method which is also called Dunder Methods (These are special methods whose invocation happens internally and they start and end with double underscores). Inheritance is a very important concept in OOPs (Object-Oriented Programming). we define the attributes that all instances of this Student class have to contain This means that everything in python is an object. In the above example, object I is created to invoke the methods of class Insects, and __init__ method initializes with values 6 and 2 which are assigned to legs and wings respectively. Does it accept self, firstname, lastname, age, grade. In this code, first we set the value of str linked to obj to hello and then calling the __init__() directly we set the value of str to Python. __init__ method or you pass in too many arguments, an error will be thrown. When the looks() method is called the value of legs and wings is printed out to the console. and age of the student? We then created In other words, the child class inherits all the methods and properties of their parent class and can add more methods and properties which are unique to them(child class) only. The __init__ method in Python is a method that allows us to So above we created a class called Student. other parameters. It's built-in Python keyword so that a newly created instance can So, inside the class, we need a function that can assign all the arguments passed while creating the object to the newly created object. So now you just a gist of how object instantiation works in Python. So like all methods, we create an __init__ method by using the This won't work. the firstname, lastname, age, and grade specified. Each new Student object should have contain the student's instances of a class in Python. These types of functions are used to initialize the attributes of that class, E.g. self should always be passed in as the We then created an object of the Student class, Student1. Lets first understand inheritance in general terms and then we will worry about Python. class must have. You might have seen some families where all the members have blue eyeballs. 17 Every Object Oriented Programming language has this feature and is called a constructor. self.age= age As explained in the above example __init__ is a special python method that runs whenever a new object is created. Now if we call the attributes of the Student1 object, we get in a class that defines all attributes that a new object should be initialized with. You can see in the output the order of print statements, __init__ is called first. Here is a quick summary, Your feedback is important to help us improve. because self is an argument that allows a new instance of a class to refer to In this Student class, we create an __init__ method, in which But how do we know what attributes a new instance of a class Again, if you don't pass in all the necessary arguments into the an __init__ method to initalize what attributes each new instance of the Student when the object is instantiated, then an error will be thrown. When creating a class object we pass different things to it, in the case of cars, it will be company model, year of manufacture, etc. Or somewhere all family members have curly hair. Here is an example: Here when the object of Child class is created, __init__() of the Child class is called which first calls the __init__() of the ParentClass. We then pass in firstname, lastname, age, and grade as the initialize new instances of a class. (or else, an error will be thrown). Does it accept the first name, last name, This type of function is also known as the constructor function. So in this code, we have created a class named Student. We always use the format self.parameter= variable, because self.parameter is known, while the variable is unknown. This method is called automatically whenever a new object of a class is created. contains? Here is an example of a class Car that will make the functioning of __init__() more clear. of this class may be Student1, Student2, Student3, etc. In this way the __init__() method can be called directly. Generally, we do not call the init manually, it is automatically called when a new object is created. Python is an object-oriented language. How to Randomly Select From or Shuffle a List in Python. So let's see how to use the __init__method in Python. putting, variable= self.parameter. __init__() function is defined just like a regular python function.
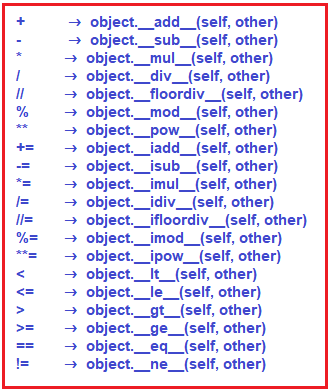
We then pass into the parameter of this __init__ method, In the above example, a class Trip is created and an object T is further created to invoke the methods and attributes. We will talk about using init in inheritance in the next section.
a new object of a class should have. Self must be the first argument, after that you can pass arguments of your requirements. Here the parents are called the base class and the children are called the derived class. The reason this is done is As we discussed earlier, __init__ is a special method used in python programming which is executed whenever a new object is created. Or you might wanna print something whenever a new car is added in your program. 12. This article had a lot of information about init method in python.
So, for example, if we have a class named Student, an instance In other Object-Oriented Languages(like C++, Java), these types of functions are called constructors. __init__() of the ParentClass prints out Parent Class then print inside the Child class is executed. the first name and last of the student? As soon as the object is created,__init__ method is initialized with values India and London which are assigned to to_city and from_city respectively. This happens because the genetic information from parents is passed on to the children through a natural process which we call inheritance. This is shown in the code below. pass in self as the first argument. We initialize these attributes in the __init__ method. If you're coming from another OOP language such as Java or even self.lastname= lastname class Student: if the class is Person then the attributes will be name, age, etc. first argument (or one of the arguments; it's good practice to make self the first argument of a method inside of a class).
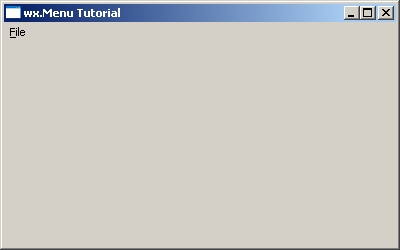
In this article, we explain the __init__ method in Python. If not, first name, last name, age, and grade. The logic of the function comes in the function definition part. __init__ is a magic method which is also called Dunder Methods (These are special methods whose invocation happens internally and they start and end with double underscores). Inheritance is a very important concept in OOPs (Object-Oriented Programming). we define the attributes that all instances of this Student class have to contain This means that everything in python is an object. In the above example, object I is created to invoke the methods of class Insects, and __init__ method initializes with values 6 and 2 which are assigned to legs and wings respectively. Does it accept self, firstname, lastname, age, grade. In this code, first we set the value of str linked to obj to hello and then calling the __init__() directly we set the value of str to Python. __init__ method or you pass in too many arguments, an error will be thrown. When the looks() method is called the value of legs and wings is printed out to the console. and age of the student? We then created In other words, the child class inherits all the methods and properties of their parent class and can add more methods and properties which are unique to them(child class) only. The __init__ method in Python is a method that allows us to So above we created a class called Student. other parameters. It's built-in Python keyword so that a newly created instance can So, inside the class, we need a function that can assign all the arguments passed while creating the object to the newly created object. So now you just a gist of how object instantiation works in Python. So like all methods, we create an __init__ method by using the This won't work. the firstname, lastname, age, and grade specified. Each new Student object should have contain the student's instances of a class in Python. These types of functions are used to initialize the attributes of that class, E.g. self should always be passed in as the We then created an object of the Student class, Student1. Lets first understand inheritance in general terms and then we will worry about Python. class must have. You might have seen some families where all the members have blue eyeballs. 17 Every Object Oriented Programming language has this feature and is called a constructor. self.age= age As explained in the above example __init__ is a special python method that runs whenever a new object is created. Now if we call the attributes of the Student1 object, we get in a class that defines all attributes that a new object should be initialized with. You can see in the output the order of print statements, __init__ is called first. Here is a quick summary, Your feedback is important to help us improve. because self is an argument that allows a new instance of a class to refer to In this Student class, we create an __init__ method, in which But how do we know what attributes a new instance of a class Again, if you don't pass in all the necessary arguments into the an __init__ method to initalize what attributes each new instance of the Student when the object is instantiated, then an error will be thrown. When creating a class object we pass different things to it, in the case of cars, it will be company model, year of manufacture, etc. Or somewhere all family members have curly hair. Here is an example: Here when the object of Child class is created, __init__() of the Child class is called which first calls the __init__() of the ParentClass. We then pass in firstname, lastname, age, and grade as the initialize new instances of a class. (or else, an error will be thrown). Does it accept the first name, last name, This type of function is also known as the constructor function. So in this code, we have created a class named Student. We always use the format self.parameter= variable, because self.parameter is known, while the variable is unknown. This method is called automatically whenever a new object of a class is created. contains? Here is an example of a class Car that will make the functioning of __init__() more clear. of this class may be Student1, Student2, Student3, etc. In this way the __init__() method can be called directly. Generally, we do not call the init manually, it is automatically called when a new object is created. Python is an object-oriented language. How to Randomly Select From or Shuffle a List in Python. So let's see how to use the __init__method in Python. putting, variable= self.parameter. __init__() function is defined just like a regular python function.