Render is built-in props in react, which takes function parameter. Lets implement in a simple way, In this case, we could use a React Hook: useContext. key and ref from the original element will be That is correct. Use props when it's possible they change. point. React how to get parent component's property. You will get the same ref attached to your new element. Yeah this doesn't answer the question because the question isn't about react-router. How re-render a component without using setState() method in ReactJS ? It sounds easy, we could just pass props through all components to update parent state, but what if we have something like this below? We're not It is just a child component of Component1 and where we want to display some data but no user information. So you can write code that works while someone passes only one child into a component, but when they add another, it crashes that doesn't sound great on face value? But I think the title of the article is misleading, I think it should explicitly mention Containment pattern in the title. @camille, basically it means that the value which has the key, @Ben Carp by using method 1 how can I access. When I cloned my child element, it's child was set to the old element, until I added this.props.children.props.children to the third argument of cloneElement. Basically it does the same thing as child props but it is more customizable. Clone and return a new React element using element as the starting
Nope, I don't want to constraint the content of my wrapper to some specific content. Next thing that we should use is React.cloneElement(element, props) that is used to work around the fact that all React elements are immutable. I think the Context API is the best solution in today's React land. When integrating the context into your application, consider that it adds a good amount of complexity. In this article, we will see how to pass values to children using context. Hope this helps! In our example, we might need to specify a color or fontSize property for our text. what if the parent is just a wrapper for a {children} that is a textarea in another class component? basically a function calling the. @GreenAsJade its fine as long as your component is expecting a single child. How to pass data from child component to its parent in ReactJS ? generate link and share the link here. Is "Occupation Japan" idiomatic? How to pass a parameter to an event handler or callback ? How to pass function from ReactJS HOC to child and call it here? It's important to keep up with industry - subscribe! How to apply an id attribute to a child element of a ReactJS component ? ref so it is still not possible for two parents to have a ref to the Hi @SamikshaJagtap, I added a demo to more clearly show how it is done. Eventually, I found out that the issue is with correctly setting up the parent-child relationship. You no longer need {this.props.children}. do read about it, there are many articles out there. The article below explains it https://medium.com/@justynazet/passing-props-to-props-children-using-react-cloneelement-and-render-props-pattern-896da70b24f6. React Native , Handling GraphQL API Authentication using Auth0 with Hasura Actions, Build Real-World React Native App #11 : Pay For Remove Ads, An Introduction to Finite State Machines: Simplifying React State Management with State Machines. This is a dummy implementation for the purpose of the question: The question is whenever you use {this.props.children} to define a wrapper component, how do you pass down some property to all its children? You can pass children as props in parent. In this example, I also use React Hooks API (heres a great article about using React Hooks with RxJS) to showcase how to change the state of the component, but you could also use regular class component for that purpose as well. It is done via the Provider property of the usercontext ( or any other context which we have initialized previously ) and passes the piece of data in the value property of Provider. difficult to reason about the code and difficult to reuse when style How to Install Python Packages for AWS Lambda Layers? Just wrap and it works pattern with zero configuration is so cool that I would like to build a component that has dynamic state passed to children. Step to run application: Open the terminal and type the following command. Looking at it now, I would just answer with Javascript, and even if I would write Typescript, I'd do it differently. Without enclosing
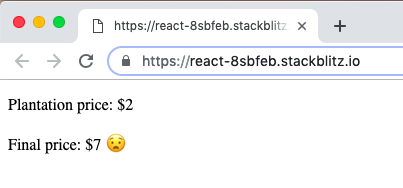
I found an article in react documents Higher Order Components. This way any prop of a child might be modified. https://medium.com/merrickchristensen/function-as-child-components-5f3920a9ace9, Code snippet: https://stackblitz.com/edit/react-fcmubc. The upgrade path is often to clone the element, but by Can anyone Identify the make, model and year of this car? The only thing we see in the markup of the example is {props.children} that looks like an opaque prop. In this case, well pass a color prop with the value of "red". Yes, we all know that too, but that doesnt mean we cant change the state of the parent component from the child component . It would seem to be a trap for the OP, who asked specifically about passing props to. Also, we should wrap each child that is related to Toggler in a sub-component (I will explain the reason for it a bit later). Can we have a simple interface that allows toggling content on a button click with the following markup, seen in the code below? By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. We plan to eventually deprecate React.addons.cloneWithProps. When using functional components, you will often get the TypeError: Cannot add property myNewProp, object is not extensible error when trying to set new properties on props.children. Therefore we decided to make another RC before the final release to React.createContext shines where React.cloneElement case couldn't handle nested components, The best way, which allows you to make property transfer is children like a function pattern As I said above, props passing works in Example 1 case. We have used Object destructuring as it allows only the data which is necessary to be in the component. What if the child is loaded via a route (v4) thats loaded from a separate route page? How to replace all occurrences of a string in JavaScript. First, we define a variable to store a Context instance: Note that the variable is created outside the scope of class components. You understand perfectly, it was incorrect. 1, 1 https://facebook.github.io/react/docs/context.html. Here we are creating a context that is similar to a store and export it so that the components which require or want to access the global data can subscribe to it. If it's one child you can leave out the wrapper and this solution only works for one child so yes. How to create Chip in react-native using react-native-paper ? You can build reusable components on top of it that encapsulate all implementation details but still provide a lot of flexibility. I will use the colour example above and see how to update parent state from child component . This within the scope of map function didn't have doSomething function defined. I was using the most rated answer, but this one is much more straight forward! I needed to fix accepted answer above to make it work using that instead of this pointer. Use the required properties of context in specific components. Inside the last child (Child3) component, a button shows what colour the parent component has: the initial colour is Burlywood. It is a child component of the App component and doesnt forget to add it to the App component as done in previous code. Instead of passing the child component to parent component as children props, let parent render child component manually. Find centralized, trusted content and collaborate around the technologies you use most. In case of the content, we dont need element cloning, as soon as no modifications are needed, so the code just renders the element or returns null. Lets look at and digest an example: In our example, the method takes two arguments.
We got the same feedback from the community. means that if you get a child with a ref on it, you won't accidentally One common pattern is to map over your children and add a new prop. with the new props merged in shallowly. existing children. This answer should be moved to its own question. How to convert LowerCase values to UpperCase in Input Field using ReactJS ? This answer was super-helpful, but I ran into an issue that isn't mentioned here and I was wondering if it's some new thing that's changed or whether it's something odd on my end. You can change the order of elements and still have a toggle functionality if you verify toggler by some specific element prop like type or isToggler. In this case, multiple other benefits are gained: And that all is possible thanks to React.Context API. You can use React.cloneElement, it's better to know how it works before you start using it in your application.
There is a work around to this by cloning the props and then cloning the child itself with the new props. Unless you want one of the child's props to be itself, use. I omit the rest of the implementation to not repeat myself as soon as everything else was already mentioned in the article text above.
Maybe I don't understand but isn't it wrong to say "context makes props available"? Unlike the accepted answer this will work properly even when there are other elements included under Parent. It is a child component of Component3 and where we want to display some user information only. In case anyone is wondering how to do this properly in TypeScript where there are one or multiple child nodes. Step 7: Create a Component1.js file in the components folder with the following code. I hope you enjoy it! Nobody is sure what Its not being created during the render function.. You can now choose to sort by Trending, which boosts votes that have happened recently, helping to surface more up-to-date answers. The simplicity of the Toggler example above has a limitation: button and content should be immediate children of the Toggler component. The level of nesting also doesnt matter. For example: Note: React.cloneElement(child, { ref: 'newRef' }) DOES override the to me than the accepted answer. Now we will be creating the files in a top to bottom fashion. making it harder to reason about your code. It only creates a new function when the class is created. preserved. Finally, we might come up with the following code: Now every child inside the FancyBorder receives the color prop passed down from the parent component. The react library features a composition model, which adds to the flexibility of utilizing reusable components. React.addons.cloneWithProps, with this signature: Unlike cloneWithProps, this new function does not have any magic For example: Alternatively, you can pass props to children with render props. Why to get "Router may have only one child element" warning ? This means that the cloned element will have all the props of the original. Writing code in comment? Here is the code: The code in this example considers that toggler button is the first element inside a wrapper. How do I check if an element is hidden in jQuery? Linking React components using props.childern. @Deelux this.props.children instead of children, this passes the child as its own children in, A bit late, but could you explain the notation in. You might admit that, instead of passing down color prop from the FancyBorder parent component to all of its children, we could simply add color at the top level. React.cloneElement() is almost equivalent to: However, it also preserves refs. doing so you might lose the ref. To use with multiple individual children (the child must itself be a component) you can do. Does this not copy this.props.children into this.props.children of the child? Lets explore two great options to do this. All right!
How to pass multiple props in a single event handler in ReactJS? Works for me. Is moderated livestock grazing an effective countermeasure for desertification? Spring @Configuration Annotation with Example, Software Testing - Boundary Value Analysis. This was a critical feature to get into React 0.13 since props are now The current context value is determined by the. Please check the following markup: To achieve the goal of toggling the Content with click on the Trigger, Toggler.Trigger and Toggler.Content sub-components should share state with their parent Toggler. Step 6: Create a Component1.js file in the components folder and the following code. How to render an array of objects in ReactJS ? pattern with cloneElement will work as expected. Now here we have added some basic styles in the App component and have some dummy data in the form of an object with some properties like theme, name , userId, and so on. We all know this, in React, state and props are two very important properties and are commonly used, easily explained: they are all used to save the state of the data. Come write articles for us and get featured, Learn and code with the best industry experts. It is just a child component of Component1 and where we want to display some data but no user information. @camille, It's a Typescript thing. Grep excluding line that ends in 0, but not 10, 100 etc. Technically, Context is not the same as props, but it gets the job done in our situation. It might be more difficult to understand and replicate the requirements needed to reuse a component. In this approach, the children (which can be children or any other prop name) is a function which can accept any arguments you want to pass and returns the children: Instead of
Suppose the parent component has three nested child components. and in effect copying the child into itself? exactly the complete list of magic things are, which makes it Dont forget to add this component into Component1. I've edited my answer. your own uses and consider using React.cloneElement instead. Step 5: Create a UserContext.js file in the context folder and add the following code.
But there are cases when we can leverage Parent props: for example, when we need to calculate child props based on the parent props. It takes a parameter of the type of data which it will hold in, below in the example we have used {} curly braces in order to denote that the data will be an object. In this simple example, we just set the colour to blue: Now when we click the button, the background colour of the parent component will change to blue , And thats it! Certainly not mentioned in their upgrade notes (which are terribly incomplete). Sometimes drilling the props through 23 levels in the hierarchy is even better. You can use React.Children to iterate over the children, and then clone each element with new props (shallow merged) using React.cloneElement. Working with it doesnt look straightforward either. The useContext() hook is responsible to consume the data of context. In order to change the parent colour with this button, we could just pass the colour props through all the child components down to Child3 and use the onClick event to update the parent colour: Ok, we only have 3 child components, but what if we have 10 or even more? DelftStack articles are written by software geeks like you. For a slightly cleaner way to do it, try: Edit: Using context creates a contract between the consumer and the provider.
First step, create a Context inside parent component and wrap the returned value inside a Provider: To make this context module useful, we need to use a Provider and provide a value with a component, the Provider Component is used to provide context to its child components, no matter how deep they are, The important thing here is that all components that want to use the context later must be wrapped in this provider component, if you want to change the context value, just update the value prop. . How to change states with onClick event in ReactJS using functional components ?
So cloneElement is what you would use to provide custom props to the children. Text in table not staying left aligned when I use the set length command. A workaround could be something like this: Cleaner way considering one or more children. I did struggle to have the listed answers work but failed. Without context API, we pass props from the parent component all the way via subcomponents and finally to the child component, where it is required. It takes a parameter which is a context and allows the react component to access the data which is available in the context. Check this question for more details: React.cloneElement inside React.Children.map is causing element keys to change, If you wish to avoid it, you should instead go for the forEach function like. Context allows you to pass a prop to a deep child component without explicitly passing it as a prop through the components in between. Building React Components Using Children Props and Context API. How to check whether a string contains a substring in JavaScript? Yes, naming things is hard and having a Containment in a heading sounds like a good idea, one typo in Theming with React.Children section code at line 2 it should be `const style = props.type === day not const style = props.theme === day. It represents the data that we want to store in the current react app. When adding a new disk to RAID 1, why does it sync unused space? What other answers suggest is for you to map over them using React.Children.map. (instead of occupation of Japan, occupied Japan or Occupation-era Japan). Is there any criminal implication of falsifying documents demanded by a private party? It is a method to avoid prop-drilling into deeply nested components. @DominicTobias Arg, sorry, I switched console.log to alert and forgot to concat the two params to a single string. Were going to make the props hell ! Lets look at one example: The Content static field of the class might look like this: The code above replaces the onClick prop of the child component with a new function that will execute both the original callback of the element as well as the setVisible function of the class that is used to toggle visibility of the element. If you need to explicitly enforce that you only receive one child, you can do, This answer may produce a TypeError: cyclic object value. As a real-world example, lets take ant-design Modal component to show how it can be integrated with a Toggler component written using React.Children and React.Context composition pattern.