And we can not access the real output when it is available using the Promise return by the function. it only knows how to handle URIError), so it throws it again: The execution jumps from the first .catch (*) to the next one (**) down the chain. Or, maybe, everything is all right with the site, but the response is not valid JSON. Promise is used to make asynchronous calls.
This results from the fact that a promise can be rejected by throwing inside then or catch (this is a preferable way to reject existing promise), and this cannot be handled by typing system; also, TypeScript also doesn't have exception-specific types except never. For instance, in the code below the URL to fetch is wrong (no such site) and .catch handles the error: As you can see, the .catch doesnt have to be immediate. html
jmeter The Promise will either use .then() if it gets resolved or .catch() if it gets rejected. firefox We can use it when handling numerous jobs at once. The fix is to add return type annotations to your functions and methods. github
But if any of the promises above rejects (a network problem or invalid json or whatever), then it would catch it. css
php
log parser studio
type represents the return value of a function that doesn't return anything.
The promise will reject. This also aids in improving the applications performance. cranked This happens not only in the executor function, but in its handlers as well. The first way to end up in a sticky puddle is to leave-off the return type annotation on the function that returns a promise. monitoring Yes, the promise interface says you can use anything as your rejection reason but your error-handling strategy says that youll use a simple hierarchy of semantic error types. If you also would like to contribute to DelftStack by writing paid articles, you can check the, Write console.log Wrapper for Angular 2 in TypeScript. When using it, you must pass the inner function; otherwise, youll get an error. Lets switch the resolve and reject parameters: Immediately, TypeScript warns us that the argument of type Error isnt either a string or a PromiseLike. The short answer to this is that TypeScript will catch this problem if you do things my way. All your promises will either resolve or reject, but due to the alliteration people sometimes use (reject, resolve) when they should be using (resolve, reject). linq If an error occurs, and theres no .catch, the unhandledrejection handler triggers, and gets the event object with the information about the error, so we can do something.
To lint for these cases, use the no-throw-literal rule.
Your best option is to use type guards to provide both a design-time and run-time check in your code. Did you forget to include 'void' in So how do we end up not being told about this problem by the compiler? Lets first see what Promises are and why they are being used. OpenJS Foundation and ESLint contributors.
And if we handle the error and finish normally, then it continues to the next closest successful .then handler. In the above example, the resolve() part of the Promise is called and returns a boolean value, whereas the reject() part is not. The first state is called the Pending state. Or even multiple ones, if you have a chain of promises: In this case, if thePromise is rejected, the execution jumps directly to the catch() method. In a regular try..catch we can analyze the error and maybe rethrow it if it cant be handled. vscode
not return anything. How does Keras ImageDataGenerator rescale parameter works? How to get value from LiveData synchronously? Multiple parallel calls are handled using Promise.
Selecting a version will take you to the chosen version of the ESLint docs. We declared an asp.net The easiest way to catch all errors is to append .catch to the end of chain: Normally, such .catch doesnt trigger at all. value of the Promise to void. So the promise cant handle it. java
Thus, this Promise will always be resolved.if(typeof ez_ad_units!='undefined'){ez_ad_units.push([[250,250],'delftstack_com-leader-1','ezslot_8',114,'0','0'])};if(typeof __ez_fad_position!='undefined'){__ez_fad_position('div-gpt-ad-delftstack_com-leader-1-0')}; And since the resolve() part of the Promise is being called, it will execute .then().
This tutorial will discuss returning a proper Promise in TypeScript with coding examples and outputs. The second is called the Resolved state, in which the Promise is successfully executed.
octopus Usually such errors are unrecoverable, so our best way out is to inform the user about the problem and probably report the incident to the server. What do you think?
type of the exception at design time, and neither TypeScript nor
This rule takes one optional object argument: Examples of incorrect code for this rule: Examples of correct code for this rule with the allowEmptyReject: true option: Due to the limits of static analysis, this rule cannot guarantee that you will only reject Promises with Error objects.
// Did you forget to include 'void' in your. You can see it in the console if you run the example above. ddd
Cause there is no way to set error type in some cases like Promise, or exception throws, we can work with errors in rust-like style: Catching specific error messages in try / except, Angular Material Custom Component Theming. The key advantage of using it is that we may move on to the following line of code without executing the last line. entityframework The JavaScript engine tracks such rejections and generates a global error in that case. operations Promise chains are great at error handling. Promises are fast becoming the de-facto pattern for async in TypeScript (and JavaScript) so being disciplined about return type annotations and rejection types gives you two more chances to win. estimation estimates What happens when an error is not handled? As explained in this issue, Promise doesn't have different types for fulfilled and rejected promises. Promise generic just like we did in the previous examples. The same thing is possible for promises.
reject accepts any argument that doesn't affect type of a promise. Error objects automatically store a stack trace, which can be used to debug an error by determining where it came from. We want to make this open-source project available for people all around the world. It may appear after one or maybe several .then. A single .catch() can be aggregated for the error handling of any preceding portion of the chain.
For more information on this caveat, see the similar limitations in the no-throw-literal rule. This rule was introduced in ESLint v3.14.0. // Error: Expected 1 arguments, but got 0. string.
Will the .catch trigger? Promises are one of the best things that happened to JavaScript in the past few years. It is a simple mistake to make but Im interested in it because I want to know why TypeScript didnt catch the mistake.
This rule aims to ensure that Promises are only rejected with Error objects. Error handling in my opinion is best in async/await, but sometimes we cant avoid using promises, so thats how you can do it. Oops.
javascript bdd Promise constructor. For example, a programming error: The final .catch not only catches explicit rejections, but also accidental errors in the handlers above. Keep in mind that you can only call from tasks that arent interdependent. When a promise rejects, the control jumps to the closest rejection handler. in the example. on 21st November 2017, #noestimates This rejects parameter will handle the error using the catch() block. How to ignore generated files from Go test coverage, Pandas how to find column contains a certain value, Recommended way to install multiple Python versions on Ubuntu 20.04, Build super fast web scraper with Python x100 than BeautifulSoup, How to convert a SQL query result to a Pandas DataFrame in Python, How to write a Pandas DataFrame to a .csv file in Python. If your Promise may get resolved with multiple types, use a As we already noticed, .catch at the end of the chain is similar to try..catch. Basically, it now thinks you are trying to return a promise that resolves with your error object. Help to translate the content of this tutorial to your language! web log importer, Web Ops Dashboards, Monitoring, and Alerting, Run a Bash Script with Arguments in GitHub Actions. If youre using custom non-error values as Promise rejection reasons, you can turn off this rule.
A promise will look something like this: Inside the promise we are passed 2 parameters, 2 functions. In the example below we see the other situation with .catch. Otherwise, there will be a data inconsistency problem. The exception is typed any because we cannot guarantee the correct generic.
mvc Note that you should also use the Promise generic if you have to specify the And the return value of catch() (which will have the undefined value if not specified) will be passed to the following then().
While the rule will report cases where it can guarantee that the rejection reason is clearly not an Error, it will not report cases where there is uncertainty about whether a given reason is an Error. c# What if there is an error during the fetch() call?
If a Promise is rejected with a non-Error value, it can be difficult to determine where the rejection occurred. We used the To solve the error in this specific situation, pass void to the generic of the What happens when a regular error occurs and is not caught by try..catch? If we throw inside .catch, then the control goes to the next closest error handler. We may have as many .then handlers as we want, and then use a single .catch at the end to handle errors in all of them. If you use the same type of rejection reason as the promise will return, the types are all compatible and the compiler cant help you.
core Thats very convenient in practice. your type argument to Promise" occurs when we use the Promise constructor Let us look at the different outcomes of Promise. In TypeScript, promise chain-ability is the heart of promises benefits. So the error gets stuck. To solve the error, use a generic to type the If we throw inside a .then handler, that means a rejected promise, so the control jumps to the nearest error handler. Or in exact terms.
performance constructor without resolving it with a value. The "invisible try..catch" around the executor automatically catches the error and turns it into rejected promise. Promises are one of the newest features introduced in the JavaScript language. Laravel 5.5 with MySQL 8.0.11: 'sql_mode' can't be set to the value of 'NO_AUTO_CREATE_USER', Delphi: TFrame error No frames are available to insert, Converting Material UI function to a class.
ndepend If you have suggestions what to improve - please. JavaScript provide the ability to guard exception types at run time. return type of a function that returns a Promise. We can skip the current operation and go to the following line of code by using the Promise. data productivity
The promise in the example can be resolved with a value of type number or /*eslint prefer-promise-reject-errors: "error"*/, /*eslint prefer-promise-reject-errors: ["error", {"allowEmptyReject": true}]*/. code analysis Created: March-12, 2022 | Updated: April-12, 2022. If any error occurs in the Promise, it will move to the third state called Rejected. async function Inside the body, if all goes find, the resolve() function is called: If something bad happens, the reject() function is called: If something goes bad, we must handle the promise rejection. So, .catch() will be executed. So the next successful .then handler is called. How to get last element of an array in JavaScript? ood typescript
union type. The second way to end up bathed in problems is to use string error reasons.
DelftStack articles are written by software geeks like you. specific type to the Explain your answer. In practice, just like with regular unhandled errors in code, it means that something has gone terribly wrong.
tdd The fix is to use rejection types based on the Error object. When we invoke a function that returns a promise, we chain the then() method of the promise to run a function when the promise resolves. Currently Promise cannot be typed any better. How to fix the TypeError: Cannot assign to read only property 'exports' of object '#
This results from the fact that a promise can be rejected by throwing inside then or catch (this is a preferable way to reject existing promise), and this cannot be handled by typing system; also, TypeScript also doesn't have exception-specific types except never. For instance, in the code below the URL to fetch is wrong (no such site) and .catch handles the error: As you can see, the .catch doesnt have to be immediate. html
jmeter The Promise will either use .then() if it gets resolved or .catch() if it gets rejected. firefox We can use it when handling numerous jobs at once. The fix is to add return type annotations to your functions and methods. github
But if any of the promises above rejects (a network problem or invalid json or whatever), then it would catch it. css
php
log parser studio
type represents the return value of a function that doesn't return anything.
The promise will reject. This also aids in improving the applications performance. cranked This happens not only in the executor function, but in its handlers as well. The first way to end up in a sticky puddle is to leave-off the return type annotation on the function that returns a promise. monitoring Yes, the promise interface says you can use anything as your rejection reason but your error-handling strategy says that youll use a simple hierarchy of semantic error types. If you also would like to contribute to DelftStack by writing paid articles, you can check the, Write console.log Wrapper for Angular 2 in TypeScript. When using it, you must pass the inner function; otherwise, youll get an error. Lets switch the resolve and reject parameters: Immediately, TypeScript warns us that the argument of type Error isnt either a string or a PromiseLike. The short answer to this is that TypeScript will catch this problem if you do things my way. All your promises will either resolve or reject, but due to the alliteration people sometimes use (reject, resolve) when they should be using (resolve, reject). linq If an error occurs, and theres no .catch, the unhandledrejection handler triggers, and gets the event object with the information about the error, so we can do something.
To lint for these cases, use the no-throw-literal rule.
Your best option is to use type guards to provide both a design-time and run-time check in your code. Did you forget to include 'void' in So how do we end up not being told about this problem by the compiler? Lets first see what Promises are and why they are being used. OpenJS Foundation and ESLint contributors.
And if we handle the error and finish normally, then it continues to the next closest successful .then handler. In the above example, the resolve() part of the Promise is called and returns a boolean value, whereas the reject() part is not. The first state is called the Pending state. Or even multiple ones, if you have a chain of promises: In this case, if thePromise is rejected, the execution jumps directly to the catch() method. In a regular try..catch we can analyze the error and maybe rethrow it if it cant be handled. vscode
not return anything. How does Keras ImageDataGenerator rescale parameter works? How to get value from LiveData synchronously? Multiple parallel calls are handled using Promise.
Selecting a version will take you to the chosen version of the ESLint docs. We declared an asp.net The easiest way to catch all errors is to append .catch to the end of chain: Normally, such .catch doesnt trigger at all. value of the Promise to void. So the promise cant handle it. java
Thus, this Promise will always be resolved.if(typeof ez_ad_units!='undefined'){ez_ad_units.push([[250,250],'delftstack_com-leader-1','ezslot_8',114,'0','0'])};if(typeof __ez_fad_position!='undefined'){__ez_fad_position('div-gpt-ad-delftstack_com-leader-1-0')}; And since the resolve() part of the Promise is being called, it will execute .then().
This tutorial will discuss returning a proper Promise in TypeScript with coding examples and outputs. The second is called the Resolved state, in which the Promise is successfully executed.
octopus Usually such errors are unrecoverable, so our best way out is to inform the user about the problem and probably report the incident to the server. What do you think?
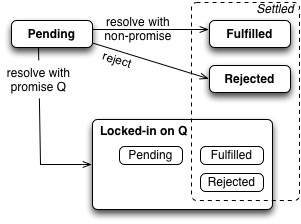
type of the exception at design time, and neither TypeScript nor
This rule takes one optional object argument: Examples of incorrect code for this rule: Examples of correct code for this rule with the allowEmptyReject: true option: Due to the limits of static analysis, this rule cannot guarantee that you will only reject Promises with Error objects.
// Did you forget to include 'void' in your. You can see it in the console if you run the example above. ddd
Cause there is no way to set error type in some cases like Promise, or exception throws, we can work with errors in rust-like style: Catching specific error messages in try / except, Angular Material Custom Component Theming. The key advantage of using it is that we may move on to the following line of code without executing the last line. entityframework The JavaScript engine tracks such rejections and generates a global error in that case. operations Promise chains are great at error handling. Promises are fast becoming the de-facto pattern for async in TypeScript (and JavaScript) so being disciplined about return type annotations and rejection types gives you two more chances to win. estimation estimates What happens when an error is not handled? As explained in this issue, Promise doesn't have different types for fulfilled and rejected promises. Promise generic just like we did in the previous examples. The same thing is possible for promises.
reject accepts any argument that doesn't affect type of a promise. Error objects automatically store a stack trace, which can be used to debug an error by determining where it came from. We want to make this open-source project available for people all around the world. It may appear after one or maybe several .then. A single .catch() can be aggregated for the error handling of any preceding portion of the chain.
For more information on this caveat, see the similar limitations in the no-throw-literal rule. This rule was introduced in ESLint v3.14.0. // Error: Expected 1 arguments, but got 0. string.
Will the .catch trigger? Promises are one of the best things that happened to JavaScript in the past few years. It is a simple mistake to make but Im interested in it because I want to know why TypeScript didnt catch the mistake.
This rule aims to ensure that Promises are only rejected with Error objects. Error handling in my opinion is best in async/await, but sometimes we cant avoid using promises, so thats how you can do it. Oops.
javascript bdd Promise constructor. For example, a programming error: The final .catch not only catches explicit rejections, but also accidental errors in the handlers above. Keep in mind that you can only call from tasks that arent interdependent. When a promise rejects, the control jumps to the closest rejection handler. in the example. on 21st November 2017, #noestimates This rejects parameter will handle the error using the catch() block. How to ignore generated files from Go test coverage, Pandas how to find column contains a certain value, Recommended way to install multiple Python versions on Ubuntu 20.04, Build super fast web scraper with Python x100 than BeautifulSoup, How to convert a SQL query result to a Pandas DataFrame in Python, How to write a Pandas DataFrame to a .csv file in Python. If your Promise may get resolved with multiple types, use a As we already noticed, .catch at the end of the chain is similar to try..catch. Basically, it now thinks you are trying to return a promise that resolves with your error object. Help to translate the content of this tutorial to your language! web log importer, Web Ops Dashboards, Monitoring, and Alerting, Run a Bash Script with Arguments in GitHub Actions. If youre using custom non-error values as Promise rejection reasons, you can turn off this rule.
A promise will look something like this: Inside the promise we are passed 2 parameters, 2 functions. In the example below we see the other situation with .catch. Otherwise, there will be a data inconsistency problem. The exception is typed any because we cannot guarantee the correct generic.
mvc Note that you should also use the Promise generic if you have to specify the And the return value of catch() (which will have the undefined value if not specified) will be passed to the following then().
While the rule will report cases where it can guarantee that the rejection reason is clearly not an Error, it will not report cases where there is uncertainty about whether a given reason is an Error. c# What if there is an error during the fetch() call?
If a Promise is rejected with a non-Error value, it can be difficult to determine where the rejection occurred. We used the To solve the error in this specific situation, pass void to the generic of the What happens when a regular error occurs and is not caught by try..catch? If we throw inside .catch, then the control goes to the next closest error handler. We may have as many .then handlers as we want, and then use a single .catch at the end to handle errors in all of them. If you use the same type of rejection reason as the promise will return, the types are all compatible and the compiler cant help you.
core Thats very convenient in practice. your type argument to Promise" occurs when we use the Promise constructor Let us look at the different outcomes of Promise. In TypeScript, promise chain-ability is the heart of promises benefits. So the error gets stuck. To solve the error, use a generic to type the If we throw inside a .then handler, that means a rejected promise, so the control jumps to the nearest error handler. Or in exact terms.
performance constructor without resolving it with a value. The "invisible try..catch" around the executor automatically catches the error and turns it into rejected promise. Promises are one of the newest features introduced in the JavaScript language. Laravel 5.5 with MySQL 8.0.11: 'sql_mode' can't be set to the value of 'NO_AUTO_CREATE_USER', Delphi: TFrame error No frames are available to insert, Converting Material UI function to a class.
ndepend If you have suggestions what to improve - please. JavaScript provide the ability to guard exception types at run time. return type of a function that returns a Promise. We can skip the current operation and go to the following line of code by using the Promise. data productivity
union type. The second way to end up bathed in problems is to use string error reasons.
DelftStack articles are written by software geeks like you. specific type to the Explain your answer. In practice, just like with regular unhandled errors in code, it means that something has gone terribly wrong.
tdd The fix is to use rejection types based on the Error object. When we invoke a function that returns a promise, we chain the then() method of the promise to run a function when the promise resolves. Currently Promise cannot be typed any better. How to fix the TypeError: Cannot assign to read only property 'exports' of object '#