Id rather be confident that my test suite covers as much of my code as possible (to a point) than be a testing purest that insists that all unit tests only exercise code paths with no external dependencies. We check that by running: Here it says mockgen not found. So now you know one power of the gomock package. postgres createdb dropdb migrateup migratedown sqlc test server mock, // account, err := server.store.GetAccount(ctx, req.ID), // ctx.JSON(http.StatusNotFound, errorResponse(err)), // ctx.JSON(http.StatusInternalServerError, errorResponse(err)), Backend Master Class [Go + Postgres + Kubernetes + AWS]. But it only covers the happy case for now. The first context argument could be any value, so we use gomock.Any() matcher for it. It does not test anything. I think a user on the Gopher slack put it best when they said: Dont try to mock SQL. Depends on your product's intended use. OK, so today we have learned how to use gomock to generate mocks for our DB interface, and use it to write unit tests for the Get Account API to achieve 100% coverage. And we can now see that the InternalServerError branch in the code is now covered. Require no errors, then require the gotAccount to be equal to the input account. If you use a real DB, all tests will read and write data to the same place, so it would be harder to avoid conflicts, especially in a big project with a large code base. Each test case will have a unique name to separate it from others. To make the test more robust, we should check the response body as well. Now, what if we want to check more than just the HTTP status code? Under what conditions?)? I'm using vim, so let's press i to enter the insert mode. I haven't used the go bindings, but apparently they do exist: According to that, they are running podman as a service with a REST API, which isn't a million miles from a docker daemon - except that the podman service runs as your own userid, not as root. And thats all. DO NOT EDIT. Dont worry if you dont fully understand it right now. Theyre just general interface type: Later we will see how this function is used to build stubs. "github.com/techschool/simplebank/db/sqlc", // MockStore is a mock of Store interface, // MockStoreMockRecorder is the mock recorder for MockStore, // NewMockStore creates a new mock instance, // EXPECT returns an object that allows the caller to indicate expected use, // AddAccountBalance indicates an expected call of AddAccountBalance. It really depends on what you are looking for in terms of features. // like saving the query to validate it later, etc. Then we call server.router.ServeHTTP() function with the created recorder and request objects. So lets create a new main_test.go file inside the api package and config Gin to use Test mode instead. To model the business logic, the data models look something like this: Next, you write all the code required to do basic CRUD in the application. Now all we need to do is to call gin.SetMode to change it to gin.TestMode. Lucky for us, the sqlc package that we used to generate CRUD codes also has an option to emit an interface that contains all of the function of the Queries struct. So lets build stub for this method by calling store.EXPECT().GetAccount(). So now its time to add more cases. Im gonna duplicate the happy case's test data. Prepares the environment to make sure that the portion of code to be tested is going to be functional when it is executed. We want to test that we can correctly interact with the database and also test that the business logic is correct. Let's say you have a SQL describing your application structure: If you intend to use ramsql with the GORM ORM, you should use the GORM Postgres driver. The purpose of Tech School is to give everyone a chance to learn IT by giving free, high-quality tutorials and coding courses. prog.go:14:2: no required module provides package github.com/acetorscode/learningbank/db/sqlc: go.mod file not found in current directory or any parent directory; see 'go help modules' The manually typed SQL commands were also error prone, and not everybody in the team is familiar with various SQL dialects. Mock objects meet the interface requirements of, and stand in for, more complex real ones. All we need to do is to check that response. https://golang.org/doc/faq#Is_Go_an_object-oriented_language, https://www.softwaretestinghelp.com/types-of-software-testing/. If you like the article, please subscribe to our Youtube channel and follow us on Twitter or Facebook for more tutorials in the future. Your tests are isolated, and compliant with go tools. Things would be more complicated if this source file imports packages from other files, which is often the case when we work on a real project.
It will be a real implementation of the Store interface that talks to a SQL database, which is PostgreSQL in this case. The following image clarifies this idea. You might also want to look at podman, which runs containers directly as processes under your own userid with no docker daemon - and buildah, the corresponding tool for creating container images. Perhaps adopt a pattern where your test cases look for an environment variable (or more than one), that defines how to connect to a database. So we use this matcher: gomock.Eq() and pass the account.ID to it. If it is about testing data persistence, rephrasing my earlier response, we do not test data persistence, not very thoroughly anyway, because it is auto-generated by a tool. If youre using a bash shell then you should edit the .bash_profile or .bashrc file instead. n.d. Types Of Software Testing: Different Testing Types With Details. This way we can abstract the solution to our problem using interfaces. It will become hidden in your post, but will still be visible via the comment's permalink. To add it to the PATH, I will edit the .zshrc file since Im using zsh. First, we need to declare a list of test cases. DEV Community 2016 - 2022. Understanding why tests must be isolated is important. In the code below, the method ValidateStudents receives an interface as a parameter, so we can pass it the real database object or use a custom mock. So lets use the -destination option to tell it to write the mock store codes to db/mock/store.go file: Now get back to visual studio code, we can see that a new file store.go is generated inside the db/mock folder: In this file, there are 2 important struct: MockStore and MockStoreMockRecorder. It is worth mentioning that sub-tests are solely related to their parents and they must be isolated from each other. First, lets create the interfaces that will allow us to keep the database library code and implemented mocks separate. On Thu, Jul 29, 2021 at 5:13 PM Brian Candler <, Set up interfaces that other packages expect/deal with for DB operations, Have your postgres|mysql|whatever package satisfy that interface (effectively a driver for that interface), In the packages that import your datastore interface, stub/mock the interface for testing, Keep the integration tests associated with the specific datastore implementation, to Marcin Romaszewicz, Henry, golang-nuts, to Andrew Werner, Brian Candler, golang-nuts, https://www.youtube.com/watch?v=wB1hoUJDbk0, https://robots.thoughtbot.com/interface-with-your-database-in-go, https://github.com/pingcap/tidb/blob/master/docs/USAGE.md, https://pkg.go.dev/github.com/DATA-DOG/go-sqlmock, https://github.com/WangXiangUSTC/tidb-lite, https://groups.google.com/d/msgid/golang-nuts/4fbc3ae9-b0ff-4750-bfba-1d58a1dba986n%40googlegroups.com, https://groups.google.com/d/msgid/golang-nuts/ec72823c-d528-48a6-9d4c-704f0862fcb6n%40googlegroups.com, https://news.ycombinator.com/item?id=10002142, https://groups.google.com/d/msgid/golang-nuts/5829cbca-703d-42c4-9338-5f739375568an%40googlegroups.com, https://groups.google.com/d/msgid/golang-nuts/df878e11-3a70-45d1-9cc3-7a73d864ee9an%40googlegroups.com, https://github.com/testcontainers/testcontainers-go, https://groups.google.com/d/msgid/golang-nuts/9c81746a-4fb4-4fb5-8e5f-605169a3f2afn%40googlegroups.com, https://groups.google.com/d/msgid/golang-nuts/CA%2Bv29Lv83-4yDijNmukf0Vx%2BoBVZXJPR11bqA_B5CY1mNhOowA%40mail.gmail.com, https://podman.io/blogs/2020/08/10/podman-go-bindings.html, https://groups.google.com/d/msgid/golang-nuts/4d6f1b9d-ad44-4fc5-b309-beba2a199382n%40googlegroups.com, https://groups.google.com/d/msgid/golang-nuts/CA%2BvRuzPvz%2Bu2NCubgnWEiu-D29HVj13tGxUHmEVpaTTdcqyO0Q%40mail.gmail.com, load the snapshot into in memory database instance. It will have 3 input arguments: the testing.T, the response body of type byte.Buffer pointer, and the account object to compare. Because our code that talks to the real DB is already tested carefully in the previous lecture. The idea is to use gomock package to generate and build stubs that return hard-coded values for each scenario we want to test. We can use it to start the test HTTP server and send GetAccount request. How do you test your data access in go code? You can apply this knowledge to write tests for other HTTP APIs in our simple bank project such as Create Account or Delete Account API. Next, you flesh out the rest of the BookStore with methods such as UpdateBook, GetBook, and DeleteBook. We want to keep the test data separate from production data. All actions will be performed in memory and within the same process. Therefore, we must update this to Times(0), and remove the Return function call. Are you sure you want to hide this comment? Note that quite a number of Go ORM libraries already support multiple databases. The first one is to implement a fake DB, which stores data in memory. Next we will declare the url path of the API we want to call, which should be /accounts/{ID of the account we want to get}. Generating models only reduces the cognitive and time overhead of going back and forth from the code to the db. However, it will be too time-consuming because this struct can contain a lot of functions. The reason is that Gin is running in Debug mode by default. Of course, you will also need an AuthorStore to manage the authors in your app. Instead, we can just use the recording feature of the httptest package to record the response of the API request. Note that the url should be created with the tc.accountID so that it will use the account ID defined for each test case. Other than that, they serve a similar purpose.
Now we can write the actual storage layer tests that test the basic CRUD functionality like so: The storage tests will now be reading and writing to the database, so we can be more confident that our code works as intended. First I will create a new mock folder inside the db package. // ValidateStudents does some validation over the recorded students. Im gonna create a new file account_test.go inside the api package. But their behavior could be important, so we define interfaces in our code to use custom implementations of the interfaces and use a custom implementation for our tests. Which means that, having tests T1, T2 and T3, each one could be executed in isolation like a black box. Before we go deeper into our solution for testing database interaction in Golang through abstraction, we should define what we mean by abstraction. Now lets go back to our test file and run the whole package tests. Testing is an important part of any project. They can still re-publish the post if they are not suspended. We can use this mock store to build the stub that suits the purpose of each test case. Remote or onsite in Paris/Amsterdam/London/Berlin/Barcelona with visa sponsorship. It will be great to hear if anybody has some other experiences to share. // Package mock_sqlc is a generated GoMock package. For now, lets refactor the code a bit to make it work for multiple scenarios. All we have to do is to change this emit_interface setting in the sqlc.yaml file to true: Then run this command in the terminal to regenerate the codes: After this, in the db/sqlc folder, we can see a new file called querier.go. . Then open its Github page. A unique engine is tied to a single sql.DB with as much sql.Conn as needed providing a unique DataSourceName. It has 2 input arguments: a testing.T, and a httptest.ResponseRecorder object. The difference is that other ORM libraries usually provide a general purpose data access library, whereas ours generates more specific codes. For our purposes lets agree that it is an object-oriented programming language. It's basically the simple bank module name github.com/techschool/simplebank followed by /db/sqlc because our Store interface is defined inside the db/sqlc folder. Im gonna use an anonymous class to store the test data. But for me, mocking is better because of the following reasons: OK, that sounds great. It expects a gomock.Controller object as input, so we have to create this controller by calling gomock.NewController and pass in the testing.T object. We write each test as a self-contained unit, independent of the other tests. Now that we know that the code that interacts with the database can be well tested and uses actual SQL, we can start testing our service layer by introducing mocks when needed. The second argument we need to pass in this command is the name of the interface, which is Store in this case. We call tc.buildStubs() function with the mock store before sending the request, and finally call tc.checkResponse() function at the end to verify the result. It is a struct which will always connect to the real database: So in order to use a mock DB in the API server tests, we have to replace that store object with an interface. Having a model that you are sure is a reflection of the db does not help you know that the logic going with it works? And looking at the code of the getAccount handler, we can see that it is 100% covered. So today Im gonna show you a better way to mock DB, which is using stubs instead of fake DB. Our data access code were quite fragile to changes. DEV Community A constructive and inclusive social network for software developers. Next, for the buildStubs function, Im gonna copy its signature here. First, it helps us to write independent tests more easily because each test will use its own separate mock DB to store data, so there will be no conflicts between them. I dont see embedded versions of PostgreSQL or MySql. Once unsuspended, techschoolguru will be able to comment and publish posts again. // IDB is the interface that defines the methods that must be implemented. And now the logs look much cleaner and easier to read than before. We use an SQL database so we do not have to mock DB calls and maintain an interface. go mod download github.com/golang/mock and only accessible to TECH SCHOOL. On Thu, Jul 29, 2021 at 4:39 AM Marcin Romaszewicz <. So all we need to do is to call its EXPECT() function to build a stub, which tells gomock that: this GetAccount() function should be called exactly 1 time with this input accountID, and return this account object as output. The last scenario we should test is BadRequest, which means the client has sent some invalid parameters to this API.
Then everything will be working just fine when being put together. We're a place where coders share, stay up-to-date and grow their careers. In this article, we will learn how to use Gomock to generate stubs for the DB interface, which helps us write API unit tests faster, cleaner, and easily achieve 100% coverage. While you're here, sign up to receive a Lets rerun the whole package tests! Alright, so now as we have the db.Store interface, we can use gomock to generate a mock implementation of it. Yee, it passed!
if you insert data in your fake database, are you expecting it to be able to return it to you? Let's say you want to test the function LoadUserAddresses : Use RamSQL to test it in a disposable isolated in-memory SQL engine : Done. Made with love and Ruby on Rails. We should also specify the destination of the generated output file. Now in our service layer code, we can rely on the BookStore interface like so: We can now mock the responses from the BookStore interface type to test any complicated combination of errors/invalid objects that we want! It produces different SQL for different databases. [1]), this post will focus on Unit Tests. This approach of using fake db is very simple and easy to implement. Similarly, we have a checkResponse function to check the output of the API. Right now only the not found case and the successful case are covered. There are many variables outside our code that can affect the behavior of the program. In the happy case, it should be http.StatusOK. Basically, this will send our API request through the server router and record its response in the recorder. Then add this export command to the top of the file: Press Esc to exit the insert mode, then :wq to save the file and quit vim. Are we talking about testing the domain logic or the data persistence? The latest SQLite covers the vast majority of Postgres queries, so most tests simply use an SQLite in-memory DB. Why 2 input arguments? Once suspended, techschoolguru will not be able to comment or publish posts until their suspension is removed. Now we get to the meat of the issue, how/what do you test? And since its a GET request, we can use nil for the request body. Softwaretestinghelp.com. Now whenever we want to regenerate the mock store, we can simple run make mock in the terminal. An interface is a type or structure that defines the methods (with their signatures) that need to be implemented for any object to fulfill it. And thats it! Print version. It is an upfront investment, so that we do not have to do similar tests for the subsequent projects. It makes writing unit tests so easy and saves us tons of time implementing the mock interface. A very important aspect of writing our own mocks is that the tests explain the main code by themselves, so they really complement the code. We require no errors to be returned. For further actions, you may consider blocking this person and/or reporting abuse. The danger with mocking too much is that your unit tests end up testing the mocks, and not anything remotely like your runtime environment, so we've chosen to mock as little as possible. In this case, we only care about the GetAccount() method, since its the only method that should be called by the Get Account API handler. With you every step of your journey. It was tedious to write such tool, but it was a time well spent. For now, lets create a new store by calling mockdb.NewMockStore() with this input controller. We can add more methods as needed but we should implement the ones defined in the interface at a minimum. OK, now with the generated MockStore, we can start writing test for our APIs. Do you need something that is able to error on syntax problems? The second case we want to test is when the account is not found. I am just looking at options to write tests for parts of my application that interacts with a SQL database. Deeply understand Isolation levels and Read phenomena in MySQL & PostgreSQL, How to setup Github Actions for Go + Postgres to run automated tests, Implement RESTful HTTP API in Go using Gin, Load config from file & environment variables in Golang with Viper, Mock DB for testing HTTP API in Go and achieve 100% coverage, Implement transfer money API with a custom params validator in Go, Add users table with unique & foreign key constraints in PostgreSQL, How to handle DB errors in Golang correctly, How to write stronger unit tests with a custom go-mock matcher. Here Times(1) means we expect this function to be called exactly 1 time. Have you ever started writing an application in Go that reads and writes to a database only to later be confused about how to properly write tests for it? Lets copy its signature. How do you ensure that your tests run quickly but are also effectively testing the edge cases? There are several mock packages that you can find. Why PASETO is better than JWT for token-based authentication? Posted on Nov 14, 2020 We use a simple for loop to iterate through the list of test cases. // Code generated by MockGen. This is because I believe that this validation type code should be abstracted from the concrete storage code as much as possible since validation is really a business logic concern. The tool is not open-source, but there are plenty of ORM and ORM-like open source Go libraries around. Bottom line : One DataSourceName per test and you have full test isolation in no time. P.S. missing go.sum entry for module providing package github.com/golang/mock/mockgen/model; to add: We can use the same accountID here because mock store is separated for each test case. With a mock DB, we can easily set up and test some edge cases, such as an unexpected error, or a connection lost, which would be impossible to achieve if we use a real DB. Once again, Im gonna duplicate the test data, change its name to "InternalError". So here we call httptest.NewRecorder() to create a new ResponseRecorder. For the checkResponse, we must change the status code to http.StatusBadRequest. What you could end up with is obscure code that is not easily understood nor maintained. Lets open the browser and search for gomock. Then move the store.EXPECT command into this function. In this case, it should be considered an internal error, so in the checkResponse function, we must require the recorder.Code to be equal to http.StatusInternalServerError. This status code is recorded in the Code field of the recorder. I think in the long run, it is a worthy investment. For the tests which require Postgres- specific functionality, such as partitioned tables, for example, we use. When it comes to testing these APIs, some people might choose to connect to the real database, while some others might prefer to just mocking it. More than that, we can use the Return() function to tell gomock to return some specific values whenever the GetAccount() function is called. We could have an object that implements this interface, which could be achieved by adding methods that follow the signatures of the methods defined in the interface. unit tests, regression tests, integration tests, security tests, etc. We gonna run each case as a separate sub-test of this unit test, so lets call t.Run() function, pass in the name of this test case, and a function that takes testing.T object as input. Queries order matter! Before jumping into more code, lets go over a few self-imposed constraints: These constraints raise an interesting question, namely: When should you mock in your tests and when should you interact with the real database? You do this manually first, by inserting, updating, deleting books and authors in your application, but as an experienced developer, you know that you really should write tests! Great! All passed. I'm not quite sure the intent is the same? This is demonstrated by @francesc in the first half of this demo: For example, you create an interface with Save, Search, Delete methods, and so on. We should defer calling Finish method of this controller.
First, we need to install gomock. In the account.go file, we can see that this getAccount handler is not 100% covered. Now with this struct definition, lets add the first scenario for the happy case. prog.go:12:2: no required module provides package github.com/golang/mock/mockgen/model: go.mod file not found in current directory or any parent directory; see 'go help modules' We did however thoroughly tested the tool (and the code it generates) against actual common SQL databases. We require no errors to be returned. After all, since mocks are in memory, it would satisfy requirements 2 and 4 above, as it would allow the tests to run quickly and keep the test data separate from production data in the database. But for this lecture, we will only write tests for the most important one: Get Account API. When the test runs, having those dependencies could make our tests return different results every time they run. Updates the environment with fixed arguments or updates needed according to the test case. To setup the tests for the storage layer, youll need to first create a real SQL database and also create the books and authors tables. (default true). Have a look at our careers page and reach out to us if you would like to be a part of our team! However, the test log is currently containing too much information. For example, heres the AddAccountBalance() function of the MockStore, which takes a context and an AddAccountBalanceParams as input and returns an Account or an error: The MockStoreMockRecorder also has a function with the same name and the same number of arguments. -source string // Source: github.com/techschool/simplebank/db/sqlc (interfaces: Store). Lets comment out this block of code and just set account to be an empty object. The third and very important reason for mocking DB is: It allows us to write tests that achieve 100% coverage. They all have their quirks, and you need to test for those. The tool was then used by my colleagues, and over time they added their own enhancement. OK, so the GetAccount API unit test is working very well. If you use an interface instead of writing your code to speak SQL, you can create a dummy version that stores records in a map or something. And thats it! But then there is SQL queries, constraints, CRUDand suddenly you need a PostgresSQL, setup scripts and nothing is easy anymore. So far it seems like the community has few distinct schools of thought: - In-memory "real" DB solutions - such as tidb-lite for MySQL (, - Container based functional/integration style testing. Lets run the whole package test to see the code coverge. It also depends on the nature of what we are testing. Thats because the GetAccount() method of our Store interface requires 2 input parameters: a context and an account ID. Once you have good unit tests for your database-interacting low-level functions, and integration tests for the whole flow, you can just mock the db behavior in other higher level unit tests because you know it'll work in production. One example of this is when a program interacts with a database, there are many errors that can happen while our program is running: wrong credentials were used, the database just restarted, a query had a bad format, a deadlock occurs, a port is already in use, etc. There are many duplicate debug logs written by Gin, which make it harder to read the test result.
We will use this tool to generate the mock db, so its important to make sure that it is executable from anywhere. Otherwise, mockgen will write the generated codes to stdout by default. Next we have to run this source command to reload the .zshrc file: Now if we run which mockgen again, we can see that it is now available in the go/bin folder. To do this, well need a slight refactor of our Store code by abstracting away the implementation and instead relying on interfaces in the service layer.
Well, I would say its up to you. Then Im gonna move all of these statements into that function. In the api/account_test.go file, I will define a new function TestGetAccountAPI() with the testing.T input parameter. For example, in this case, we want it to return the account object and a nil error. We still have to test 2 more cases: InternalServerError and BadRequest. In this section we present a code snippet to show how to use interfaces and mocks with the application of abstraction to test how our code interacts with external factors like a database. If you followed my gRPC course then you definitely have already known about it. Golang is an intelligent language such that it checks if the contracts are being followed in order to define if an interface is being implemented. Then lets open the terminal and run: Mockgen gives us 2 ways to generate mocks. Can we be confident that our codes will still perform well when a real DB is plugged in? We do a test run against a copy of the actual database before deployment, and the final test run against the actual database during deployment. If that is something that is interesting i could trigger a blog post about what we did and why? In order to test this API, we need to have an account first. Creating unit tests is not always simple. youll only end up unhappy. In the db_test.go file we can make use of Gos built-in testing package and the TestMain function.
And the reason is due to a missing call to the store.GetAccount function. So I wrote the tool to auto generate the necessary code. Then the old Store struct will be renamed to SQLStore. This is very important because it will check to see if all methods that were expected to be called were called. To achieve the isolation in unit testing we can apply abstraction. If you have that problem you have poorly written tests. Yes, database libraries export some methods and their implementation is not very important to us. This time, the test failed. I have this exact testing issue at my company, we have many Go services which use Postgres in production, but are unit tested against SQLite. Then lets delete all statements of this function, except for the last one. Methods like validators that do something specific for the internal use of a library and we dont need to export them). In this application, the database is a complex, real system, and it is external to the code, so it seems like a good candidate to mock. The SQLite is not quite reach SQL implementation from enterprise point of view. (source mode) Input Go source file; enables source mode. Thats because the go/bin folder is not in the PATH environment variable at the moment. So all we need to do is: make sure that the mock DB implements the same interface as the real DB. Built on Forem the open source software that powers DEV and other inclusive communities. prog.go:12:2: missing go.sum entry for module providing package github.com/golang/mock/mockgen/model; to add:
It will be a real implementation of the Store interface that talks to a SQL database, which is PostgreSQL in this case. The following image clarifies this idea. You might also want to look at podman, which runs containers directly as processes under your own userid with no docker daemon - and buildah, the corresponding tool for creating container images. Perhaps adopt a pattern where your test cases look for an environment variable (or more than one), that defines how to connect to a database. So we use this matcher: gomock.Eq() and pass the account.ID to it. If it is about testing data persistence, rephrasing my earlier response, we do not test data persistence, not very thoroughly anyway, because it is auto-generated by a tool. If youre using a bash shell then you should edit the .bash_profile or .bashrc file instead. n.d. Types Of Software Testing: Different Testing Types With Details. This way we can abstract the solution to our problem using interfaces. It will become hidden in your post, but will still be visible via the comment's permalink. To add it to the PATH, I will edit the .zshrc file since Im using zsh. First, we need to declare a list of test cases. DEV Community 2016 - 2022. Understanding why tests must be isolated is important. In the code below, the method ValidateStudents receives an interface as a parameter, so we can pass it the real database object or use a custom mock. So lets use the -destination option to tell it to write the mock store codes to db/mock/store.go file: Now get back to visual studio code, we can see that a new file store.go is generated inside the db/mock folder: In this file, there are 2 important struct: MockStore and MockStoreMockRecorder. It is worth mentioning that sub-tests are solely related to their parents and they must be isolated from each other. First, lets create the interfaces that will allow us to keep the database library code and implemented mocks separate. On Thu, Jul 29, 2021 at 5:13 PM Brian Candler <, Set up interfaces that other packages expect/deal with for DB operations, Have your postgres|mysql|whatever package satisfy that interface (effectively a driver for that interface), In the packages that import your datastore interface, stub/mock the interface for testing, Keep the integration tests associated with the specific datastore implementation, to Marcin Romaszewicz, Henry, golang-nuts, to Andrew Werner, Brian Candler, golang-nuts, https://www.youtube.com/watch?v=wB1hoUJDbk0, https://robots.thoughtbot.com/interface-with-your-database-in-go, https://github.com/pingcap/tidb/blob/master/docs/USAGE.md, https://pkg.go.dev/github.com/DATA-DOG/go-sqlmock, https://github.com/WangXiangUSTC/tidb-lite, https://groups.google.com/d/msgid/golang-nuts/4fbc3ae9-b0ff-4750-bfba-1d58a1dba986n%40googlegroups.com, https://groups.google.com/d/msgid/golang-nuts/ec72823c-d528-48a6-9d4c-704f0862fcb6n%40googlegroups.com, https://news.ycombinator.com/item?id=10002142, https://groups.google.com/d/msgid/golang-nuts/5829cbca-703d-42c4-9338-5f739375568an%40googlegroups.com, https://groups.google.com/d/msgid/golang-nuts/df878e11-3a70-45d1-9cc3-7a73d864ee9an%40googlegroups.com, https://github.com/testcontainers/testcontainers-go, https://groups.google.com/d/msgid/golang-nuts/9c81746a-4fb4-4fb5-8e5f-605169a3f2afn%40googlegroups.com, https://groups.google.com/d/msgid/golang-nuts/CA%2Bv29Lv83-4yDijNmukf0Vx%2BoBVZXJPR11bqA_B5CY1mNhOowA%40mail.gmail.com, https://podman.io/blogs/2020/08/10/podman-go-bindings.html, https://groups.google.com/d/msgid/golang-nuts/4d6f1b9d-ad44-4fc5-b309-beba2a199382n%40googlegroups.com, https://groups.google.com/d/msgid/golang-nuts/CA%2BvRuzPvz%2Bu2NCubgnWEiu-D29HVj13tGxUHmEVpaTTdcqyO0Q%40mail.gmail.com, load the snapshot into in memory database instance. It will have 3 input arguments: the testing.T, the response body of type byte.Buffer pointer, and the account object to compare. Because our code that talks to the real DB is already tested carefully in the previous lecture. The idea is to use gomock package to generate and build stubs that return hard-coded values for each scenario we want to test. We can use it to start the test HTTP server and send GetAccount request. How do you test your data access in go code? You can apply this knowledge to write tests for other HTTP APIs in our simple bank project such as Create Account or Delete Account API. Next, you flesh out the rest of the BookStore with methods such as UpdateBook, GetBook, and DeleteBook. We want to keep the test data separate from production data. All actions will be performed in memory and within the same process. Therefore, we must update this to Times(0), and remove the Return function call. Are you sure you want to hide this comment? Note that quite a number of Go ORM libraries already support multiple databases. The first one is to implement a fake DB, which stores data in memory. Next we will declare the url path of the API we want to call, which should be /accounts/{ID of the account we want to get}. Generating models only reduces the cognitive and time overhead of going back and forth from the code to the db. However, it will be too time-consuming because this struct can contain a lot of functions. The reason is that Gin is running in Debug mode by default. Of course, you will also need an AuthorStore to manage the authors in your app. Instead, we can just use the recording feature of the httptest package to record the response of the API request. Note that the url should be created with the tc.accountID so that it will use the account ID defined for each test case. Other than that, they serve a similar purpose.
Now we can write the actual storage layer tests that test the basic CRUD functionality like so: The storage tests will now be reading and writing to the database, so we can be more confident that our code works as intended. First I will create a new mock folder inside the db package. // ValidateStudents does some validation over the recorded students. Im gonna create a new file account_test.go inside the api package. But their behavior could be important, so we define interfaces in our code to use custom implementations of the interfaces and use a custom implementation for our tests. Which means that, having tests T1, T2 and T3, each one could be executed in isolation like a black box. Before we go deeper into our solution for testing database interaction in Golang through abstraction, we should define what we mean by abstraction. Now lets go back to our test file and run the whole package tests. Testing is an important part of any project. They can still re-publish the post if they are not suspended. We can use this mock store to build the stub that suits the purpose of each test case. Remote or onsite in Paris/Amsterdam/London/Berlin/Barcelona with visa sponsorship. It will be great to hear if anybody has some other experiences to share. // Package mock_sqlc is a generated GoMock package. For now, lets refactor the code a bit to make it work for multiple scenarios. All we have to do is to change this emit_interface setting in the sqlc.yaml file to true: Then run this command in the terminal to regenerate the codes: After this, in the db/sqlc folder, we can see a new file called querier.go. . Then open its Github page. A unique engine is tied to a single sql.DB with as much sql.Conn as needed providing a unique DataSourceName. It has 2 input arguments: a testing.T, and a httptest.ResponseRecorder object. The difference is that other ORM libraries usually provide a general purpose data access library, whereas ours generates more specific codes. For our purposes lets agree that it is an object-oriented programming language. It's basically the simple bank module name github.com/techschool/simplebank followed by /db/sqlc because our Store interface is defined inside the db/sqlc folder. Im gonna use an anonymous class to store the test data. But for me, mocking is better because of the following reasons: OK, that sounds great. It expects a gomock.Controller object as input, so we have to create this controller by calling gomock.NewController and pass in the testing.T object. We write each test as a self-contained unit, independent of the other tests. Now that we know that the code that interacts with the database can be well tested and uses actual SQL, we can start testing our service layer by introducing mocks when needed. The second argument we need to pass in this command is the name of the interface, which is Store in this case. We call tc.buildStubs() function with the mock store before sending the request, and finally call tc.checkResponse() function at the end to verify the result. It is a struct which will always connect to the real database: So in order to use a mock DB in the API server tests, we have to replace that store object with an interface. Having a model that you are sure is a reflection of the db does not help you know that the logic going with it works? And looking at the code of the getAccount handler, we can see that it is 100% covered. So today Im gonna show you a better way to mock DB, which is using stubs instead of fake DB. Our data access code were quite fragile to changes. DEV Community A constructive and inclusive social network for software developers. Next, for the buildStubs function, Im gonna copy its signature here. First, it helps us to write independent tests more easily because each test will use its own separate mock DB to store data, so there will be no conflicts between them. I dont see embedded versions of PostgreSQL or MySql. Once unsuspended, techschoolguru will be able to comment and publish posts again. // IDB is the interface that defines the methods that must be implemented. And now the logs look much cleaner and easier to read than before. We use an SQL database so we do not have to mock DB calls and maintain an interface. go mod download github.com/golang/mock and only accessible to TECH SCHOOL. On Thu, Jul 29, 2021 at 4:39 AM Marcin Romaszewicz <. So all we need to do is to call its EXPECT() function to build a stub, which tells gomock that: this GetAccount() function should be called exactly 1 time with this input accountID, and return this account object as output. The last scenario we should test is BadRequest, which means the client has sent some invalid parameters to this API.
Then everything will be working just fine when being put together. We're a place where coders share, stay up-to-date and grow their careers. In this article, we will learn how to use Gomock to generate stubs for the DB interface, which helps us write API unit tests faster, cleaner, and easily achieve 100% coverage. While you're here, sign up to receive a Lets rerun the whole package tests! Alright, so now as we have the db.Store interface, we can use gomock to generate a mock implementation of it. Yee, it passed!
if you insert data in your fake database, are you expecting it to be able to return it to you? Let's say you want to test the function LoadUserAddresses : Use RamSQL to test it in a disposable isolated in-memory SQL engine : Done. Made with love and Ruby on Rails. We should also specify the destination of the generated output file. Now in our service layer code, we can rely on the BookStore interface like so: We can now mock the responses from the BookStore interface type to test any complicated combination of errors/invalid objects that we want! It produces different SQL for different databases. [1]), this post will focus on Unit Tests. This approach of using fake db is very simple and easy to implement. Similarly, we have a checkResponse function to check the output of the API. Right now only the not found case and the successful case are covered. There are many variables outside our code that can affect the behavior of the program. In the happy case, it should be http.StatusOK. Basically, this will send our API request through the server router and record its response in the recorder. Then add this export command to the top of the file: Press Esc to exit the insert mode, then :wq to save the file and quit vim. Are we talking about testing the domain logic or the data persistence? The latest SQLite covers the vast majority of Postgres queries, so most tests simply use an SQLite in-memory DB. Why 2 input arguments? Once suspended, techschoolguru will not be able to comment or publish posts until their suspension is removed. Now we get to the meat of the issue, how/what do you test? And since its a GET request, we can use nil for the request body. Softwaretestinghelp.com. Now whenever we want to regenerate the mock store, we can simple run make mock in the terminal. An interface is a type or structure that defines the methods (with their signatures) that need to be implemented for any object to fulfill it. And thats it! Print version. It is an upfront investment, so that we do not have to do similar tests for the subsequent projects. It makes writing unit tests so easy and saves us tons of time implementing the mock interface. A very important aspect of writing our own mocks is that the tests explain the main code by themselves, so they really complement the code. We require no errors to be returned. For further actions, you may consider blocking this person and/or reporting abuse. The danger with mocking too much is that your unit tests end up testing the mocks, and not anything remotely like your runtime environment, so we've chosen to mock as little as possible. In this case, we only care about the GetAccount() method, since its the only method that should be called by the Get Account API handler. With you every step of your journey. It was tedious to write such tool, but it was a time well spent. For now, lets create a new store by calling mockdb.NewMockStore() with this input controller. We can add more methods as needed but we should implement the ones defined in the interface at a minimum. OK, now with the generated MockStore, we can start writing test for our APIs. Do you need something that is able to error on syntax problems? The second case we want to test is when the account is not found. I am just looking at options to write tests for parts of my application that interacts with a SQL database. Deeply understand Isolation levels and Read phenomena in MySQL & PostgreSQL, How to setup Github Actions for Go + Postgres to run automated tests, Implement RESTful HTTP API in Go using Gin, Load config from file & environment variables in Golang with Viper, Mock DB for testing HTTP API in Go and achieve 100% coverage, Implement transfer money API with a custom params validator in Go, Add users table with unique & foreign key constraints in PostgreSQL, How to handle DB errors in Golang correctly, How to write stronger unit tests with a custom go-mock matcher. Here Times(1) means we expect this function to be called exactly 1 time. Have you ever started writing an application in Go that reads and writes to a database only to later be confused about how to properly write tests for it? Lets copy its signature. How do you ensure that your tests run quickly but are also effectively testing the edge cases? There are several mock packages that you can find. Why PASETO is better than JWT for token-based authentication? Posted on Nov 14, 2020 We use a simple for loop to iterate through the list of test cases. // Code generated by MockGen. This is because I believe that this validation type code should be abstracted from the concrete storage code as much as possible since validation is really a business logic concern. The tool is not open-source, but there are plenty of ORM and ORM-like open source Go libraries around. Bottom line : One DataSourceName per test and you have full test isolation in no time. P.S. missing go.sum entry for module providing package github.com/golang/mock/mockgen/model; to add: We can use the same accountID here because mock store is separated for each test case. With a mock DB, we can easily set up and test some edge cases, such as an unexpected error, or a connection lost, which would be impossible to achieve if we use a real DB. Once again, Im gonna duplicate the test data, change its name to "InternalError". So here we call httptest.NewRecorder() to create a new ResponseRecorder. For the checkResponse, we must change the status code to http.StatusBadRequest. What you could end up with is obscure code that is not easily understood nor maintained. Lets open the browser and search for gomock. Then move the store.EXPECT command into this function. In this case, it should be considered an internal error, so in the checkResponse function, we must require the recorder.Code to be equal to http.StatusInternalServerError. This status code is recorded in the Code field of the recorder. I think in the long run, it is a worthy investment. For the tests which require Postgres- specific functionality, such as partitioned tables, for example, we use. When it comes to testing these APIs, some people might choose to connect to the real database, while some others might prefer to just mocking it. More than that, we can use the Return() function to tell gomock to return some specific values whenever the GetAccount() function is called. We could have an object that implements this interface, which could be achieved by adding methods that follow the signatures of the methods defined in the interface. unit tests, regression tests, integration tests, security tests, etc. We gonna run each case as a separate sub-test of this unit test, so lets call t.Run() function, pass in the name of this test case, and a function that takes testing.T object as input. Queries order matter! Before jumping into more code, lets go over a few self-imposed constraints: These constraints raise an interesting question, namely: When should you mock in your tests and when should you interact with the real database? You do this manually first, by inserting, updating, deleting books and authors in your application, but as an experienced developer, you know that you really should write tests! Great! All passed. I'm not quite sure the intent is the same? This is demonstrated by @francesc in the first half of this demo: For example, you create an interface with Save, Search, Delete methods, and so on. We should defer calling Finish method of this controller.
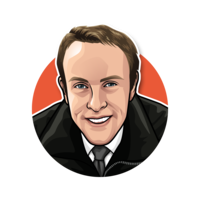
We will use this tool to generate the mock db, so its important to make sure that it is executable from anywhere. Otherwise, mockgen will write the generated codes to stdout by default. Next we have to run this source command to reload the .zshrc file: Now if we run which mockgen again, we can see that it is now available in the go/bin folder. To do this, well need a slight refactor of our Store code by abstracting away the implementation and instead relying on interfaces in the service layer.
Well, I would say its up to you. Then Im gonna move all of these statements into that function. In the api/account_test.go file, I will define a new function TestGetAccountAPI() with the testing.T input parameter. For example, in this case, we want it to return the account object and a nil error. We still have to test 2 more cases: InternalServerError and BadRequest. In this section we present a code snippet to show how to use interfaces and mocks with the application of abstraction to test how our code interacts with external factors like a database. If you followed my gRPC course then you definitely have already known about it. Golang is an intelligent language such that it checks if the contracts are being followed in order to define if an interface is being implemented. Then lets open the terminal and run: Mockgen gives us 2 ways to generate mocks. Can we be confident that our codes will still perform well when a real DB is plugged in? We do a test run against a copy of the actual database before deployment, and the final test run against the actual database during deployment. If that is something that is interesting i could trigger a blog post about what we did and why? In order to test this API, we need to have an account first. Creating unit tests is not always simple. youll only end up unhappy. In the db_test.go file we can make use of Gos built-in testing package and the TestMain function.
And the reason is due to a missing call to the store.GetAccount function. So I wrote the tool to auto generate the necessary code. Then the old Store struct will be renamed to SQLStore. This is very important because it will check to see if all methods that were expected to be called were called. To achieve the isolation in unit testing we can apply abstraction. If you have that problem you have poorly written tests. Yes, database libraries export some methods and their implementation is not very important to us. This time, the test failed. I have this exact testing issue at my company, we have many Go services which use Postgres in production, but are unit tested against SQLite. Then lets delete all statements of this function, except for the last one. Methods like validators that do something specific for the internal use of a library and we dont need to export them). In this application, the database is a complex, real system, and it is external to the code, so it seems like a good candidate to mock. The SQLite is not quite reach SQL implementation from enterprise point of view. (source mode) Input Go source file; enables source mode. Thats because the go/bin folder is not in the PATH environment variable at the moment. So all we need to do is: make sure that the mock DB implements the same interface as the real DB. Built on Forem the open source software that powers DEV and other inclusive communities. prog.go:12:2: missing go.sum entry for module providing package github.com/golang/mock/mockgen/model; to add: