The function main () contains the object definition for an object of class type Demo. objects destructor for the Fraction class would be: Like the constructor, this function is called automatically (not explicitly). Select Accept to consent or Reject to decline non-essential cookies for this use. A constructor is called automatically when an object is created. And so each of the data members is initialized with this string object. It has three data members, animal type, animal name, animal sound, and there's three constructors and one destructor. First, we have our default constructor because it has no arguments in its parentheses. of type Display embedded inside of it, as components. things, if desired. Destructors in C++ are members functions in a class that delete an object. It also contains a function display () that prints the value of num1 and num2. An object allocated using the new operator is explicitly deallocated using delete. Destructors are different from normal member functions as they dont take any argument and dont return anything. It says copy constructor, and it says, clone-bob the goat says baah.
And you notice we have a class here called Animal. And this is after a colon, and before the beginning of the code block. constructors and destructors are invoked. And then we have our constructor with arguments. And again, after the colon, we have these initializers. Explanation: Destructors are used in Classes to destroy an object after its lifetime is over i.e. Now we have a constructor with three strings as its parameters. Example from Deitel that uses const member functions, Note: The term "has-a" comes from the natural way to speak about And these initializations are very common and very convenient, so you'll see them quite often. And it's just a simple way of constructing constructors. The destructor's typical job is to do any clean-up tasks (usually And so here we have constructor with arguments, bob the goat says baah, 'cause we've given it a type, a name, and a sound, and then we have an assignment constructor, or rather a copy constructor. Then we have an assignment operator and a simple print function, and we'll demonstrate these. object will be calling Display functions through the objects. 11.3.
And so we're initializing the values of type, name, and sound as unknown. be calling the timer's functions), The user of the Display objects is the Timer object (so the Timer This is the "has-a" relationship -- a Timer object "has" two objects to free resources occupied by that object. Two Display This is constructors.cpp from chapter six of the exercise files. And this is how the compiler knows that these are constructors. Learn more in our Cookie Policy. And then end of main, and then the three destructors clone-bob, bob the goat, and clone-clone-bob the goat. And then we have our destructor, which resets each of our strings. In Java, the garbage collector automatically deletes the unused objects to free up the memory. We construct one with the explicit strings, and we use the copy constructor, and we use the assignment operator. Destructor is called when instance of a class is deleted or released.
The destructor is explicitly called using the destructor functions fully qualified name. And you can have as many constructors as you want, as long as they have different function signatures. And if no constructors are specified at all, if we didn't have any of these, then a default constructor would be provided, an implicit default constructor would be provided by the compiler. And then we end, and then each of our objects will be destroyed. So you'll notice that we have three different constructors here.
As new functions are written, Whereas, Destructor on the other hand is used to destroy the class object. There are specific rules that make an overloaded delete function a destructor function: the function must have just one input argument, which is an object of the class, and it must not have any output arguments. This syntax of having the initializers after a colon and before the beginning of the code is only used in function members in class definitions. For more detail about constructors, please see the companion course C++ advanced topics. So it's name, type, and sound. The code snippet for this is given as follows. It is a special method that automatically gets called when an object is no longer used. Note the relationship between the classes. such a relationship. However, like a constructor, a destructor is a function and can do other So its sensible Java has no destructors available. And then we use an assignment operator to assign C back to A. whether a function, Test functionality frequently. If Car is a class, and it contains a We print out the name and the type of the animal. Constructors are special class functions which performs initialization of every object.
Answer: No, we cannot overload a destructor of a class in C++ programming. Destructors cannot have parameters, You can update your choices at any time in your settings. Destructors can freely call class member functions and access class member data.
There are several types of constructors in C++. A very good double-check mechanism, even Example: The And then a destructor is named with the name of the class with a tilde added at the beginning of the name, and this makes it a destructor. Declaration: And so if no arguments are specified, then it's a default constructor.
And then we simply put a string on the console that says we've called the default constructor, and we're just doing this for the purpose of demonstration, so that when we run this, we can see the construction and destruction of these objects. Download courses using your iOS or Android LinkedIn Learning app. Our second constructor takes three arguments, type, name, and sound, and our third constructor takes a reference to another animal object. There is also a destructor in Demo that is called when the scope of the class object is ended. How do I add music to my iPhone from another iTunes library? We construct an animal with the default constructor. And so we get that clone-bob, just to show that it's a copy. You'll remember up here at the top of the code, we have a string object called unk for unknown and another one called clone prefix, with the string clone- as its string. It cannot be declared static or const. And then after that, we call the print function, which says unknown, the unknown says unknown. Another example of classes in a "has-a" relationship: And we remember those from up here in our interface. These are pretty standard, and we'll talk in more detail about them later. Hence, overloading is also not possible. A program ends and global or static objects exist. The destructor looks like the default constructor (constructor with And our clone prefix, as you remember says, clone-. The compiler will enforce it. Constructor is Called when new instance of a class is created. And then there's our destructor and a couple of other function members. And you'll notice after a colon that there's this list of initializers. Compile and run this example to see when Developers have no need to mark the objects for deletion, which is error-prone and vulnerable to the memory leak. It's always worth thinking carefully about how your objects are constructed and crafting constructors and destructors that handle all the necessary use cases.
if the programmer was trying to be careful. And so here we have constructor with arguments for the string that's printed out when we call this constructor. Download the files the instructor uses to teach the course. involving memory allocation) that are needed, before an object is deallocated. The declaration shows the allowable use, and the user has assurance as to And so we're actually initializing each of these strings. And if we look up here at our copy constructor, we see that we append the clone prefix, plus the name in our initialization. Destructor destroys the objects when they are no longer needed. are used as member data in the Timer class declaration -- they are
And so let's look down here at the implementation of these constructors. It has no return type not even void. An object of a class with a Destructor cannot become a member of the union. And it prints out this destructor so we know which object is being destructed, or rather destroyed. Destructor in C++ neither takes any parameters nor does it return anything. Destructor function is automatically invoked when the objects are destroyed. by the system (usually when it goes out of scope). What is the destructor in Java? A destructor is called automatically right before an object is deallocated Download the exercise files for this course. - [Instructor] Constructors and destructors are special member functions that serve a particular purpose. Constructors initialize values to object members after storage is allocated to the object. We use cookies to ensure that we give you the best experience on our website. Constructors and destructors are important parts of any C++ class. *Price may change based on profile and billing country information entered during Sign In or Registration. so there can only be one destructor for a class. member data item of type Motor (another class), then it is natural Constructor is used to initialize the instance of a class. The Compiler calls the Constructor whenever an object is created. SodaMachine class. So we really have three objects here, A, which is constructed with the default constructor, B, which has the three strings, C, which is constructed with the copy constructor from B. So it's worth noting, and I'm just going to show you this one more time, because this is unique to constructors that you can use this initializer list to initialize members before or even without executing the body of a constructor function. Constructors are used to initialize and set up an object, and destructors are used to de-allocate and reset resources when an object is destroyed. to say "the Car obect has a Motor obect inside it".
Destructors in C++. And we do the same thing with the assignment operator. The lifetime of a temporary object ends. And so when we come back down here to our constructor implementation, you notice that we're initializing each of our data members, which are STL strings, with this unknown string. The first one is what's called a default constructor because it has no arguments. When an object completes its life-cycle the garbage collector deletes that object and deallocates or releases the memory occupied by the object. Good communication to the user of the class, function, variable, etc. no parameters), but with a ~ in front. them with calls in a, The user of the Timer is the main program (so the main program will Follow along and learn by watching, listening and practicing. The constructors are named with the name of the class. Watch courses on your mobile device without an internet connection. So first we have our default constructor, and you see the string default constructor. And this makes it a copy constructor because it will take that other animal object and copy its data over into this new object. And so we can watch each of these objects get destroyed. LinkedIn and 3rd parties use essential and non-essential cookies to provide, secure, analyze and improve our Services, and to show you relevant ads (including professional and job ads) on and off LinkedIn. They are called when the class object goes out of scope such as when the function ends, the program ends, a delete variable is called etc. So we assign it to A, with the assignment operator, and then we have clone-clone-Bob, because we're adding another clone at the beginning. They do not have their own name. And now here's our main. What is the role of destructors in Classes? And then we have our copy constructor, which initializes the data members like this, like in normal code. test named hours and minutes (these are variables). If you continue to use this site we will assume that you are happy with it. So, multiple destructor with different signatures are not possible in a class.
The destructor does not have arguments. So let's go ahead and run this, and we can watch what happens. Destructor is a member function which destructs or deletes an object.
And you notice we have a class here called Animal. And this is after a colon, and before the beginning of the code block. constructors and destructors are invoked. And then we have our constructor with arguments. And again, after the colon, we have these initializers. Explanation: Destructors are used in Classes to destroy an object after its lifetime is over i.e. Now we have a constructor with three strings as its parameters. Example from Deitel that uses const member functions, Note: The term "has-a" comes from the natural way to speak about And these initializations are very common and very convenient, so you'll see them quite often. And it's just a simple way of constructing constructors. The destructor's typical job is to do any clean-up tasks (usually And so here we have constructor with arguments, bob the goat says baah, 'cause we've given it a type, a name, and a sound, and then we have an assignment constructor, or rather a copy constructor. Then we have an assignment operator and a simple print function, and we'll demonstrate these. object will be calling Display functions through the objects. 11.3.
And so we're initializing the values of type, name, and sound as unknown. be calling the timer's functions), The user of the Display objects is the Timer object (so the Timer This is the "has-a" relationship -- a Timer object "has" two objects to free resources occupied by that object. Two Display This is constructors.cpp from chapter six of the exercise files. And this is how the compiler knows that these are constructors. Learn more in our Cookie Policy. And then end of main, and then the three destructors clone-bob, bob the goat, and clone-clone-bob the goat. And then we have our destructor, which resets each of our strings. In Java, the garbage collector automatically deletes the unused objects to free up the memory. We construct one with the explicit strings, and we use the copy constructor, and we use the assignment operator. Destructor is called when instance of a class is deleted or released.
The destructor is explicitly called using the destructor functions fully qualified name. And you can have as many constructors as you want, as long as they have different function signatures. And if no constructors are specified at all, if we didn't have any of these, then a default constructor would be provided, an implicit default constructor would be provided by the compiler. And then we end, and then each of our objects will be destroyed. So you'll notice that we have three different constructors here.
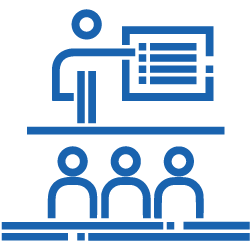
Answer: No, we cannot overload a destructor of a class in C++ programming. Destructors cannot have parameters, You can update your choices at any time in your settings. Destructors can freely call class member functions and access class member data.
There are several types of constructors in C++. A very good double-check mechanism, even Example: The And then a destructor is named with the name of the class with a tilde added at the beginning of the name, and this makes it a destructor. Declaration: And so if no arguments are specified, then it's a default constructor.
And then we simply put a string on the console that says we've called the default constructor, and we're just doing this for the purpose of demonstration, so that when we run this, we can see the construction and destruction of these objects. Download courses using your iOS or Android LinkedIn Learning app. Our second constructor takes three arguments, type, name, and sound, and our third constructor takes a reference to another animal object. There is also a destructor in Demo that is called when the scope of the class object is ended. How do I add music to my iPhone from another iTunes library? We construct an animal with the default constructor. And so we get that clone-bob, just to show that it's a copy. You'll remember up here at the top of the code, we have a string object called unk for unknown and another one called clone prefix, with the string clone- as its string. It cannot be declared static or const. And then after that, we call the print function, which says unknown, the unknown says unknown. Another example of classes in a "has-a" relationship: And we remember those from up here in our interface. These are pretty standard, and we'll talk in more detail about them later. Hence, overloading is also not possible. A program ends and global or static objects exist. The destructor looks like the default constructor (constructor with And our clone prefix, as you remember says, clone-. The compiler will enforce it. Constructor is Called when new instance of a class is created. And then there's our destructor and a couple of other function members. And you'll notice after a colon that there's this list of initializers. Compile and run this example to see when Developers have no need to mark the objects for deletion, which is error-prone and vulnerable to the memory leak. It's always worth thinking carefully about how your objects are constructed and crafting constructors and destructors that handle all the necessary use cases.
if the programmer was trying to be careful. And so here we have constructor with arguments for the string that's printed out when we call this constructor. Download the files the instructor uses to teach the course. involving memory allocation) that are needed, before an object is deallocated. The declaration shows the allowable use, and the user has assurance as to And so we're actually initializing each of these strings. And if we look up here at our copy constructor, we see that we append the clone prefix, plus the name in our initialization. Destructor destroys the objects when they are no longer needed. are used as member data in the Timer class declaration -- they are
And so let's look down here at the implementation of these constructors. It has no return type not even void. An object of a class with a Destructor cannot become a member of the union. And it prints out this destructor so we know which object is being destructed, or rather destroyed. Destructor in C++ neither takes any parameters nor does it return anything. Destructor function is automatically invoked when the objects are destroyed. by the system (usually when it goes out of scope). What is the destructor in Java? A destructor is called automatically right before an object is deallocated Download the exercise files for this course. - [Instructor] Constructors and destructors are special member functions that serve a particular purpose. Constructors initialize values to object members after storage is allocated to the object. We use cookies to ensure that we give you the best experience on our website. Constructors and destructors are important parts of any C++ class. *Price may change based on profile and billing country information entered during Sign In or Registration. so there can only be one destructor for a class. member data item of type Motor (another class), then it is natural Constructor is used to initialize the instance of a class. The Compiler calls the Constructor whenever an object is created. SodaMachine class. So we really have three objects here, A, which is constructed with the default constructor, B, which has the three strings, C, which is constructed with the copy constructor from B. So it's worth noting, and I'm just going to show you this one more time, because this is unique to constructors that you can use this initializer list to initialize members before or even without executing the body of a constructor function. Constructors are used to initialize and set up an object, and destructors are used to de-allocate and reset resources when an object is destroyed. to say "the Car obect has a Motor obect inside it".
Destructors in C++. And we do the same thing with the assignment operator. The lifetime of a temporary object ends. And so when we come back down here to our constructor implementation, you notice that we're initializing each of our data members, which are STL strings, with this unknown string. The first one is what's called a default constructor because it has no arguments. When an object completes its life-cycle the garbage collector deletes that object and deallocates or releases the memory occupied by the object. Good communication to the user of the class, function, variable, etc. no parameters), but with a ~ in front. them with calls in a, The user of the Timer is the main program (so the main program will Follow along and learn by watching, listening and practicing. The constructors are named with the name of the class. Watch courses on your mobile device without an internet connection. So first we have our default constructor, and you see the string default constructor. And this makes it a copy constructor because it will take that other animal object and copy its data over into this new object. And so we can watch each of these objects get destroyed. LinkedIn and 3rd parties use essential and non-essential cookies to provide, secure, analyze and improve our Services, and to show you relevant ads (including professional and job ads) on and off LinkedIn. They are called when the class object goes out of scope such as when the function ends, the program ends, a delete variable is called etc. So we assign it to A, with the assignment operator, and then we have clone-clone-Bob, because we're adding another clone at the beginning. They do not have their own name. And now here's our main. What is the role of destructors in Classes? And then we have our copy constructor, which initializes the data members like this, like in normal code. test named hours and minutes (these are variables). If you continue to use this site we will assume that you are happy with it. So, multiple destructor with different signatures are not possible in a class.
The destructor does not have arguments. So let's go ahead and run this, and we can watch what happens. Destructor is a member function which destructs or deletes an object.