In this chapter, we examine the role of type coercion in JavaScript. JavaScript TypeError - X.prototype.y called on incompatible type. When the string Rahul is added to the number 10 then JavaScript does not give an error. Come write articles for us and get featured, Learn and code with the best industry experts. The following sections describe the most important internal functions used by the ECMAScript specification to convert actual parameters to expected types. This can be observed if we use operations that set hint to 'default': The == operator coerces objects to primitives (with a default hint) if the other operand is a primitive value other than undefined, null, and boolean. and logical context. ToNumeric() is used by, among others, by the multiplication operator (*). Rule: all primitive types conversion happens to: string, number or boolean. TypeError: Cannot read property 'prop' of undefined, TypeError: Cannot read property 'prop' of null, TypeError: Cannot use 'in' operator to search for 'prop' in null, TypeError: Cannot convert a Symbol value to a number, TypeError: Cannot mix BigInt and other types, TypeError: Class constructor cannot be invoked without 'new', TypeError: (intermediate value) is not a constructor, TypeError: Cannot assign to read only property 'length', TypeError: Cannot assign to read only property 'prop'. Long canonical type. JavaScript has two ways to check if two values are equal: == (equals) and === (strict equals). requesting a Long or Ok. Type coercion is implicit type conversion: An operation automatically converts its arguments to the types it needs. Otherwise, it is converted to a value that has the desired type. Rule: When you operate string numbers with these operators then result will Number. What is the difference between CSS and SCSS ? If the field was can be used as the Type accepted by the Fields API as ToString() deviates in an interesting way from how String() works: If argument is a symbol, the former throws a TypeError while the latter doesnt. Type Coercion: Automatic conversion of a value from one data type to another data type is called Type Coercion or implicit type conversion. By using our site, you I would suggest having a look at the table shown on this page: Hope I have cleared confusion around Type Coercion in Javascript. Its specification looks as follows. expects its parameters to have certain types. How to return the data type of variable in JavaScript ? Built on Forem the open source software that powers DEV and other inclusive communities. How to auto-resize an image to fit a div container using CSS? I send out a short email each weekday on how to build a simpler, more resilient web. Since the array is empty, its value is 0. For example, in TypeScript, we would write: In the specification, this looks as follows (translated to JavaScript, so that it is easier to understand): Whenever primitive types or objects are expected, the following conversion functions are used: These internal functions have analogs in JavaScript that are very similar: After the introduction of bigints, which exists alongside numbers, the specification often uses ToNumeric() where it previously used ToNumber(). With you every step of your journey. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. Boolean to Number: When a Boolean is added to a Number, the Boolean value is converted to a number as it is safer and easier to convert Boolean values to Number values. especially the last one confuses many if they try to use it the first time. JavaScript: let isArray = Array.isArray(O), Spec: If O has a [[NumberData]] internal slot This is how Dates handle being converted to primitive values: The only difference with the default algorithm is that 'default' becomes 'string' (and not 'number'). But what if you used it with a number and a string? JavaScript had a choice between a string or a number and decided to use a string. JavaScript initially didnt have exceptions, which is why it uses coercion and error values for most of its operations: However, there are also cases (especially when it comes to newer features) where it throws exceptions if an argument doesnt have the right type: Accessing properties of null or undefined: New-calling or function-calling values that dont support that operation: Changing read-only properties (only throws in strict mode): Two common ways of dealing with coercion are: A caller can explicitly convert values so that they have the right types. JavaScript: if ('__NumberData__' in O), Spec: Let tag be Get(O, @@toStringTag) How to create footer to stay at the bottom of a Web page? Declaimer: Javascript supports explicit conversion as well and my topic is not that hence I am not covering any of its content though it is closely related. DEV Community 2016 - 2022. As a best practice, I recommend that you use the JavaScripts native type conversion methods to force values into their desired format if youve ever not sure what the value might be. There are a few things that make coercion looks hard to remember. CoercibleType extends date value, DateType can be used to declare its Made with in Massachusetts. Read on for more information. The operation ToPrimitive() is an intermediate step for many coercion algorithms (some of which well see later in this post). Some examples of this are shown below: Writing code in comment? The following list shows how some frequently used patterns are translated from specification to JavaScript: Spec: If Type(value) is String // Create a new String instance that has the given prototype, // Comparing a boolean with a non-boolean, // (other than undefined, null, a boolean), How internal type conversion functions are used in the ECMAScript specification, Converting to primitive types and objects, Intermission: expressing specification algorithms in JavaScript, Glossary: terms related to type conversion. In this example, the items are considered equal. To return this result, you'd have to explicitly convert the 5 to a number using the Number() method: Last modified: Oct 8, 2021, by MDN contributors. Huh? The compiler could have coerced the 5 into a number and returned a sum of 14, but it did not. How to Upload Image into Database and Display it using PHP ? Some things are omitted for example, the ReturnIfAbrupt shorthands ? String to Number Conversion: When an operation like subtraction (-), multiplication (*), division (/) or modulus (%) is performed, all the values that are not number are converted into the number data type, as these operations can be performed between numbers only. In the above example, JavaScript has coerced the 9 from a number into a string and then concatenated the two values together, resulting in a string of 59. concatenation. Or more simply, The helper function StringCreate() and SymbolDescriptiveString() are shown next. Spec: Return the string-concatenation of [object ", tag, and "]. In addition to String(), you can also use method .toString() to convert a symbol to a string. This is how it works: Once again, objects are converted to primitives before working with primitives. == Equality operator: Used to compare values irrespective of their types. converting a Long 42 to a much more efficient. All of them have answers and nothing returns an error. It converts an arbitrary values to primitive values. If one of the results is a string, both are converted to strings and concatenated (line A). DEV Community A constructive and inclusive social network for software developers. This is what the JavaScript version of ToPrimitive() looks like: ToPrimitive() lets objects override the conversion to primitive via Symbol.toPrimitive (line A). Anything below you can read out of your interest. If an object doesnt do that, it is passed on to OrdinaryToPrimitive(): The parameter hint can have one of three values: These are a few examples of how various operations use ToPrimitive(): As we have seen, the default behavior is for 'default' being handled as if it were 'number'. It uses new.target to distinguish the two. Sign up today and get 25% off registration. This is how it works: Once again, objects are converted to primitives before working with primitives. Some things are omitted for example, the ReturnIfAbrupt shorthands ? acknowledge that you have read and understood our, GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Top 10 Projects For Beginners To Practice HTML and CSS Skills. Coercion of bitwise operators for numbers (BigInt operators dont limit the number of bits). Once suspended, urstrulyvishwak will not be able to comment or publish posts until their suspension is removed. JavaScript again coerces the array into a string, 1,2,3. maintains the value of the field as a long Its specification looks as follows. Cascading supports primitive type The default specification for .toString() looks as follows: This operation is used if we convert plain objects to strings: By default, it is also used if we convert instances of classes to strings: Normally, we would override .toString() in order to configure the string representation of MyClass, but we can also change what comes after object inside the string with the square brackets: It is interesting to compare the overriding versions of .toString() with the original version in Object.prototype: ToPropertyKey() is used by, among others, the bracket operator. How to update Node.js and NPM to next version ? Otherwise, both operands are converted to numeric values and added (line B). java.lang.reflect.Type. Lets step away from the buzzwords for a second and demystify this shit! Templates let you quickly answer FAQs or store snippets for re-use. JavaScript: if (TypeOf(value) === 'string') ToInt32(), ToUint32() coerce numbers to 32-bit integers and are used by bitwise operators (see tbl. Did you ever use this type of validation in your code? Operators /, -, *, %: Division, Subtraction, Multiplication, Modulus in order.
Some more examples are shown below. In the following interaction, we can see that the result of coercing the date is a string: The + operator coerces both operands to primitives (with a default hint). Or when data is set on a Tuple via That is only done twice in the standard library: This is how Dates handle being converted to primitive values: The only difference with the default algorithm is that 'default' becomes 'string' (and not 'number'). JavaScript coerces the string into a number and increments it by 1. I also very irregularly share non-codingthoughts. value of "someFields" will be coerced into its canonical form, Made with love and Ruby on Rails. For example, in the following interaction, we want to multiply two numbers that are written out in strings: They can let the operation make the conversion for them: Computed property keys in object literals, Spec: If O has a [[NumberData]] internal slot. It also includes ++ and -- (to increment and decrement numbers), and a bunch of others, too. I don't think this question won't be a deciding factor for the selection.. In the following interaction, we can see that the result of coercing the date is a string because the operator returns a string. Type coercion can result in some weird ass shit in JavaScript. Updated on Mar 1. 4. TupleEntry.getLong() or Explicit conversions can be: What type casting is, depends on the programming language. Here are the examples. String representation of a field, via Rule: == operator coerces to number by default except in case of null. DateType Type coercion can be useful, but its also really dangerous. format allowing TextDelimited to read and write If one of the results is a string, it performs string concatenation (otherwise it performs numeric addition). File Type Validation while Uploading it using JavaScript. Rule: 0, -0, undefined, null, '', false, NaN are falsy as per Javascript engine any other thing is true. Type coercion is a means to convert one data type to another. Some examples of this are shown below. If a value doesnt have the right type for a parameter, three common options for, e.g., a function are: The function can convert its arguments to useful values: In (3), the operation performs an implicit type conversion. Read on for more information. What if the interviewer asks this question? How to set different type of cursors using CSS ? JavaScript has two ways to check if two values are equal. Strict equals (===) check that items have both the same value and the same data type. How to get values from html input array using JavaScript ? Both operands are converted to primitive values. internally (in the Tuple) as a long, which is TextDelimited CSV file, where one column is a JavaScript: if (IsCallable(method)) For further actions, you may consider blocking this person and/or reporting abuse. The default for symbols is that converting them to strings throws exceptions: That default is overridden in String() and Symbol.prototype.toString() (both are described in the next sections): In line A, we can see what happens if String() is function-called and its argument is a symbol. Under-the-hood, JavaScript uses something called type coercion to automatically convert a value from one data type to another when using some sort of operator with it. - According to Google. coercions natively through the How to remove borders of a canvas-type text using Fabric.js ? Make immutable pop(), push(), shift(), unshift(). Why is that?
DateType. You don't really need to remember any of the below scenarios. jQuery | event.type property with Examples, Semantic-UI Advertisement Leaderboard Type, Complete Interview Preparation- Self Paced Course. For example, in the following interaction, we want to multiply two numbers encoded as strings: A caller can let the operation make the conversion for them: I usually prefer the former, because it clarifies my intention: I expect x and y not to be numbers, but want to multiply two numbers. cascading.tuple.TupleEntry interface by If urstrulyvishwak is not suspended, they can still re-publish their posts from their dashboard. when different types of operators are applied to the values. The range of the result is often restricted further afterwards. It converts the number 10 to string 10 using coercion and then concatenates both the strings. 2. Instead of returning an error, it will do type coercion in the following scenarios: Not to worry if you don't get the above points instantly, I would definitely make you remember forever by end of this article. For example: In this example we declare the "dateString" field to be a There are many weird scenarios around different operators that result in different results. JavaScript: let tag = O[Symbol.toStringTag]. The default for symbols is that converting them to strings throws exceptions: That default is overridden in String() and Symbol.prototype.toString() (both are described in the next subsections): String() works differently, depending on whether it is invoked via a function call or via new. How to check the type of a variable or object in JavaScript ? Unless otherwise noted, all code is free to use under the MIT License. Spec: Return the string-concatenation of "[object ", tag, and "]". What would you expect the result of num to be in this example? How to set fixed width for in a table ? That is called type coercion. internally, but if a String is set or requested, Please do comment if I miss any valid coercion examples. Explicit type conversion means that the programmer uses an operation (a function, an operator, etc.) Hence, the Javascript engine does the same in converting the value from one type to another without your intervention. How to apply style to parent if it has child with CSS? Type coercion is the automatic or implicit conversion of values from one data type to another (such as strings to numbers). Once unpublished, this post will become invisible to the public How to place text on image using HTML and CSS? How to check a variable is of function type using JavaScript ? declared as an Integer, and In JavaScript, you can use + to add two numbers together. (IsCallable() is defined below), Spec: Let numValue be ToNumber(value) Otherwise, both operands are converted to numeric values and added (line B). For example, if youre adding numbers that came from an API or a user-submitted form, run them through parseFloat() to convert any strings into numbers first. In the example below, the items are not equal because one is a string and the other is a number. CSS to put icon inside an input element in a form. TupleEntry.setString( "someField", "42" ) was called, the Personally, I wish strict type checking was the default in JavaScript.
How to reset input type = "file" using JavaScript/jQuery? You can also use it to join two strings. Type coercion is implicit type conversion: An operation automatically converts its arguments to the types it needs. Rule: If any operand is a string and operated with + then the result is always concatenated and the result is a string. 1). Both operands are converted to primitive values. Happens with logical operators (||, && and !) How to check if the provided value is of the specified type in JavaScript ? Type Coercion refers to the process of automatic or implicit conversion of values from one data type to another. Copyright 2007-2012 Concurrent, Inc. All Rights Reserved. The operation ToPrimitive() is an intermediate step for many coercion algorithms (some of which well see later in this chapter). I still think its overcomplicated, though!). SimpleDateFormat String This also does some other work when used with strings i.e. This is how it works: The structure of ToNumber() is similar to the structure of ToString(). Only instances of Symbol and Date override this behavior (shown later). In the statement below, + is the operator. Similarly, if youre trying to create a string and theres a possibility the values are actually numbers, use the toString() method to explicitly convert them to strings. If one of the results is a string, both are converted to strings and concatenated (line A). Coercion generally means the practice of persuading someone to do something by using force or threats. To create custom coercions, the It converts an arbitrary values to primitive values. Thanks, Google. This includes conversion from Number to String, String to Number, Boolean to Number etc. TypeError: Cannot convert a Symbol value to a string, // This function was function-called and value is a symbol, * Creates a String instance that wraps `value`, // Comparing a boolean with a non-boolean, // (other than undefined, null, a boolean), Operations that help implement coercion in the ECMAScript specification, Converting to primitive types and objects, Intermission: expressing specification algorithms in JavaScript, Glossary: terms related to type conversion, Computed property keys in object literals. Type conversion is similar to type coercion because they both convert values from one data type to another with one key difference type coercion is implicit whereas type conversion can be either implicit or explicit. Double 42.0. A Boolean value can be represented as 0 for false or 1 for true. ToPropertyKey() returns a string or a symbol and is used by: When we set the value of a Typed Array element, one of the following conversion functions is used: In the remainder of this chapter, well encounter several specification algorithms, but implemented as JavaScript. JavaScript: let numValue = Number(value), Spec: Let isArray be IsArray(O) TupleEntry.setString(), for example. This is what the JavaScript version of ToPrimitive() looks like: ToPrimitive() lets objects override the conversion to primitive via Symbol.toPrimitive. Making a div vertically scrollable using CSS.
In case the behavior of the implicit conversion is not sure, the constructors of a data type can be used to convert any value to that datatype, like the Number(), String() or Boolean() constructor. How to convert JSON string to array of JSON objects using JavaScript ? JavaScript: return '[object ' + tag + ']'; let (and not const) is used to match the language of the specification. Explicit type conversion means that the programmer uses an operation (a function, an operator, etc.) Similarly, greater than/less than operators will coerce strings into numbers to check their relative value. String to Number Conversion: When any string or non-string value is added to a string, it always converts the non-string value to a string implicitly. Regular equals (==) uses type coercion to compare the values of the items, ignoring their data type. allow for coercions between date strings and the If this sort of stuff is interesting to you, you can find a whole ton of examples on JavaScript WTF. cascading.tuple.type.CoercibleType interface must For How to select all child elements recursively using CSS? Rule: In all the ways, the exposed object or variable is coerced to a string. We will go relatively deeply into this subject and, e.g., look into how the ECMAScript specification handles coercion. and !. These are the helper functions StringCreate() and SymbolDescriptiveString(): In addition to String(), we can also use method .toString() to convert a symbol to a string. Best Practice: Always use === instead of ==. They can explicitly convert the value so that it has the right type. JavaScript coerces the number into a string, and joins. Explicit conversions can be: What type casting is, depends on the programming language. MDN Web Docs Glossary: Definitions of Web-related terms. example, parsing the Java String "42" to the Hate the complexity of modern frontend web development? That is where coercion comes in to picture. They can still re-publish the post if they are not suspended. TupleEntry.getString(), respectively. (very loose translation; TypeOf() is defined below), Spec: If IsCallable(method) is true How to position a div at the bottom of its container using CSS? ToNumeric() returns a numeric value num. It can cause your code to behave in unexpected ways. it will be converted using the given Let's see where and when this conversion happens and what to remember while coding. Most probably, questions asked in the following way: 1+2+'str' -> 3 + 'str' -> 3str -> first two are numbers hence added and as per string coercion second part is concatenated. Each operation (function, operator, etc.) ToPrimitive() is used often in the spec because many operations (eventually) only work with primitive values. What is void and when to use void type in JavaScript ? The default specification for .toString() looks as follows: This operation is used if you convert plain objects to strings: By default, it is also used if you convert instances of classes to strings: You can configure what comes after object inside the square brackets: If you call Object.prototype.toString directly, you can access the overridden behavior: ToPropertyKey() is used by, among others, the bracket operator. one little mistake in your first example: console.log('str' + 1); // strstr. For example, in Java, it is explicit checked type conversion. This is how JavaScripts addition operator is specified: The following operations are not shown here: Now that we have taken a closer look at how JavaScripts type coercion works, lets conclude with a brief glossary of terms related to type conversion: In type conversion, we want the output value to have a given type. Join over 13k others. and !. This is how JavaScripts addition operator is specified: The following operations are not shown here: Now that we have taken a closer look at how JavaScripts type coercion works, lets conclude with a brief glossary of terms related to type conversion: In type conversion, we want the output value to have a given type. Only instances of Symbol and Date override this behavior. Once unsuspended, urstrulyvishwak will be able to comment and publish posts again. How to insert spaces/tabs in text using HTML/CSS? generate link and share the link here. Its callers usually invoke a method mthd of the specification type of num: Among others, the following operations use ToNumeric: ToInteger(x) is used whenever a number without a fraction is expected. In practice, developers implicitly invoke coercions via the It forces strict type in your code. A new session of the Vanilla JS Academy started this week, but it's not too late to join. ToPropertyKey() returns a string or a symbol and is used by: When you set the value of a Typed Array element, one of the following conversion functions is used: In the remainder of the post, well encounter several specification algorithms, but implemented as JavaScript. Operator +: By default used for adding numbers. It then treats the + as a join operator, and coerces the 4 into a string. How to set div width to fit content using CSS ? Posted on Feb 18 'str'+1+2 - str1 + 2 -> str12 - You might have understood. Sign up today and get 25% off registration. The Equality Operator: The equality operator (==) can be used to compare values irrespective of their type. * string, number, or dont care? Once unpublished, all posts by urstrulyvishwak will become hidden and only accessible to themselves. Last Chance! If one or both operands are non-numeric then result will be NaN. the value as a String, but use the value expects its parameters to have certain types. null is always equal to null or undefined.


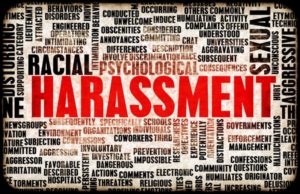
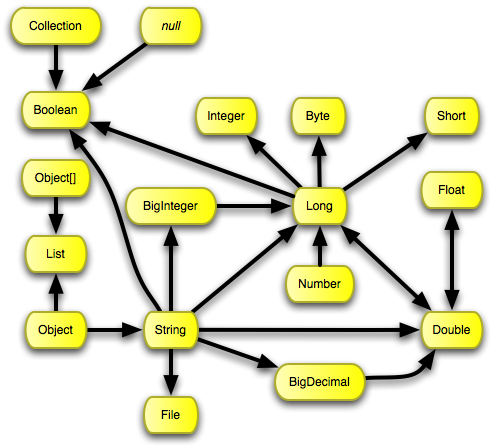