The new class created is called derived class or child class and the existing class is known as the base class or parent class. }; Example: Why do we use constructors in C#? The main use of constructors is to initialize the private fields of the class while creating an instance for the class. When you have not created a constructor in the class, the compiler will automatically create a default constructor of the class. 17.4 Constructors and initialization of derived classes. If two classes form an inheritance hierarchy, then when an instance of a derived class is created, the constructor of the base class is first called, which constructs the object of the derived class.
as single,multiple,multilevel or Hierarchical inheritances. If there is a virtual inheritance then it's given higher preference). A constructor cannot be static, abstract, final or synchronized. Constructors in Java are used to initialize the values of the attributes of the object serving the goal to bring Java closer to the real world. Instead of inheriting constructors by the derived class, it is only allowed to invoke the constructor of base class. Below is the source code for C++ Program to illustrates the use of Constructors in multilevel inheritance which is successfully compiled and run on Windows System to produce desired output as shown below : Derived class can not inherit the base class constructor but it can call the base class constructor by using. Instead of writing a complete constructor, you just inherit the base class constructors and are ready to use them: class Derived : public Base { using Base::Base; }; cpp call base constructor syntax.
The following program demonstrates the order of constructors in inheritance. cpp call base constructor syntax. They are called implicitly or explicitly by the child constructor. i.e. The object created by the constructors is fully initialized as soon as any constructor is finished. Step 5: Perform s=s+a and i=i+1. #include. I'm replicating what I have in the Base Account because I don't know which other way to create the Saving Account by using the setters/getters.
This article briefly explains inheritance and the types of inheritance. Constructor is a class member function with the same name as the class name.
Well, Scott Meyers does discuss simulatiom of virtual constructors in More Effective C++ (Item 25). Constructors are not called automatically.
Multiple Inheritance: Multiple Inheritance is a feature of C++ where a class can derive from several (two or more) base classes. It only contains constructors it defines. Step 4: Take a number a an as input. base class constructor call c++. You can specify which of several base class constructors should be called during the creation of a derived class object. using System; The name and return type are automatically the same, both being constructors, and you made both the paramters to them the same, void. using System; Constructors and Inheritance Problem. It allows you to define a child class that reuses (inherits), extends, or modifies the behavior of a parent class.
This is straight from Bjarne Stroustrup's page : If you so choose, you can still shoot yourself in the foot by inheriting constructors in a derive How constructors are different from a normal member function? In most cases, this makes sense. The following program demonstrates the order of constructors in inheritance. Step 3: set s=0 and i=0. For more information, see Delegating Constructors. {
};
derived class inherits all the members(fields and methods) of the base class, but derived class cannot inherit the constructor of the base class be }; It is very important to understand how constructors are called in inheritance. Constructor is automatically called when the object is created. In C++, derived classes cannot inherit the constructors of their base classes -- nor can they inherit assignment operators. We already have a default constructor that is called automatically if no constructor is found in the code. The syntax define constructors in multiple inheritance with arguments is also similar to the definition of single inheritance with arguments. Inheritance is almost like embedding an object into a class. This includes the inherited members." This is because constructors are not members of the class, they are just use initialize the object values. The class whose members are inherited is called the base class. Instead of writing a complete constructor, you just inherit the base class constructors and are ready to use them: class Derived : public Base { using Base::Base; }; We have a Base class Base which has a default constructor and a parameterized constructor. 4. constructors in inheritance in c++. Instead of writing a complete constructor, you just inherit the base class constructors and are ready to use them: class Derived : public Base { using Base::Base; }; Hi, I'm trying to create a Saving Account derived from a Base Account using only setters and getters. I'm replicating what I have in the Base Account because I don't know which other way to create the Saving Account by using the setters/getters. C# and .NET support single inheritance only. That is, a class can only inherit from a single class. However, inheritance is transitive, which allows you to define an inheritance hierarchy for a set of types. In other words, type D can inherit from type C, which inherits from type B, which inherits from the base class type A. Destructors are called in reverse order, right to left. Here are some problems if constructors were inherited in C++. Figure 1. They are called implicitly or explicitly by the child constructor. If there are multiple base classes then, construction starts with the leftmost base. elements. Constructors of both classes must be executed when the object of child class is created.
We have a Base class Base which has a default constructor and a parameterized constructor. Instead of inheriting constructors by the derived class, it is only allowed to invoke the constructor of base class. It cannot be overridden. 1+2=. Constructor of A Constructor of B Constructor of C Constructor of D Destructor of D Destructor of C Destructor of B Destructor of A .
class C:public A,public B. Answer (1 of 2): Constructors do not follow the notion of inheritance themselves but they do respect the rules of inheritance defined for their classes. class C:public A,public B. Constructors can be defined either inside the class definition or outside class definition using class name and scope resolution :: operator. or B() : A(5) { } But if we need to call the base class constructor, then we need to call it explicitly in the derived classs constructor. Constructors can also take parameters (just like regular functions), which can be useful for setting initial values for attributes. The compiler creates a default constructor (one with no arguments) and a default copy constructor (one with an argument which is a reference to the same type). From the inheritance point of view constructors are not derived, so derived class does not have same constructors as base class has. As you can see, constructors are called in order of derivation, left to right, as specified in derived's inheritance list. The compilers default action is to call the default constructor in the base class. If there is a virtual inheritance then it's given higher preference). For more information, see Delegating Constructors. The following class have brand, model and year attributes, and a constructor with different parameters. when class is inheriting a base class then the base class's constructor is called first then derived class's ,and the
cpp call constructor of base class. Why are constructors not inherited: the answer is surprisingly simple: The constructor of the base class "builds" the base class and the constructor of the inherited class "builds" the inherited class. It cannot be overridden. explicit A(int x) {} The class that inherits the members of the base class is called the derived class. Constructor is a class member function with the same name as the class. Inheritance is a feature or a process in which, new classes are created from the existing classes. Answer (1 of 5): If constructors were inherited in C++, it would cause many undesirable problems, and the main benefit of inheritance of members would not apply to constructors. Then the member fields are constructed. It allows you to define a child class that reuses (inherits), extends, or modifies the behavior of a parent class.
In this post, we will write a C++ program to inherit the derived constructor. It is special member function of the class. In inheritance, the derived class inherits all the members (fields, methods) of the base class, but derived class cannot inherit the constructor of the base class because constructors are not the members of the class. Constructors are not inherited. 4 Inheritance : Hybrid inheritance is combination of two or more inheritances such.
Constructor Inheritance in C++ We can see from the previous example that the functions and properties are inherited in the derived class as it is from the base class. Then the member fields are constructed. It only contains constructors it defines. Constructors can be defined either inside the class definition or outside class definition using class name and scope resolution :: operator. Constructor is automatically called when the object is created. Overloaded operators of the base class. Base class constructors are executed first ,before the derived class constructors execution.
6. If we want to know the sequence of Invocation of constructors and destructors, then it is important to know that it depends on the type of inheritance being used.
Constructor Inheritance in C++ We can see from the previous example that the functions and properties are inherited in the derived class as it is from the base class. Base_class name::base_class_constructor () in derived class definition. The main job of the constructor is to allocate memory for class objects. Constructor is a class member function with the same name as the class. The friend functions of the base class. 1. special_item s = special_item (); A function's signature comprises of it's name, it's parameters, and it's return type. It is very important to understand how constructors are called in inheritance. Example c++ call base class constructor from derived class constructor body. Constructors, destructors and copy constructors of the base class. The last to be called is the constructor of class C, which is at the bottom of the hierarchy. You can specify which of several base class constructors should be called during the creation of a derived class object. In the definition of a constructor of a class, member initializer list specifies the initializers for direct and virtual bases and non-static data members. You can specify which of several base class constructors should be called during the creation of a derived class object. Example The diamond problem occurs when a subclass inherits from more than one base class which in turn are inherited from a single base class. First: T4Tutorials class constructor. If there are multiple base classes then, construction starts with the leftmost base. The object created by the constructors is fully initialized as soon as any constructor is finished. In the past two lessons, weve explored some basics around inheritance in C++ and the order that derived classes are initialized. Why must we call the base class constructor explicitly for it to work? Constructor is a special non-static member function of a class that is used to initialize objects of its class type. What is the use of private constructor in C#?It is used to stop object creation of a class.It is used to stop a class to be inherited.It is used in singleton design patterns, to make sure that the only one instance of a class can ever be created. Derived class can not inherit the base class constructor but it can call the base class constructor by using. Constructors and Inheritance Problem. c++ calling the constructor of base class. Just remove the constructor with the empty body most likely. Step 1: Start the program. The constructor calling order will be top to bottom.
calling a base constructor C++. Then the member fields are constructed. But you can use the parent's constructor in the child's constructor Sword () : Item (3) // Call the superclass constructor and set damage to 3 { // do something } Share Improve this answer Inheriting Constructors. Answer (1 of 5): If constructors were inherited in C++, it would cause many undesirable problems, and the main benefit of inheritance of members would not apply to constructors. Step 3: set s=0 and i=0. The main job of the constructor is to allocate memory for class objects. The constructor of the derived class calls the constructors of the base classes in the same order in which they are specified in the header of the derived class. Derived class can not inherit the base class constructor but it can call the base class constructor by using.
1. special_item s = special_item (); A function's signature comprises of it's name, it's parameters, and it's return type. }; This is because constructors are not members of the class, they are just use initialize the object values. Correct Code is class A Inheritance and Constructors in Java. Constructors are not called automatically. { Method overriding in C# inheritance Method overriding is a feature that allows to invoke child class method having same name and signature as in base class method in inheritance hierarchy if we use base class reference. In below c# inheritance source code , child class B overrides func () method of base class using override keyword and to
A subclass must declare its own constructors.
It is executed when an instance of the class is created. The name and return type are automatically the same, both being constructors, and you made both the paramters to them the same, void. The name and return type are automatically the same, both being constructors, and you made both the paramters to them the same, void. In a hierarchy, it is possible for both base classes and derived classes to have their own constructors. Correct Code is class A You can specify which of several base class constructors should be called during the creation of a derived class object.
Constructor call in multiple inheritance constructors class C: public A, public B; Constructors are called upon the order in which they are inherited First class A constructors are executed followed by class B constructors, then class C constructors Quiz Time Question 1. The reason for this is that when you write a constructor for an object of a derived class, you are responsible for ensuring that the members of the derived class object are properly initialized. Step 5: Perform s=s+a and i=i+1. calling a base constructor C++. The compilers default action is to call the default constructor in the base class. Method overriding in C# inheritance Method overriding is a feature that allows to invoke child class method having same name and signature as in base class method in inheritance hierarchy if we use base class reference. In below c# inheritance source code , child class B overrides func () method of base class using override keyword and to 1. special_item s = special_item (); A function's signature comprises of it's name, it's parameters, and it's return type.
as single,multiple,multilevel or Hierarchical inheritances. If there is a virtual inheritance then it's given higher preference). A constructor cannot be static, abstract, final or synchronized. Constructors in Java are used to initialize the values of the attributes of the object serving the goal to bring Java closer to the real world. Instead of inheriting constructors by the derived class, it is only allowed to invoke the constructor of base class. Below is the source code for C++ Program to illustrates the use of Constructors in multilevel inheritance which is successfully compiled and run on Windows System to produce desired output as shown below : Derived class can not inherit the base class constructor but it can call the base class constructor by using. Instead of writing a complete constructor, you just inherit the base class constructors and are ready to use them: class Derived : public Base { using Base::Base; }; cpp call base constructor syntax.
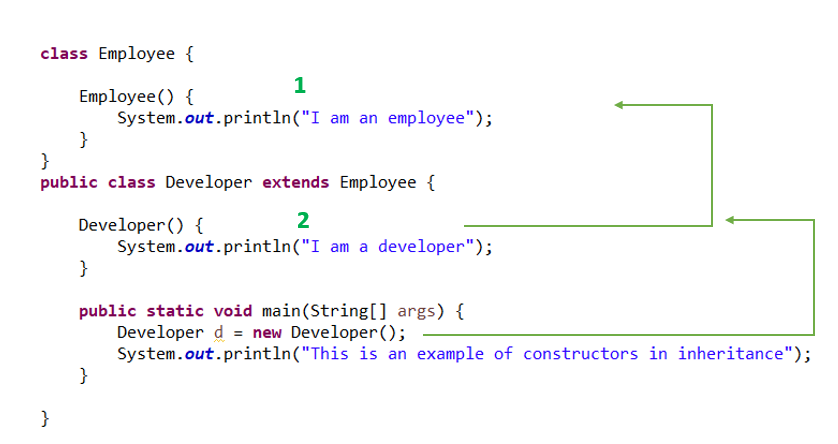
This article briefly explains inheritance and the types of inheritance. Constructor is a class member function with the same name as the class name.
Well, Scott Meyers does discuss simulatiom of virtual constructors in More Effective C++ (Item 25). Constructors are not called automatically.
Multiple Inheritance: Multiple Inheritance is a feature of C++ where a class can derive from several (two or more) base classes. It only contains constructors it defines. Step 4: Take a number a an as input. base class constructor call c++. You can specify which of several base class constructors should be called during the creation of a derived class object. using System; The name and return type are automatically the same, both being constructors, and you made both the paramters to them the same, void. using System; Constructors and Inheritance Problem. It allows you to define a child class that reuses (inherits), extends, or modifies the behavior of a parent class.
This is straight from Bjarne Stroustrup's page : If you so choose, you can still shoot yourself in the foot by inheriting constructors in a derive How constructors are different from a normal member function? In most cases, this makes sense. The following program demonstrates the order of constructors in inheritance. Step 3: set s=0 and i=0. For more information, see Delegating Constructors. {
};
derived class inherits all the members(fields and methods) of the base class, but derived class cannot inherit the constructor of the base class be }; It is very important to understand how constructors are called in inheritance. Constructor is automatically called when the object is created. In C++, derived classes cannot inherit the constructors of their base classes -- nor can they inherit assignment operators. We already have a default constructor that is called automatically if no constructor is found in the code. The syntax define constructors in multiple inheritance with arguments is also similar to the definition of single inheritance with arguments. Inheritance is almost like embedding an object into a class. This includes the inherited members." This is because constructors are not members of the class, they are just use initialize the object values. The class whose members are inherited is called the base class. Instead of writing a complete constructor, you just inherit the base class constructors and are ready to use them: class Derived : public Base { using Base::Base; }; We have a Base class Base which has a default constructor and a parameterized constructor. 4. constructors in inheritance in c++. Instead of writing a complete constructor, you just inherit the base class constructors and are ready to use them: class Derived : public Base { using Base::Base; }; Hi, I'm trying to create a Saving Account derived from a Base Account using only setters and getters. I'm replicating what I have in the Base Account because I don't know which other way to create the Saving Account by using the setters/getters. C# and .NET support single inheritance only. That is, a class can only inherit from a single class. However, inheritance is transitive, which allows you to define an inheritance hierarchy for a set of types. In other words, type D can inherit from type C, which inherits from type B, which inherits from the base class type A. Destructors are called in reverse order, right to left. Here are some problems if constructors were inherited in C++. Figure 1. They are called implicitly or explicitly by the child constructor. If there are multiple base classes then, construction starts with the leftmost base. elements. Constructors of both classes must be executed when the object of child class is created.
We have a Base class Base which has a default constructor and a parameterized constructor. Instead of inheriting constructors by the derived class, it is only allowed to invoke the constructor of base class. It cannot be overridden. 1+2=. Constructor of A Constructor of B Constructor of C Constructor of D Destructor of D Destructor of C Destructor of B Destructor of A .
class C:public A,public B. Answer (1 of 2): Constructors do not follow the notion of inheritance themselves but they do respect the rules of inheritance defined for their classes. class C:public A,public B. Constructors can be defined either inside the class definition or outside class definition using class name and scope resolution :: operator. or B() : A(5) { } But if we need to call the base class constructor, then we need to call it explicitly in the derived classs constructor. Constructors can also take parameters (just like regular functions), which can be useful for setting initial values for attributes. The compiler creates a default constructor (one with no arguments) and a default copy constructor (one with an argument which is a reference to the same type). From the inheritance point of view constructors are not derived, so derived class does not have same constructors as base class has. As you can see, constructors are called in order of derivation, left to right, as specified in derived's inheritance list. The compilers default action is to call the default constructor in the base class. If there is a virtual inheritance then it's given higher preference). For more information, see Delegating Constructors. The following class have brand, model and year attributes, and a constructor with different parameters. when class is inheriting a base class then the base class's constructor is called first then derived class's ,and the
cpp call constructor of base class. Why are constructors not inherited: the answer is surprisingly simple: The constructor of the base class "builds" the base class and the constructor of the inherited class "builds" the inherited class. It cannot be overridden. explicit A(int x) {} The class that inherits the members of the base class is called the derived class. Constructor is a class member function with the same name as the class. Inheritance is a feature or a process in which, new classes are created from the existing classes. Answer (1 of 5): If constructors were inherited in C++, it would cause many undesirable problems, and the main benefit of inheritance of members would not apply to constructors. Then the member fields are constructed. It allows you to define a child class that reuses (inherits), extends, or modifies the behavior of a parent class.
In this post, we will write a C++ program to inherit the derived constructor. It is special member function of the class. In inheritance, the derived class inherits all the members (fields, methods) of the base class, but derived class cannot inherit the constructor of the base class because constructors are not the members of the class. Constructors are not inherited. 4 Inheritance : Hybrid inheritance is combination of two or more inheritances such.
Constructor Inheritance in C++ We can see from the previous example that the functions and properties are inherited in the derived class as it is from the base class. Then the member fields are constructed. It only contains constructors it defines. Constructors can be defined either inside the class definition or outside class definition using class name and scope resolution :: operator. Constructor is automatically called when the object is created. Overloaded operators of the base class. Base class constructors are executed first ,before the derived class constructors execution.
6. If we want to know the sequence of Invocation of constructors and destructors, then it is important to know that it depends on the type of inheritance being used.
Constructor Inheritance in C++ We can see from the previous example that the functions and properties are inherited in the derived class as it is from the base class. Base_class name::base_class_constructor () in derived class definition. The main job of the constructor is to allocate memory for class objects. Constructor is a class member function with the same name as the class. The friend functions of the base class. 1. special_item s = special_item (); A function's signature comprises of it's name, it's parameters, and it's return type. It is very important to understand how constructors are called in inheritance. Example c++ call base class constructor from derived class constructor body. Constructors, destructors and copy constructors of the base class. The last to be called is the constructor of class C, which is at the bottom of the hierarchy. You can specify which of several base class constructors should be called during the creation of a derived class object. In the definition of a constructor of a class, member initializer list specifies the initializers for direct and virtual bases and non-static data members. You can specify which of several base class constructors should be called during the creation of a derived class object. Example The diamond problem occurs when a subclass inherits from more than one base class which in turn are inherited from a single base class. First: T4Tutorials class constructor. If there are multiple base classes then, construction starts with the leftmost base. The object created by the constructors is fully initialized as soon as any constructor is finished. In the past two lessons, weve explored some basics around inheritance in C++ and the order that derived classes are initialized. Why must we call the base class constructor explicitly for it to work? Constructor is a special non-static member function of a class that is used to initialize objects of its class type. What is the use of private constructor in C#?It is used to stop object creation of a class.It is used to stop a class to be inherited.It is used in singleton design patterns, to make sure that the only one instance of a class can ever be created. Derived class can not inherit the base class constructor but it can call the base class constructor by using. Constructors and Inheritance Problem. c++ calling the constructor of base class. Just remove the constructor with the empty body most likely. Step 1: Start the program. The constructor calling order will be top to bottom.
calling a base constructor C++. Then the member fields are constructed. But you can use the parent's constructor in the child's constructor Sword () : Item (3) // Call the superclass constructor and set damage to 3 { // do something } Share Improve this answer Inheriting Constructors. Answer (1 of 5): If constructors were inherited in C++, it would cause many undesirable problems, and the main benefit of inheritance of members would not apply to constructors. Step 3: set s=0 and i=0. The main job of the constructor is to allocate memory for class objects. The constructor of the derived class calls the constructors of the base classes in the same order in which they are specified in the header of the derived class. Derived class can not inherit the base class constructor but it can call the base class constructor by using.
1. special_item s = special_item (); A function's signature comprises of it's name, it's parameters, and it's return type. }; This is because constructors are not members of the class, they are just use initialize the object values. Correct Code is class A Inheritance and Constructors in Java. Constructors are not called automatically. { Method overriding in C# inheritance Method overriding is a feature that allows to invoke child class method having same name and signature as in base class method in inheritance hierarchy if we use base class reference. In below c# inheritance source code , child class B overrides func () method of base class using override keyword and to
A subclass must declare its own constructors.
It is executed when an instance of the class is created. The name and return type are automatically the same, both being constructors, and you made both the paramters to them the same, void. The name and return type are automatically the same, both being constructors, and you made both the paramters to them the same, void. In a hierarchy, it is possible for both base classes and derived classes to have their own constructors. Correct Code is class A You can specify which of several base class constructors should be called during the creation of a derived class object.
Constructor call in multiple inheritance constructors class C: public A, public B; Constructors are called upon the order in which they are inherited First class A constructors are executed followed by class B constructors, then class C constructors Quiz Time Question 1. The reason for this is that when you write a constructor for an object of a derived class, you are responsible for ensuring that the members of the derived class object are properly initialized. Step 5: Perform s=s+a and i=i+1. calling a base constructor C++. The compilers default action is to call the default constructor in the base class. Method overriding in C# inheritance Method overriding is a feature that allows to invoke child class method having same name and signature as in base class method in inheritance hierarchy if we use base class reference. In below c# inheritance source code , child class B overrides func () method of base class using override keyword and to 1. special_item s = special_item (); A function's signature comprises of it's name, it's parameters, and it's return type.